Checkpoint B If this population plays (and loses) the lottery 2 times: It could become [0,3,4,5,6], if the first individual played twice Or it could become [2,3,4,4,5], if the last two individuals each played once etc Continuing the example, scholarships might then award a total of $3 of awards to the population in the form of $1 scholarships. If the wealth had originally been [0,3,4,5,6], then: It could become[3,3,4,5,6], if the 1st individual got all three awards Or it could become [0,4,5,5,7], if it was distributed equally among the 2nd, 3rd, and 5th individuals etc We assume the lottery system is backed by a relatively huge pool of capital, so that scholarships are awarded no matter how many lottery winners there are. We also assume who plays the lottery and who benefits from scholarships will be random, at the individual-level. Later, at the population-level, we will select behaviors for our simulation based on social science research. The function generate_disparity_msg() returns a string summarizing the distribution of wealth. Here are examples of that analysis: highIncomeList lowIncomeList Wealth Distribution [2,3,4,5,6] [6,5,4,3,2] High income: 50% of wealth Low income: 50% of wealth [5, 6, 10, 14] [1, 5, 7, 2] High income: 70% of wealth Low income: 30% of wealth [4, 10, 2, 5, 8] [2, 7] High income: 76% of wealth Low income: 24% of wealth Implementation Strategy Implement each function from the template following the description in their docstring: sim_lottery() award_scholarship() generate_disparity_msg() For the messages returned by generate_disparity_msg(), adapt this fstring for your code: msg = f"Decade {decade}: High income group " +\ f"has {highIncomePercent:.0f}% of the community's wealth. "+\ f"Low income group has {lowIncomePercent:.0f}% "+\ f"of the community's wealth." Here is my code from checkpoint A : import random def generate_lottery_numbers(): """Generates a list of 5 random integers between 1 and 42, inclusive, with no duplicates.""" return random.sample(range(1, 43), 5) def count_matches(my_list, lottery_list): """Counts the number of matches between two lists representing the player’s chosen numbers and the generated lottery numbers.""" return len(set(my_list) & set(lottery_list)) def play_lottery_once(): """Plays the lottery one time and returns the total dollar amount gained by the player.""" player_numbers = generate_lottery_numbers() lottery_numbers = generate_lottery_numbers() matches = count_matches(player_numbers, lottery_numbers) # Determine the reward based on the number of matches if matches == 0: return -1 # Loss, -$1 elif matches == 1: return -1 # Break-even, $0 elif matches == 2: return 0 # No win for 2 matches elif matches == 3: return 10 # Win $4 (considering the $1 cost) elif matches == 4: return 197 # Win $19 (considering the $1 cost) elif matches == 5: return 212534 # Win $99 (considering the $1 cost) def sim_many_plays(n): """Simulates a single person playing the lottery n times, to determine their overall winnings at the end of each simulated lottery.""" winnings = [] for _ in range(n): result = play_lottery_once() if not winnings: winnings.append(result) else: winnings.append(winnings[-1] + result) return winnings if __name__ == "__main__": seed = int(input('Enter a seed for the simulation: ')) random.seed(seed) # Simulate 1000 plays by one person and print the winnings. winnings = sim_many_plays(1000) print("Total winnings after each lottery:", winnings) This is template for check point B:
Checkpoint B
If this population plays (and loses) the lottery 2 times:
- It could become [0,3,4,5,6], if the first individual played twice
- Or it could become [2,3,4,4,5], if the last two individuals each played once
- etc
Continuing the example, scholarships might then award a total of $3 of awards to the population in the form of $1 scholarships. If the wealth had originally been [0,3,4,5,6], then:
- It could become[3,3,4,5,6], if the 1st individual got all three awards
- Or it could become [0,4,5,5,7], if it was distributed equally among the 2nd, 3rd, and 5th individuals
- etc
We assume the lottery system is backed by a relatively huge pool of capital, so that scholarships are awarded no matter how many lottery winners there are. We also assume who plays the lottery and who benefits from scholarships will be random, at the individual-level. Later, at the population-level, we will select behaviors for our simulation based on social science research.
The function generate_disparity_msg() returns a string summarizing the distribution of wealth. Here are examples of that analysis:
highIncomeList | lowIncomeList | Wealth Distribution |
---|---|---|
[2,3,4,5,6] | [6,5,4,3,2] | High income: 50% of wealth Low income: 50% of wealth |
[5, 6, 10, 14] | [1, 5, 7, 2] | High income: 70% of wealth Low income: 30% of wealth |
[4, 10, 2, 5, 8] | [2, 7] | High income: 76% of wealth Low income: 24% of wealth |
Implementation Strategy
Implement each function from the template following the description in their docstring:
- sim_lottery()
- award_scholarship()
- generate_disparity_msg()
For the messages returned by generate_disparity_msg(), adapt this fstring for your code:
msg = f"Decade {decade}: High income group " +\ f"has {highIncomePercent:.0f}% of the community's wealth. "+\ f"Low income group has {lowIncomePercent:.0f}% "+\ f"of the community's wealth."
Here is my code from checkpoint A :
import random
def generate_lottery_numbers():
"""Generates a list of 5 random integers between 1 and 42, inclusive, with no duplicates."""
return random.sample(range(1, 43), 5)
def count_matches(my_list, lottery_list):
"""Counts the number of matches between two lists representing the player’s chosen numbers and the generated lottery numbers."""
return len(set(my_list) & set(lottery_list))
def play_lottery_once():
"""Plays the lottery one time and returns the total dollar amount gained by the player."""
player_numbers = generate_lottery_numbers()
lottery_numbers = generate_lottery_numbers()
matches = count_matches(player_numbers, lottery_numbers)
# Determine the reward based on the number of matches
if matches == 0:
return -1 # Loss, -$1
elif matches == 1:
return -1 # Break-even, $0
elif matches == 2:
return 0 # No win for 2 matches
elif matches == 3:
return 10 # Win $4 (considering the $1 cost)
elif matches == 4:
return 197 # Win $19 (considering the $1 cost)
elif matches == 5:
return 212534 # Win $99 (considering the $1 cost)
def sim_many_plays(n):
"""Simulates a single person playing the lottery n times, to determine their overall winnings at the end of each simulated lottery."""
winnings = []
for _ in range(n):
result = play_lottery_once()
if not winnings:
winnings.append(result)
else:
winnings.append(winnings[-1] + result)
return winnings
if __name__ == "__main__":
seed = int(input('Enter a seed for the simulation: '))
random.seed(seed)
# Simulate 1000 plays by one person and print the winnings.
winnings = sim_many_plays(1000)
print("Total winnings after each lottery:", winnings)
This is template for check point B:

Step by step
Solved in 3 steps

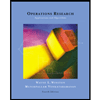
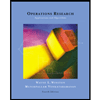