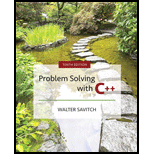
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13, Problem 8PP
Program Plan Intro
Creation of program to simulate customer arrivals at motor vehicles department
Program Plan:
- Define a class “Queue” to denote methods and operations required for queue.
- Declare variables that are required for program.
- Define a constructor “Queue()” and assign values.
- Define a method “Enqueue()” to insert values into a queue.
- Define a method “Dequeue()” to remove value from a queue.
- Define a method “Front()” to return front element of queue.
- Define a method “Size()” to return size of queue.
- Define a method “isEmpty()” to check whether queue is empty or not.
- Define a main method
- Call method “Enqueue()” to insert values into a queue.
- Call method “Dequeue()” to remove value from a queue.
- Call method “Front()” to return front element of queue.
- Call method “Size()” to return size of queue.
- Call method “isEmpty()” to check whether queue is empty or not.
- Loop until the user needs to compute result.
- To simulate customer arrival call “Enqueue()” method with required parameters.
- To help next customer call “Dequeue()” method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
QUESTION 9
Write a complete Java program to implement a QUEUE in a bank using LinkedList class as follows:
• Provide the menu as shown below; use an infinite loop; stop when user opts 4.
1. Add a customer
2. Remove a customer
3. Show the queue
4. Exit
• Add a customer should add an int number at the end of queue using the method addLast().
• Remove a customer should delete the int from front using the method removeFirst().
Show the queue should display the numbers in queue using the method System.out.printIn().
For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac).
An airport has a runway for airplanes landing and taking off. When the runway is busy, airplanes wishing to take off or land have to wait. Landing airplanes get priority, and if the runway is available, it can be used. Implement a Java class, Airport.java, for this simulation, using two appropriate lists, one for the airplanes waiting to take off and one for those waiting to land. Note that the data structures you select for the two lists must be suitable for this purpose. For instance, the sooner an airplane comes for landing, the sooner it will land. Also, you must keep the record of all the airplanes that have already landed or taken off in one single list, to print out the activity log whenever asked, such that the sooner an airplane landed, the later it shows in the printout. To get a clear idea, please have a close look at the expected outputs of the execution of the tester class provided. The user enters the following commands: (The user entry has already been done in the tester…
Write a main() method for the QuickSort class. Create a queue of Item objects and sort them in increasing order. Populate the queue with several Account/Item objects and then call the quicksort method. The Account class has the following instance variables:
int accountNumber
String customerName
double accountBalance
The Item class has the following instance variables:
int itemId;
String itemName
double itemPrice
Note: This is JAVA PROGRAMMING
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Similar questions
- The implementation of a queue in an array, as given in this chapter, uses the variable count to determine whether the queue is empty or full. You can also use the variable count to return the number of elements in the queue. On the other hand, class linkedQueueType does not use such a variable to keep track of the number of elements in the queue. Redefine the class linkedQueueType by adding the variable count to keep track of the number of elements in the queue. Modify the definitions of the functions addQueue and deleteQueue as necessary. Add the function queueCount to return the number of elements in the queue. Also, write a program to test various operations of the class you defined.arrow_forwardIn Java In the real world, you will often be tasked with understanding and improving another person’sinelegant, hard-to-understand code. This project provides practice for that type of work.The given program, shown below, implements a circular-array queue. A queue is a British term for aline of people waiting to be served. A queue can also refer to any line of items where the item at thefront of the queue is served next, and new items are added at the rear of the queue. Informationtransmission systems, like the Internet, have lots of queues, where messages in transit are temporarilystalled at some intermediate system node, waiting to get into the next available time slot on the next legof their journey. A queue’s length is the total number of people or items currently waiting. When thenext item is served, that shortens the queue by one. When another person arrives, that lengthens thequeue by one. A queue’s capacity is the maximum number of items that can fit in the line at one time.If…arrow_forwardGiven a partial main() and PlaneQueue class, write the push() and pop() methods for PlaneQueue. Then complete the main() to read in whether flights are arriving or have landed at an airport. An "arriving" flight is pushed onto the queue. A "landed" flight is popped from the front of the queue. Output the queue after each plane is pushed or popped. Entering -1 exits the program. Ex: If the input is: arriving AA213 arriving DAL23 arriving UA628 landed -1 the output is: Air-traffic control queue Next to land: AA213 Air-traffic control queue Next to land: AA213 Arriving flights: DAL23 Air-traffic control queue Next to land: AA213 Arriving flights: DAL23 UA628 AA213 has landed. Air-traffic control queue Next to land: DAL23 Arriving flights: UA628 JAVA Pleasearrow_forward
- For the Assignment 5 (Part 2), Routes v.2 at the end of this module you will create a Route class that has a member a "bag" of Leg objects. The bag is to use a vector of pointers, but since all the objects in the bag are to be Leg s, it's okay to use Leg* instead of void* in the bag's declaration. You wrote its first constructor in a previous exercise. Now write a second one. (You may refer to the Assignment page to refer to the completed class declarations of Leg and Route classes). Assume that class Leg has data member C strings const char* const beginCity; and const char* const endCity; that are available to Route by a friend relationship. Write a constructor function for a Route class that takes exactly two parameters: a constant Route object reference to an already-existing Route , and a constant Leg object reference to be appended to the end of that Route to form the new Route . Here's the algorithm: Copy the first parameter's bag into the host object's bag. If the endCity of…arrow_forwardA readinglist is a doubly linked list in which each element of the list is a book. So, you must make sure that Books are linked with the previous prev and next element. A readinglist is unsorted by default or sorted (according to title) in different context. Please pay attention to the task description below. Refer to the relevance classes for more detail information. Implement the add_book_sorted method of the ReadingList class. Assume the readinglist is sorted by title, the add_book_sorted method takes an argument new_book (a book object), it adds the new_book to the readinglist such that the readinglist remain sorted by title. For example, if the readinglist contain the following 3 books: Title: Artificial Intelligence Applications Author: Cassie Ng Published Year: 2000 Title: Python 3 Author: Jack Chan Published Year: 2016 Title: Zoo Author: Cassie Chun Published Year: 2000 If we add another book (titled "Chinese History"; author "Qin Yuan"; and published year 1989) to the…arrow_forwardPlease help me with this! The countOff() method displays the name of each officer removed from the queue, in the order in which they are removed, and displays the name of the officer that goes for help. For example, the output for the queue of defenders above with a count of 4 would appear as:Defenders leaving the queue are: Horace Alsbury, PVT, Kentucky, survivorSimon Arreola, —, —, survivorPeter James Bailey III, PVT, Kentucky, fatalityJohn J. Ballentine, PVT, Pennsylvania, fatalityRobert Allen, PVT, Virginia, fatalityMicajah Autry, PVT, North Carolina, fatalityWilliam Charles M. Baker, CPT, Missouri, fatalityJames L. Allen, PVT, Kentucky, survivorJesse B. Badgett, —, Texas, survivorRichard W. Ballentine, PVT, Scotland, fatalityJosé María Arocha, —, —, survivorJuan Abamillo, SGT, Texas, fatalityIsaac G. Baker, PVT, Arkansas, fatalityJuan A. Badillo, SGT, Texas, fatalityJohn Ballard, —, —, fatalityMiles DeForest Andross, PVT, Vermont, fatality The defender going for help is: George…arrow_forward
- Write a Java public static general method that doesn't belong to the Queue class(assuming the Queue type is Book and Book has a clone method, Book also has a method called getGenre() which returns the genre of the book): Queue findOneGenreBooks(Queue Q, String genreType) that searches in the first parameter Q for all books that has the same genre type as defined by the second parameter genreType, return all of the books that have the specified genre type as a Queue. (For example, you may want to find all cartoon books and return them as a Queue). Please note: You are only allowed to use the Queue class which has constructors, enqueue, dequeue, front, isEmpty, size, toString, and clone methods.arrow_forwardUsing c++ I would like to implement a queue as a class with a linked list. This queue Is used to help the class print job in displaying things like: a confirmation of the job request received and the status ( denied/accepted) along with the details such as a tracking number, position in the queue( if accepted), reason for denial (if denied), etc. These classes will be used in a menu-driven application that has the following options: request a print job ask for the job name; the name may have blank spaces and consist of alphanumeric characters only, must start with a letter display a confirmation of the job request received and the status ( denied/accepted) along with the details such as a tracking number, position in the queue( if accepted), reason for denial (if denied), etc execute a print job (remove from the queue ) display a confirmation along with the tracking number, the name of the print job, and the number of print jobs currently in the queue display number of print…arrow_forwardA singly linked circular list is a linked list where the last node in the list points to the first node in the list. A circular list does not contain NULL pointers. A good example of an application where a circular linked list should be used is an item in the shopping cart In an online shopping cart, the system must maintain a list of items and must calculate the total bill by adding the amount of all the items in the cart, Implement the above scenario using a Circular Link List of data structure in C++ Programming. Do Following: First create a class Item having id, name, price, and quantity provide appropriate methods and then Create Cart/List class which holds an items object to represent total items in the cart and next pointer Implement the method to add items in the array, remove an item and display all items. Now in the main do the following Insert Items in the list Display all items. Traverse the link list so that each item's bill gets calculated (by multiplying quantity with…arrow_forward
- Java Program, Dont Copy Consider a class Student that has an attribute age and a method getAge(): StudentList is a class representing a linkedlist of students. Write sumListAge_lterative......), a method that computes and returns the sum of the ages of all students in the list using the iterative way. Parameters of the method should be specified accordinglyarrow_forwardWrite a Java public static general method which doesn't belong to Queue class(assuming the Queue type is Book and Book has a clone method, Book also has a method called getGenre() which returns the genre of the book): Queue findOneGenreBooks(Queue Q, String genreType) that searches in the first parameter Q for all books that has the same genre type as defined by the second parameter genreType, return all of the books that have the specified genre type as a Queue. (For example, you may want to find all cartoon books and return them as a Queue). Please note: You are only allowed to use the Queue class which has constructors, enqueue, dequeue, front, isEmpty, size, toString, and clone methods.arrow_forwardGiven a ListItem class, complete main() using the built-in LinkedList type to create a linked list called shoppingList. The program should read items from input (ending with -1), adding each item to shoppingList, and output each item in shoppingList using the printNodeData() method. Ex. If the input is: milk bread eggs waffles cereal -1 the output is: milk bread eggs waffles cereal ListItem.java (read only) public class ListItem {private String item; public ListItem() {item = "";} public ListItem(String itemInit) {this.item = itemInit;} // Print this node public void printNodeData() {System.out.println(this.item);}} ShoppingList.java import java.util.Scanner;import java.util.LinkedList; public class ShoppingList {public static void main (String[] args) {Scanner scnr = new Scanner(System.in); // TODO: Declare a LinkedList called shoppingList of type ListItem String item;// TODO: Scan inputs (items) and add them to the shoppingList LinkedList// Read inputs until a -1 is input// TODO:…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
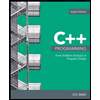
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning