import java.awt.*; public class TestRandomWalker { public static final int STEPS = 500; public static void main(String[] args) { RandomWalker walker = new RandomWalker(); // instantiate Walker object DrawingPanel panel = new DrawingPanel(500, 500); Graphics g = panel.getGraphics(); // advanced features -- center and zoom in the image panel.getGraphics().translate(250, 250); panel.getGraphics().scale(4, 4); // make the walker walk, and draw its movement int prevX = walker.getX(); // initialize Walker display int prevY = walker.getY(); for (int i = 1; i <= STEPS; i++) { g.setColor(Color.BLACK); g.drawLine(prevX, prevY, walker.getX(), walker.getY()); // update Walker display walker.move(); // move Walker 1 step prevX = walker.getX(); // update x value prevY = walker.getY(); // update y value g.setColor(Color.RED); g.drawLine(prevX, prevY, walker.getX(), walker.getY()); // update Walker display int steps = walker.getSteps(); // record Walker steps if (steps % 10 == 0) { System.out.println(steps + " steps"); } panel.sleep(100
import java.awt.*;
public class TestRandomWalker {
public static final int STEPS = 500;
public static void main(String[] args) {
RandomWalker walker = new RandomWalker(); // instantiate Walker object
DrawingPanel panel = new DrawingPanel(500, 500);
Graphics g = panel.getGraphics();
// advanced features -- center and zoom in the image
panel.getGraphics().translate(250, 250);
panel.getGraphics().scale(4, 4);
// make the walker walk, and draw its movement
int prevX = walker.getX(); // initialize Walker display
int prevY = walker.getY();
for (int i = 1; i <= STEPS; i++) {
g.setColor(Color.BLACK);
g.drawLine(prevX, prevY, walker.getX(), walker.getY()); // update Walker display
walker.move(); // move Walker 1 step
prevX = walker.getX(); // update x value
prevY = walker.getY(); // update y value
g.setColor(Color.RED);
g.drawLine(prevX, prevY, walker.getX(), walker.getY()); // update Walker display
int steps = walker.getSteps(); // record Walker steps
if (steps % 10 == 0) {
System.out.println(steps + " steps");
}
panel.sleep(100);
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

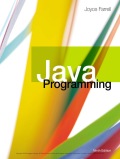
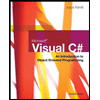
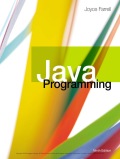
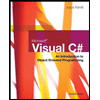