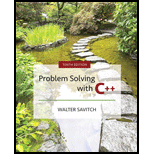
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13, Problem 9PP
Program Plan Intro
Creation of program to construct classes to implement maze
Program Plan:
- Define a class “Room” to define properties and methods.
- Define constructors to create new instance.
- Define a method “get_name()” to get name.
- Define a method “get_adjacent_room()” to get adjacent room.
- Define a method “link_room()” to assign value based on direction.
- Define a method “get_available_directions()” to get available directions.
- Define a main method.
- Create new room instance using class.
- Define connections in maze using “link_room()”.
- Call method “get_available_directions()” to get available directions.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
The purpose of this project is to assess your ability to (JAVA):
Implement a graph abstract data type.
The getDistance method should print the distance of all connected nodes/ vertices.
A graph is a set of vertices and a set of edges. Represent the vertices in your graph with an array of strings:
Represent the edges in your graph as a two-dimensional array of integers. Use the distances shown in the graph pictured here.Add the following functions to your graph class:
A getDistance function that takes two vertices and returns the length of the edge between them. If the vertices are not connected, the function should return the max value for an integer.
A getNeighbors function that takes a single vertex and returns a list of all the vertices connected to that vertex.
A print method that outputs an adjacency matrix for your graph.
Write a test program for your Graph class.
In C++, write a program that outputs the nodes of a graph in a breadth first traversal.
Data File: Please use this data file.
Text to copy:
100 1 3 -9991 4 -9992 5 -9993 2 -9994 -9995 7 8 -9996 4 7 -9997 -9998 -9999 7 8 -999
Diagram: Also, please take a look at the attached figure on and calculate the weights for the following edges:
0 -> 1 -> 4
0 -> 3 -> 2 -> 5 -> 7
0 -> 3 -> 2 -> 5 -> 8
6 -> 4
6 -> 7
9 -> 7
9 -> 8
To calculates these weights, please assume the following data:
0 -> 1 = 1
0 -> 3 = 2
1 -> 4 = 3
3 -> 2 = 4
2 -> 5 = 5
5 -> 7 = 6
5 -> 8 = 7
6 -> 4 = 8
6 -> 7 = 9
9 -> 7 = 10
9 -> 8 = 11
Create the following graph. Implement the following functions.
1. addEdge() that takes two vertices as two parameters and creates an Edge between them.
2. nonAdjacentVertices() that takes a single vertex as parameter, and returns an array of vertices that are NOT adjacent to that vertex.
3. Write a function addUnknownVertices() that takes a vertex as a parameters, and creates an Edge with the vertices that are NOT adjacent to it.
4. Write a method searchVertex() which takes an array of Vertices as parameters. The first member of the array will be the starting vertex and the last member will be the Vertex you want to reach. For example searchVertex(Vertex 0, Vertex 4, Vertex 3) will output “Vertex cannot be reached” since you can reach from 0 to 4, but cannot from 4 to 3. Again, searchVertex(Vertex 0, Vertex 1, Vertex 2, Node 3) will output “Vertex can be reached” since you can reach from 0 to 1, 1 to 2, 2 to 3.
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Similar questions
- Application "networkx" library is used for implementing graphs. Draw a graph using this library in python. (Hint: use 'add_node()", 'add_edge()' functions to draw edges and nodes). Construct the graph shown in figure using python language. Also, plot the graph using matplotlib library, Traverse the graph using DFS, and output the DFS order. 2arrow_forwardA programmer is designing a program to catalog all books in a library. He plans to have a Book class that stores features of each book: author, title, isOnShelf, and so on, with operations like getAuthor, getTitle, getShelf Info, and set Shelf Info. Another class, LibraryList, will store an array of Book objects. The LibraryList class will include operations such as listAll Books, addBook, removeBook, and searchForBook. The programmer plans to implement and test the Book class first, before implementing the LibraryList class. The program- mer's plan to write the Book class first is an example of (A) top-down development. (B) bottom-up development. (C) procedural abstraction. (D) information hiding. (E) a driver program.arrow_forwardThis is for python For this assignment, you will write a program to simulate a payroll application. To that effect, you will also create an Employee class, according to the specifications below. Since an Employee list might be large, and individual Employee objects may contain significant information themselves, we store the Employee list as a linked list. Employee class: Attributes • Employee ID: you can use a string – the ID will contain digits and/or hyphens. The ID must be provided during object construction and there should be no mechanism to change it later. • Number of hours worked in a week: a floating-point number. • Hourly pay rate: a floating-point number that represents how much the employee is paid for one hour of work. • Gross wages: a floating-point number that stores the number of hours times the hourly rate. Methods • A constructor (__init__) • Setter methods as needed. • Getter methods as needed. • This class should overload the __str__ or __repr__ methods so that…arrow_forward
- A graph (also known as a network) consists of nodes and edges. Edges connect nodes. Graphs/networks have many real-life applications. One of the most common examples is a social network (e.g., Facebook), where the people are represented by nodes and friendship/connection between two people is represented by an edge. See a picture of a graph below where the nodes are colored in red, green, and blue. -A blue node An edge connecting a blue node and a green node. [Image source: https://en.wikipedia.org/wiki/Graph_coloring] A simple graph can be expressed by the following two predicates. Given the predicates below: Edge(x, y): x is connected to y Color(x, z): x has color z and the following domains: domain of x and y: {all nodes in a graph}, domain of z: {RED, GREEN, BLUE, YELLOW}, translate the following statements into English. a) Vx3y Edge(x, y) b) Vz 3x Color(x, z) c) VxVyVz (Edge(x, y) A Color(x, z) → ¬Color(y, z))arrow_forwardAn airport has a runway for airplanes landing and taking off. When the runway is busy, airplanes wishing to take off or land have to wait. Landing airplanes get priority, and if the runway is available, it can be used. Implement a Java class, Airport.java, for this simulation, using two appropriate lists, one for the airplanes waiting to take off and one for those waiting to land. Note that the data structures you select for the two lists must be suitable for this purpose. For instance, the sooner an airplane comes for landing, the sooner it will land. Also, you must keep the record of all the airplanes that have already landed or taken off in one single list, to print out the activity log whenever asked, such that the sooner an airplane landed, the later it shows in the printout. To get a clear idea, please have a close look at the expected outputs of the execution of the tester class provided. The user enters the following commands: (The user entry has already been done in the tester…arrow_forwardStudent: Michael Reyes 2. Write a Java program that would resemble a payroll system. An input window that will accept the employee number and the number of days of rendered work. A 2d-ArrayList that contains the employee number and the rate per day is to be searched and used to compute the gross salary. Gross salary is computed by multiplying the number of days of rendered work by the rate per day of the particular employee. If the gross salary computed is greater than 100,000 the tax is 20% of the gross salary, otherwise, the tax is 10%. Deduct the tax from the gross salary to get the net pay. Display the employee number, gross salary, tax deduction, and net pay in an output window. Design your own input/output windows. (USE OOP CONCEPT JFRAME)arrow_forward
- 1. Write a program that would resemble a power utility billing system. There will be an input window that would enter or input the meter number (5 numeric digits) and the present meter reading in kilowatt hours, the maximum would be 9999 kilowatts. A search from a 2d-arraylist would be made in order to get the previous meter reading of a particular meter number. The 2d- arraylist consists of meter number (5 numeric digits) and the previous meter reading in kilowatt hours (4 numeric digits with 9999 as maximum value). Provide at least 5 sample data or 5 rows with meter number and previous meter reading each row for the 2d-arraylist. When an input of meter number and present meter reading is made, search the 2d-arraylist for the equivalent meter number. If a match is found, get the kilowatt hour used (KWH) by subtracting the previous meter reading from the present meter reading. Note that if the present meter reading is less than previous meter reading, add 10000 first to the present…arrow_forwardIN JAVA Alice and Bob are playing a board game with a deck of nine cards. For each digit between 1 to 9, there is one card with that digit on it. Alice and Bob each draw two cards after shuffling the cards, and see the digits on their own cards without revealing the digits to each other. Then Alice gives her two cards to Bob. Bob sees the digits on Alice’s cards and lays all the four cards on the table in increasing order by the digits. Cards are laid facing down. Bob tells Alice the positions of her two cards. The goal of Alice is to guess the digits on Bob’s two cards. Can Alice uniquely determine these two digits and guess them correctly? Input The input has two integers p,q (1≤p<q≤9) on the first line, giving the digits on Alice’s cards. The next line has a string containing two ‘A’s and two ‘B’s, giving the positions of Alice’s and Bob’s cards on the table. It is guaranteed that Bob correctly sorts the cards and gives the correct positions of Alice’s cards. Output If Alice can…arrow_forwardGiven a singly linked list, reverse the list. This means you have to reverse every node. For example if there are 4 nodes, the node at position 0 will move to position 3, the node at 1 will move to position 2 and so on. You do not have to write the Node class just write what you have been asked to. You are NOT allowed to create a new list. In python languagearrow_forward
- Write a java class Library for implementing a library application which accepts books into its list and user should be able to search books based on a book title or the Author. The books should be added to an ArrayList. Write the Main class with main function in it to display a menu to opt whether to add books, list books and search books. Use a constructor of Library class to add books. Use a method named searchTitle and searchAuthor in Library class to search the books. 1) Define class Library with required instance variable ArrayList for adding books 2) The Library class should have one constructor and three methods to do the below functions The Constructor needs to add books Method displayBook to List all the books in the library. Method named search Title to search books based on book title, this method should be able to accept a user string and search if a book with the title is in the added book list Method named searchAuthor to search books based on book title, this method…arrow_forwardProblem 3: In classroom, we implemented MyStack by including an ArrayList as private data field of the class (using composition). In this problem, we will use another way to implement the stack class. Define a new MyStack class that extends ArrayList. Draw the UML diagram for the classes and then implement MyStack. Write a test program that prompts the user to enter five strings and displays them in reverse order. (1) Your UML diagram: (3)arrow_forwardJava In this assignment, you will practice using an ArrayList. Your class will support a sample program that demonstrates some of the kinds of operations a windowing system must perform. Instead of windows, you will be manipulating “tiles.” A tile consists of a position (specified by the upper-left corner x and y), a width, a height, and color. Positions and distances are specified in terms of pixels, with the upper-left corner being (0, 0) and the x and y coordinate increasing as you move left and down, respectively. You won’t have to understand a lot about tiles and coordinates. That code has been written for you. You are writing a small part of the overall system that keeps track of the list of tiles. You are required to implement this using an ArrayList. The only method you need to use from the Tile class is the following: // returns true if the given (x, y) point falls inside this tile public boolean inside(int x, int y) Your class is to be called TileList and must…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
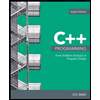
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning