legacy_scrapeAll
.py
keyboard_arrow_up
School
Rutgers University *
*We aren’t endorsed by this school
Course
213
Subject
Computer Science
Date
May 2, 2024
Type
py
Pages
23
Uploaded by PrivateDragonMaster1090 on coursehero.com
import json import sys from bs4 import BeautifulSoup import requests as requests from tqdm import tqdm import datetime import operator #http://sis.rutgers.edu/oldsoc/courses.json?
subject=198&semester=12022&campus=NB&level=U #http://sis.rutgers.edu/oldsoc/courses.json?
subject=198&semester=12022&campus=NB,NK,CM&level=U # above works but only gets subject 198 which isn't very useful #http://sis.rutgers.edu/oldsoc/courses.json?
semester=12022&campus=CM&level=U,G #break down api... #base url base_url = "http://sis.rutgers.edu/oldsoc/courses.json" #ALL COURSES ARE 20MB in unzipped form #surprisingly only 871kb gzipped #NEW API ALERT BABYYYY #https://sis.rutgers.edu/soc/api/courses.json?
year=2022&term=9&campus=NB #this does work #split term off #get all courses for new brunswick in one file here lets gooo currentDayList = [] locationList = [] campusList = [] buildingCodeList = [] #get year argument from command line #expecting 4 digit year year = sys.argv[1] #expecting 1 digit term code #7 - summer #9 - fall #0 - winter
#1 - spring term = sys.argv[2] #expecting NB,NK,CM schoolcampus = sys.argv[3] #if unset, use default (figure out from current date) # currCalendarYear = datetime.datetime.now().year # currMonth = datetime.datetime.now().month # currDay = datetime.datetime.now().day #found this from #https://github.com/anxious-engineer/Rutgers-Course-API/blob/
56af65bd17c5ddf512be5bb2a3d28f05c8d50085/DB-PoC/rusoc/api.py month_to_semester_map = { '1' : '1', 1 : '1', '2' : '1', 2 : '1', '3' : '1', 3 : '1', '4' : '1', 4 : '1', '5' : '1', 5 : '1', '6' : '1', 6 : '1', '7' : '7', 7 : '7', '8' : '7', 8 : '7', '9' : '9', 9 : '9', '10' : '9', 10 : '9', '11' : '9', 11 : '9', '12' : '9', 12 : '9', } # if year == "": # #get current school year # year = str(currCalendarYear) # if term == "": # #determine which term it is
# #after jan 15th and before may 2 is spring session # if currMonth >= 1 and currMonth <= 4: # #spring # term = "1" # elif currMonth >= 5 and currMonth <= 8: # #summer # term = "5" # elif currMonth >= 9 and currMonth <= 12: # #fall # term = "9" # if schoolcampus == "": # schoolcampus = "NB" #https://sis.rutgers.edu/soc/?term=92022 #this can convert PH -> to PHARMACY & id:3750 #nameConversion nameConvertUrl = "https://sis.rutgers.edu/soc/?
term="+term+""+year print(nameConvertUrl) # r = requests.get("https://sis.rutgers.edu/soc/?term=92022") r = requests.get(nameConvertUrl) soup = BeautifulSoup(r.content, features="html.parser") jsonDiv = json.loads(soup.find('div', {"id": "initJsonData"}).text) buildingCodes = [d["code"] for d in jsonDiv["buildings"]] buildingNames = [d["name"] for d in jsonDiv["buildings"]] buildingIDs = [d["id"] for d in jsonDiv["buildings"]] def getBuildingID(buildingCodeAbbrev): tempIndex = buildingCodes.index(buildingCodeAbbrev) return { "name": buildingNames[tempIndex], "id": buildingIDs[tempIndex] } #print(dict.keys(jsonDiv)) #print(json.dumps(jsonDiv, indent=2)) #print(json.dumps(jsonDiv['buildings'], indent=2))
#get coords of buildings #for now, import file. later, pull directly from URL buildingLayer = open("fromMap/buildings-parking-layer.json") buildingLayerJson = json.load(buildingLayer) #print(json.dumps(buildingLayerJson, indent=2)) #print(dict.keys(buildingLayerJson)) buildingLayerIndex = [d["id"] for d in buildingLayerJson['features']] def getBuildingGeoData(buildingID): if buildingID in buildingLayerIndex: curr = buildingLayerJson['features']
[buildingLayerIndex.index(buildingID)] return { "geometry": curr['geometry'], "properties": curr['properties'] } else: return #get coords of regions #NOT HELPFUL! # campusLayer = open("fromMap/districts.json") # campusLayerJSON = json.load(campusLayer) # #print(json.dumps(buildingLayerJson, indent=2)) # #print(dict.keys(buildingLayerJson)) # campusLayerIndex = [d["id"] for d in campusLayerJSON['features']] # print(campusLayerIndex) # def getDistrictGeoData(campusID): # campusID = int(campusID) # if campusID in campusLayerIndex: # #print("Campus id:"+str(campusID)) # curr = campusLayerJSON['features']
[campusLayerIndex.index(campusID)] # return { # "geometry": curr['geometry'], # "properties": curr['properties'] # } # else: # return #MAP API ALERT!!! #https://maps.rutgers.edu/#/?click=true&selected=3117 map_base_url = ""
#IMAGE API image_base_url = "https://storage.googleapis.com/rutgers-campus-
map-building-images-prod/" image_end_url = "/00.jpg" #IMPORT NOW DOWNLOAD LATER # coursedataFile = open("fromSIS/courses2.json") # coursedataJSON = json.load(coursedataFile) #download #https://sis.rutgers.edu/soc/api/courses.json?
year=2022&term=9&campus=NB,NK,CM #could look into #https://scheduling.rutgers.edu/scheduling/class-scheduling/
standard-course-periods-0 #this page to help compress the data #since most classes follow the standard course periods listed here, #the abbreviations can be used to express the standard class times #and the full times can be used for the exceptions #Standard Course Periods # 80min Periods
55min Periods
180min Periods # 1
8:30 9:40am
1,2
8:30 11:40am
2*
10:35 1:20pm # 3
12:10 1:20pm
3,4
12:10 3:20pm
4*
2:15 5:00pm # 5
3:50 5:00pm
5,6
3:50 7:00pm
6*
5:55 8:40pm # 7
7:30 8:40pm
Grad Eve
6:00 10:40pm
8*
9:35 10:30pm #Standard period combinations: 2x80min, 2x55min # Monday
Tuesday
Wednesday Thursday
Friday # 1
MTh1 TF1
W1F6
MTh1
TF1 # 2
MTh2 TF2
W2F5
MTh2
TF2 # 3
MTh3 TF3
W3F4
MTh3
TF3 # 4
MW4
TTh4
MW4
TTh4
W3F4
# 5
MW5
TTh5
MW5
TTh5
W2F5 # 6
MW6
TTh6
MW6
TTh6
W1F6 # 7
MW7
TTh7
MW7
TTh7
# 8
MW8
TTh8
MW8
TTh8
# Standard Period Combinations: 3x80min, 3x55min # All meetings should take place in the same room # Monday
Tuesday
Wednesday Thursday
Friday # 1
MWTh1
TWF1 MWTh1 MWTh1
TWF1 # TWF1 # 2
MWTh2
TWF2 MWTh2 MWTh2
TWF2 # TWF2 # 3
MWTh3
TWF3 MWTh3 MWTh3
TWF3 # TWF3 # 4
MWF4 TThF4
MWF4 TThF4 MWF4 # TThF4 # 5
MWF5 TThF5
MWF5 TThF5 MWF5 # TThF5 # 6
MWF6 TThF6
MWF6 TThF6 MWF6 # TThF6 #https://scheduling.rutgers.edu/scheduling/academic-calendar scheduleURL = "https://scheduling.rutgers.edu/scheduling/academic-calendar" sched = requests.get(scheduleURL) schedSoup = BeautifulSoup(sched.content, features="html.parser") #find div.responsive-table__scroll table.pretty-table.responsive-
enabled # schedTable = schedSoup.find_all('div', {"class": "responsive-
table__scroll"}) # print(schedSoup.body.find({"class": "responsive-table"})) #found it! # print(schedSoup.table) #.find('table', {"class": "pretty-table responsive-enabled"}) #this is for new api, which does not support multiple campuses, so I am using old api
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
/* Filename: logserver.c *//*******************************************************************************************modify this program to log the message from clients to a log file.
******************************************************************************************/#include<stdio.h>#include<sys/ipc.h>#include<sys/shm.h>#include<sys/types.h>#include<string.h>#include<errno.h>#include<stdlib.h>
#define BUF_SIZE 1024#define SHM_KEY 0x1234
struct shmseg {int cnt;int new;int complete;char buf[BUF_SIZE];};
int main(int argc, char *argv[]) {int shmid;struct shmseg *shmp;shmid = shmget(SHM_KEY, sizeof(struct shmseg), 0666|IPC_CREAT);if (shmid == -1) {perror("Shared memory");return 1;}// Attach to the segment to get a pointer to it.shmp = shmat(shmid, NULL, 0);if (shmp == (void *) -1) {perror("Shared memory attach");return 1;}/* Transfer blocks of data from shared memory to log file if the message from client set shmp->complete ==1, the we…
arrow_forward
C PROGRAMMING.
using the following program as a start point:
/* * NetworkServer.c * ProgrammingPortfolio Skeleton * */
/* You will need to include these header files to be able to implement the TCP/IP functions */#include <stdio.h>#include <stdlib.h>#include <string.h>#include <sys/types.h>#include <netinet/in.h>#include <netdb.h>#include <fcntl.h>#include <unistd.h>#include <errno.h>#include <sys/socket.h>
/* You will also need to add #include for your graph library header files */
int main(int argc, const char * argv[]){ int serverSocket = -1; printf("Programming Portfolio 2022 Implementation\n"); printf("=========================================\n\n"); /* Insert your code here to create, and the TCP/IP socket for the Network Server * * Then once a connection has been accepted implement protocol */
TASK:
you are to write a TCP/IP server that can build up a graph of a network ofnetworks…
arrow_forward
IN SCALA PLEASE
COULD YOU COMPLETE THE CODE OF FUNCTIONS: get_csv_url, process_ratings, process_movies, groupById, favourites, suggestions and recommendations
import io.Source
import scala.util._
// (1) Implement the function get_csv_url which takes an url-string
// as argument and requests the corresponding file. The two urls
// of interest are ratings_url and movies_url, which correspond
// to CSV-files.
//
// The function should ReTurn the CSV-file appropriately broken
// up into lines, and the first line should be dropped (that is without
// the header of the CSV-file). The result is a list of strings (lines
// in the file).
def get_csv_url(url: String) : List[String] = ???
val ratings_url = """https://nms.kcl.ac.uk/christian.urban/ratings.csv"""
val movies_url = """https://nms.kcl.ac.uk/christian.urban/movies.csv"""
// testcases
//-----------
//:
//val movies = get_csv_url(movies_url)
//ratings.length // 87313
//movies.length // 9742
// (2) Implement two functions that…
arrow_forward
What does the following code segment and subroutine do?
.equ SIZE =128.equ TABLE_L =$60.equ TABLE_H =$00
rjmp RESET
.def A =r13.def B =r14.def cnt2 =r15.def cnt1 =r16.def endL =r17.def endH =r18
mystery:
mov ZL,endLmov ZH,endHmov cnt2,cnt1i_loop: ld A,Z ld B,-Z cp A,B brlo L1 st Z,A std z+1,BL1: dec cnt2 brne i_loop dec cnt1 brne mystery ret
Converts temperature values from one scale to another
Adds a constant to a table of bytes
Sorts numbers in ascending order
Converts ASCII characters from lower case to upper case
arrow_forward
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import org.json.JSONObject;
public class Myclass {
public static void main(String[] args) throws Exception {
try {
URL url = new URL("https://parseapi.back4app.com/classes/City?count=1&limit=10&keys=name,population");
HttpURLConnection urlConnection = (HttpURLConnection)url.openConnection();
urlConnection.setRequestProperty("X-Parse-Application-Id", "mxsebv4KoWIGkRntXwyzg6c6DhKWQuit8Ry9sHja"); // This is the fake app's application id
urlConnection.setRequestProperty("X-Parse-Master-Key", "TpO0j3lG2PmEVMXlKYQACoOXKQrL3lwM0HwR9dbH"); // This is the fake app's readonly master key
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(urlConnection.getInputStream()));
StringBuilder stringBuilder = new…
arrow_forward
#The Iris Dataset
import sklearn.datasetsimport matplotlib.pyplot as plt import numpy as np import scipy
iris = sklearn.datasets.load_iris()
Write a function that takes in an index i and prints out a verbose desciption of the species and measurements for data point i. For example:Data point 5 is of the species setosaIts sepal length (cm) is 5.4Its sepal width (cm) is 3.9Its petal length (cm) is 1.7Its petal width (cm) is 0.4
arrow_forward
STEP 1: Begin work within your Jupyter Notebook by importing the following modules:
import numpy as np
import pandas as pd
from matplotlib import pyplot as plt
import re
Jupyter Notebooks
Q1. Within your Jupyter Notebook, write the code for a Python function called
def parseWeatherByYear(year) :
This function will parse an html page containing weather for an entire year of data for the city of Toronto.
The html pages containing weather data can be downloaded from: https://www.extremeweatherwatch.com/cities/toronto/year-2023
The file to parse for this lab however can be downloaded here: https://matrix.senecacollege.ca/~danny.abesdris/prg550.232/labs/lab6/torontoWeather.2023.html
The html file itself contains markers as where to begin parsing the data to extract. The 3 pieces of data that must be extracted consist of the high and low temperatures (in degrees Celsius) as well as the amount of precipitation (in cm) for every day so far in the current year (2023).
A series…
arrow_forward
The questions should be solved using Linux Bash Scripting. In the file there should be solution code and one/two screenshot for running of program. Student id and names should be written inside the file. The assignment should be sent as PDF. Upload Link: https://forms.office.com/r/JfpSa2dDi8 Question 1. Read "n" and generate following pattern according to givennas below. Ex: for given value forn:4\[ \begin{array}{l} 1 \\ 23 \\ 456 \\ 78910 \end{array} \]
arrow_forward
Rewrite the program in Example 3-1. DigestThread class to output the result of each file on an output file for each one by assigning a new name for the output file.
The language is Java
Example 3-1. DigestThread
import java.io.*;
import java.security.*;
import javax.xml.bind.*; // for DatatypeConverter; requires Java 6 or JAXB 1.0
public class DigestThread extends Thread {
private String filename;
public DigestThread(String filename) {
this.filename = filename;
}
@Override
public void run() {
56 | Chapter 3: Threads
www.it-ebooks.info
try {
FileInputStream in = new FileInputStream(filename);
MessageDigest sha = MessageDigest.getInstance("SHA-256");
DigestInputStream din = new DigestInputStream(in, sha);
while (din.read() != -1) ;
din.close();
byte[] digest = sha.digest();
StringBuilder result = new StringBuilder(filename);
result.append(": ");
result.append(DatatypeConverter.printHexBinary(digest));
System.out.println(result);
} catch (IOException ex) {…
arrow_forward
; initialized data heresection .data
msg db "Print String!", 0xamsglen equ $-msg ; string length in bytesSTDOUT equ 1 ; standard output deviceSYS_write equ 1 ; write messageSYS_exit equ 60 ; exit programEXIT_OK equ 0 ; return value
; code goes heresection .text
; The '_start' label identifies the program's entry point for the; linker for the 'nasm' assembler. If you use 'gcc', the entry point; is labeled 'main'global _start_start:
; send message string to standard output mov rsi, msg ; text address mov rdx, msgln ; string length (error here) mov rdi, STDOUT ; output device mov rax, SYS_write ; rax holds the function syscall ; system…
arrow_forward
In python, I'd like to split the contents in an XML file into separate parallel arrays. An example would be:
<PROFILE>
<STUDENT>
<NAME>Jim</NAME>
<AGE>18</AGE>
<GRADE>95%</GRADE>
</STUDENT>
<STUDENT>
<NAME>Andrew</NAME>
<AGE>16</AGE>
<GRADE>82%</GRADE>
</STUDENT>
</PROFILE>
into:
name = ['Jim', 'Andrew']
age = ['18', '16']
grade = ['95%', '82%']
arrow_forward
Python
Data =
{
"logs": [
{
"logType": “xxxxx”
},
{
"logType": “43435”
},
{
"logType": “count”
},
{
"logType": “4452”
},
{
"logType": “count”
},
{
"logType": “count”
},
{
"logType": “43435”
},
{
"logType": "count"
},
{
"logType": "count"
}
]
}
So, please count the total of "logType": “count”
My desired output:
{
"count”: 5
}
arrow_forward
In C#: Create a function that takes in one argument: The url to an API endpoint. The function should make the API call and pull the data in, convert it to a dictionary, and pull out the number of kills from the last 7 games the user played. The function should return the sum of the top five number of kills from these games.
Call Of Duty API: https://rapidapi.com/elreco/api/call-of-duty-modern-warfare
arrow_forward
Implement a blog server in C
1. Implement get of all posts (GET /posts), and individual post (GET /post/1)
2. Implement creation of post (POST /posts). Allow the user to enter their name, but check that they are a valid user in db. Do error checking! The user should not specify the post id; that should come from the database.
3. Write three html files to test this; serve them with the blog server: index.html shows the list of posts with a link to each. post.html shows a post. publish.html allows the creation of a new post. Link these together as a user would expect!
arrow_forward
package encrypt1;
import java.io.*;import java.util.*;
public class Encrypt1 {
public static void main(String[] args) { if(args.length!2) ERROR MESSAGE { System.out.println("Invalid number of argumentss. Usage:src target"); } try { BufferedInputStream in = new BufferedInputStream (new FileInputStream(args[0])); // Create BufferedOutputStream from bos BufferedOutputStream out = new BufferedOutputStream (new FileOutputStream(args[1])); // Declare the variable num int buffer; // Checks content in the variable num is not equal to -1 while ((buffer=in.read())!=-1) { // Call the function Write() out.write(buffer+5); } System.out.println("Encrypted file saved"); } catch(IOException ex) { ex.printStackTrace(); }}}
arrow_forward
Language: Python
Goal: implement decryption in the attached code block
import argparse
import os
import time
import sys
import string
# Handle command-line arguments
parser = argparse.ArgumentParser()
parser.add_argument("-d", "--decrypt", help='Decrypt a file', required=False)
parser.add_argument("-e", "--encrypt", help='Encrypt a file', required=False)
parser.add_argument("-o", "--outfile", help='Output file', required=False)
args = parser.parse_args()
encrypting = True
try:
ciphertext = open(args.decrypt, "rb").read()
try:
plaintext = open(args.encrypt, "rb").read()
print("You can't specify both -e and -d")
exit(1)
except Exception:
encrypting = False
except Exception:
try:
plaintext = open(args.encrypt, "rb").read()
except Exception:
print("Input file error (did you specify -e or -d?)")
exit(1)
def lrot(n, d):
return ((n << d) & 0xff) | (n >> (8 - d))
if encrypting:
#…
arrow_forward
Convert from C to C++ .Thank you.
/ include all required libraries#include<stdio.h>#include<stdlib.h>#include<sys/types.h>#include<sys/wait.h>#include<unistd.h>
// variable to store number of generationsint num_generations;
// function to print the generationsvoid printGeneration(int n){// stop if number of generations are reachedif(n>num_generations)return;
// create a forkpid_t p = fork();
// generate new generationsif(p==0)printGeneration(n+1);else if(p>0)wait(0); // wait until child process terminates
// print the present generationif(p!=0){// if n is 0if(n==0)printf("Parent. "); // print parent// if n is 1else if(n==1)printf("Child. "); // print child// for all other caseselse{// print grandchildrenfor(int i=0;i<n-2;i++)printf("Great ");printf("Grandchild. ");}// print pid and ppidprintf("pid: %d ppid: %d\n",getpid(),getppid());}}
// main functionint main(int argc, char* argv[]){// if no arguments are providedif(argc==1){// print…
arrow_forward
A.) This script, named build.sh gets as argument the path to a folder where to find a source file and builds the executable.
B.) This script named script02.sh and take two arguments: a string to look for, and a path to the root folder to start the search from. The script should then explore recursively the folder hierarchy starting from the folder located at the path given as argument. At each folder encountered through the recursive search, the script should build a list of all text files in the folder and send this list, along with the target and the folder’s path, in a call to prog02 ( c program).
The output of your script should have the following format:
Recursive search for target <target> starting at <path>
Search depth: <depth>
Total number of folders: <number>
Total number of text files: <number>
<output produced by the C program>
arrow_forward
Problem
Given the target file f0 and candidate files f1-f20, there is an identical copy of f0 among the files f1-f20.
Write a python program to perform the following tasks:
· Read in each file and calculate the hex hash values by using SHA3 function with the length of 512 for all the given files f0, f1-f20.
· Save the hex hash values of each file as a new line in a .txt file named hash.txt file.
· Read hash values from hash.txt and compare them to find the identical copy of f0 among f1-f20.
· Write the finding result (the matched file name) back to hash.txt
Submission
• Name your project fileHash.py and submit both fileHash.py and hash.txt on Blackboard.
• The program should be properly documented.
• The program should have user friendly Input/Output design
• Duplicate work (or obviously similar one) will result in an F grade in the course.
Grading
1. Include comments as specified in the course syllabus. (10%)
2. Source code and results.
· Correctness (70%)
· User-friendliness…
arrow_forward
In this part, we add the CPU temperature ID of the Raspberry Pi to the MQTT stream.
Open a new terminal and create a new script by the name of py and add the following content to it.
import paho.mqtt.client as mqtt
import time
from subprocess import check_output
from re import findall
def get_temp():
temp = check_output(["vcgencmd","measure_temp"]).decode("UTF-8")
return(findall("\d+\.\d+",temp)[0])
def on_connect(client, userdata, flags, rc):
print("Connected with result code "+str(rc))
client.subscribe("etec224_mqtt_test/")
global Connected
Connected = True
Connected = False
client = mqtt.Client()
client.on_connect = on_connect
client.connect("broker.emqx.io", 1883, 60)
client.loop_start()
while Connected!= True:
#Wait Here
time.sleep(0.1)
try:
while True:
value = get_temp()
client.publish('etec224_mqtt_test/', value)
time.sleep(1)
except KeyboardInterrupt:
client.disconnect()…
arrow_forward
Write down Python code lines which will retrieve registered courses of a student via an API pointhttps://someuni.edu/stinfo in JSON format. Note that you need to specify the student by using a student ID with the"stid" parameter, specify that you want courses by setting the "info" parameter equal to "course", and specify the"format" to be "JSON".
arrow_forward
# The first message in the file was decrypted, its plaintext is:
plain_text = 'PICK UP THAT CAN CITIZEN'
# create a list of characters of the alphabet
alphabet_list = list('ABCDEFGHIJKLMNOPQRSTUVWXYZ')
# use list compression to create the alphabet mapping to the integers, starting
with 0
alphabet_int_list = [i for i in range(0,26)]
# create a list to hold the encrypted message strings
encrypted_messages_list = []
# open the file of encrypted messages in read mode, call it encrypted_messages_file
with open('encrypted_messages.txt', 'r') as encrypted_messages_file:
# readlines() reads all the lines of a file in one go and returns each line as
a string element in a list
encrypted_messages_list = encrypted_messages_file.readlines()
# we use list compression to, for each line in the list, remove the whitespace
(i.e. the new line character)
# and then store the results in a list
encrypted_messages_list = [line.strip() for line in encrypted_messages_list]…
arrow_forward
import pandas as pd
import matplotlib.pyplot as plt
dengue = pd.read_excel('Dengue.xlsx', header=0, index_col='Date', parse_dates=True, squeeze=True)
reg = dengue['Total']
reg = reg.reset_index()
reg.hist(bins = 100)
plt.grid(False)
plt.show()
arrow_forward
You can download;
restadata.txt : https://dosyam.org/2D1j/restdata.txt
restaurant.c :https://dosyam.org/2D1i/restaurant.c
Given code should be modify in C Language. Can someone help me please ?
#include <stdio.h> // Do not edit these directives or add another.#include <stdlib.h>#include <string.h>#include <time.h>
#define MAX 1000 // Do not edit this macro.
typedef struct // Do not edit this struct.{unsigned long restaurant_id;char restaurant_name[15];char description[127];double rate;char cuisine[31];unsigned short opening_year;unsigned long capacity;char city[31];char address[63];char owner[31];} RECORD_t, *RECORD;
/* DECLARE YOUR FUNCTION PROTOTYPES HERE. */void find_by_opening_year(unsigned short opening_year, RECORD *restaurant_array, unsigned long size, unsigned long start, unsigned long end, unsigned long *p_found_num, RECORD **p_found_restaurants);RECORD find_by_restaurant_name(char *restaurant_name, RECORD *restaurant_array, unsigned long size,…
arrow_forward
EXPLAIN THE BELOW PYTHON AND DBMS CODE STEP BY STEP WITH TERMINOLOGY
import osimport platformimport mysql.connectorimport pandas as pdimport datetimemydb = mysql.connector.connect(user='root', password='Arun@102003',host='localhost',database='food')mycursor=mydb.cursor()
def MenuSet(): #Function Food Booking System print("Enter 1 : To Add Cutomer details") print("Enter 2 : For Food Order") print("Enter 3 : Exit") try: #Using Exceptions For Validation userInput = int(input("Please Select An Above Option: ")) #Will Take Input From User except ValueError: exit("\nHy! That's Not A Number") #Error Message else: print("\n") #Print New Line if(userInput == 1): Customer() elif (userInput==2): orderitem() elif (userInput==3): Food() elif (userInput==4): OrderFood() elif (userInput==5): View() else: print("Enter correct choice. . . ")
MenuSet()
def Customer(): L=[] c_name=input("Enter…
arrow_forward
Load the given data set into Google Colab python
1. In USD, find the average salary of Data Scientists as well as the standard deviation.
2. List each unique Job Title
3. For each unique Job Title, find their average salary (USD) and standard deviations.
https://uc-bcf.instructure.com/courses/28339/files/5680123?verifier=fXym8nGyMe7CmvKYy7QmRDfcunF4DZwhuqakH1rL&wrap=1
arrow_forward
JAVA: Use natural split to split the bonus_txt file, then use natural merge to merge the two resulting files back together.
What is the value at index 100?
bonus.txt file
200
998 744 735 402 22 387 547 944 76 50 85 395 603 121 453 552 27 658 576 961 975 436 235 111 691 870 392 391 340 660 947 522 664 771 575 275 847 222 992 68 763 975 14 226 763 114 108 502 997 430 654 730 857 443 60 339 500 910 961 824 571 835 196 152 170 78 927 537 857 690 282 955 962 244 144 287 749 536 268 975 81 199 744 669 31 999 905 384 537 753 228 344 609 134 363 819 774 859 479 945 406 120 265 732 371 445 488 210 907 576 805 916 14 344 579 780 686 7 844 742 225 938 172 348 513 122 153 955 565 404 362 101 899 38 218 68 283 686 860 654 159 618 259 690 646 580 69 910 362 450 373 342 398 547 336 539 745 224 972 585 95 146 673 19 459 500 906 520 278 208 459 430 721 131 960 988 705 134 941 440 743 847 601 18 118 945 537 178 23 739 167 354 268 963 248 934 844 664 886 128
arrow_forward
Create a TST client with a static method that takes as command-line arguments an int value L and two file names and computes the L-similarity of the two documents: the Euclidean distance between the frequency vectors defined by the number of occurrences of each trigram divided by the number of trigrams. Include a static method main() that accepts an int value L as a command-line argument and a list of file names from standard input and prints a matrix displaying the L-similarity of all document pairs.
arrow_forward
import org.firmata4j.IODevice;import org.firmata4j.Pin;import org.firmata4j.firmata.FirmataDevice;import org.firmata4j.ssd1306.SSD1306;import java.io.IOException;import java.util.HashMap;import java.util.TimerTask;public class minorproj extends TimerTask {static String recLog = "\"/dev/cu.usbserial-0001\"";static IODevice myGroveBoard;private final SSD1306 theOledObject;private Pin MoistureSensor;private Pin WaterPump;private Pin Button;private int sampleCount;public minorproj(SSD1306 theOledObject, Pin Button, Pin MoistureSensor, Pin WaterPump) {this.theOledObject = theOledObject;this.Button = Button;this.MoistureSensor = MoistureSensor;this.WaterPump = WaterPump;this.sampleCount = 0;}double startTime = System.currentTimeMillis();@Overridepublic void run() {while (true) {{// check if button is pressedif (this.Button.getValue() == 1) {break; // exit loop and stop program}String VolValue = String.valueOf(MoistureSensor.getValue());System.out.println("Moisture Sensor Value:" +…
arrow_forward
import org.firmata4j.IODevice;import org.firmata4j.Pin;import org.firmata4j.firmata.FirmataDevice;import org.firmata4j.ssd1306.SSD1306;import java.io.IOException;import java.util.HashMap;import java.util.TimerTask;public class minorproj extends TimerTask {static String recLog = "\"/dev/cu.usbserial-0001\"";static IODevice myGroveBoard;private final SSD1306 theOledObject;private Pin MoistureSensor;private Pin WaterPump;private Pin Button;private int sampleCount;public minorproj(SSD1306 theOledObject, Pin Button, Pin MoistureSensor, Pin WaterPump) {this.theOledObject = theOledObject;this.Button = Button;this.MoistureSensor = MoistureSensor;this.WaterPump = WaterPump;this.sampleCount = 0;}double startTime = System.currentTimeMillis();@Overridepublic void run() {while (true) {{// check if button is pressedif (this.Button.getValue() == 1) {break; // exit loop and stop program}String VolValue = String.valueOf(MoistureSensor.getValue());System.out.println("Moisture Sensor Value:" +…
arrow_forward
Using the attached shell program (AirportCombos.cpp), create a list of strings to process and place on a STL DEQUE container.
Using the provided 3 char airport codes, create a 6 character string that is the origin & destination city pair. Create all the possible origin/destinations possible combinations from the airport codes provided. Load the origin/destination string onto a queue ( using the STL deque) for processing. Do not load same/same values, such as DALDAL, or LAXLAX. See comments in the program from more details.
Do not confuse the term queue with the 'queue' container. A queue provides data in a First-in First-out (FIFO) order. A 'queue' container adapter is a coding definition that can be built using vectors or lists or deques.
After loading the values, display all the values in the container.
#include <iostream>#include <string>
using namespace std;
const int AIRPORT_COUNT = 12;string airports[AIRPORT_COUNT] =…
arrow_forward
PLEASE FOR FRIDAY 24 :(
DMESG Analyzer
Implement a system log analyzer and classifier for GNU/Linux. In this particular case, a sample log output file is provided. It's highly recommended to implement in a Linux-based operating system. This challenge involves a proper struct design choice for the logs classification and storing.
Requirements
The program must be implemented in the C programming language.
You program will classify log entries by the <log_type>: prefix
Those log entries that don't follow the <log_type>: convention will go to the General classification.
Repeated log classes are not allowed.
Use the dmesg-analyzer.c template
The solution must use at least 3 of the following system calls:
open()
read()
write()
lseek()
close()
Don't forget to handle errors properly
Sample Execution
./dmesg-analizer -input dmesg.txt -output dmesg_report.txt
Sample Expected output file dmesg_report.txt
General: [ 0.000000] Linux version 4.15.0-45-generic (buildd@lgw01-amd64-031)…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
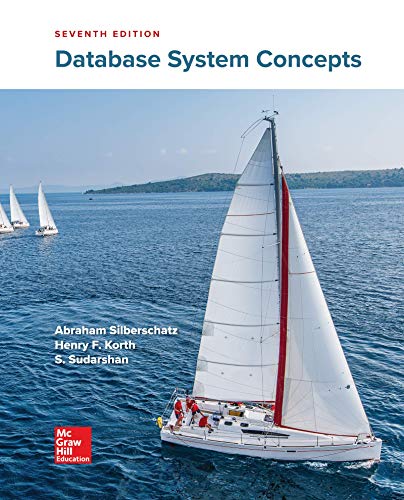
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
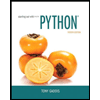
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
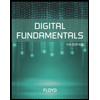
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
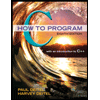
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
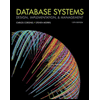
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
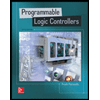
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- /* Filename: logserver.c *//*******************************************************************************************modify this program to log the message from clients to a log file. ******************************************************************************************/#include<stdio.h>#include<sys/ipc.h>#include<sys/shm.h>#include<sys/types.h>#include<string.h>#include<errno.h>#include<stdlib.h> #define BUF_SIZE 1024#define SHM_KEY 0x1234 struct shmseg {int cnt;int new;int complete;char buf[BUF_SIZE];}; int main(int argc, char *argv[]) {int shmid;struct shmseg *shmp;shmid = shmget(SHM_KEY, sizeof(struct shmseg), 0666|IPC_CREAT);if (shmid == -1) {perror("Shared memory");return 1;}// Attach to the segment to get a pointer to it.shmp = shmat(shmid, NULL, 0);if (shmp == (void *) -1) {perror("Shared memory attach");return 1;}/* Transfer blocks of data from shared memory to log file if the message from client set shmp->complete ==1, the we…arrow_forwardC PROGRAMMING. using the following program as a start point: /* * NetworkServer.c * ProgrammingPortfolio Skeleton * */ /* You will need to include these header files to be able to implement the TCP/IP functions */#include <stdio.h>#include <stdlib.h>#include <string.h>#include <sys/types.h>#include <netinet/in.h>#include <netdb.h>#include <fcntl.h>#include <unistd.h>#include <errno.h>#include <sys/socket.h> /* You will also need to add #include for your graph library header files */ int main(int argc, const char * argv[]){ int serverSocket = -1; printf("Programming Portfolio 2022 Implementation\n"); printf("=========================================\n\n"); /* Insert your code here to create, and the TCP/IP socket for the Network Server * * Then once a connection has been accepted implement protocol */ TASK: you are to write a TCP/IP server that can build up a graph of a network ofnetworks…arrow_forwardIN SCALA PLEASE COULD YOU COMPLETE THE CODE OF FUNCTIONS: get_csv_url, process_ratings, process_movies, groupById, favourites, suggestions and recommendations import io.Source import scala.util._ // (1) Implement the function get_csv_url which takes an url-string // as argument and requests the corresponding file. The two urls // of interest are ratings_url and movies_url, which correspond // to CSV-files. // // The function should ReTurn the CSV-file appropriately broken // up into lines, and the first line should be dropped (that is without // the header of the CSV-file). The result is a list of strings (lines // in the file). def get_csv_url(url: String) : List[String] = ??? val ratings_url = """https://nms.kcl.ac.uk/christian.urban/ratings.csv""" val movies_url = """https://nms.kcl.ac.uk/christian.urban/movies.csv""" // testcases //----------- //: //val movies = get_csv_url(movies_url) //ratings.length // 87313 //movies.length // 9742 // (2) Implement two functions that…arrow_forward
- What does the following code segment and subroutine do? .equ SIZE =128.equ TABLE_L =$60.equ TABLE_H =$00 rjmp RESET .def A =r13.def B =r14.def cnt2 =r15.def cnt1 =r16.def endL =r17.def endH =r18 mystery: mov ZL,endLmov ZH,endHmov cnt2,cnt1i_loop: ld A,Z ld B,-Z cp A,B brlo L1 st Z,A std z+1,BL1: dec cnt2 brne i_loop dec cnt1 brne mystery ret Converts temperature values from one scale to another Adds a constant to a table of bytes Sorts numbers in ascending order Converts ASCII characters from lower case to upper casearrow_forwardimport java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import org.json.JSONObject; public class Myclass { public static void main(String[] args) throws Exception { try { URL url = new URL("https://parseapi.back4app.com/classes/City?count=1&limit=10&keys=name,population"); HttpURLConnection urlConnection = (HttpURLConnection)url.openConnection(); urlConnection.setRequestProperty("X-Parse-Application-Id", "mxsebv4KoWIGkRntXwyzg6c6DhKWQuit8Ry9sHja"); // This is the fake app's application id urlConnection.setRequestProperty("X-Parse-Master-Key", "TpO0j3lG2PmEVMXlKYQACoOXKQrL3lwM0HwR9dbH"); // This is the fake app's readonly master key try { BufferedReader reader = new BufferedReader(new InputStreamReader(urlConnection.getInputStream())); StringBuilder stringBuilder = new…arrow_forward#The Iris Dataset import sklearn.datasetsimport matplotlib.pyplot as plt import numpy as np import scipy iris = sklearn.datasets.load_iris() Write a function that takes in an index i and prints out a verbose desciption of the species and measurements for data point i. For example:Data point 5 is of the species setosaIts sepal length (cm) is 5.4Its sepal width (cm) is 3.9Its petal length (cm) is 1.7Its petal width (cm) is 0.4arrow_forward
- STEP 1: Begin work within your Jupyter Notebook by importing the following modules: import numpy as np import pandas as pd from matplotlib import pyplot as plt import re Jupyter Notebooks Q1. Within your Jupyter Notebook, write the code for a Python function called def parseWeatherByYear(year) : This function will parse an html page containing weather for an entire year of data for the city of Toronto. The html pages containing weather data can be downloaded from: https://www.extremeweatherwatch.com/cities/toronto/year-2023 The file to parse for this lab however can be downloaded here: https://matrix.senecacollege.ca/~danny.abesdris/prg550.232/labs/lab6/torontoWeather.2023.html The html file itself contains markers as where to begin parsing the data to extract. The 3 pieces of data that must be extracted consist of the high and low temperatures (in degrees Celsius) as well as the amount of precipitation (in cm) for every day so far in the current year (2023). A series…arrow_forwardThe questions should be solved using Linux Bash Scripting. In the file there should be solution code and one/two screenshot for running of program. Student id and names should be written inside the file. The assignment should be sent as PDF. Upload Link: https://forms.office.com/r/JfpSa2dDi8 Question 1. Read "n" and generate following pattern according to givennas below. Ex: for given value forn:4\[ \begin{array}{l} 1 \\ 23 \\ 456 \\ 78910 \end{array} \]arrow_forwardRewrite the program in Example 3-1. DigestThread class to output the result of each file on an output file for each one by assigning a new name for the output file. The language is Java Example 3-1. DigestThread import java.io.*; import java.security.*; import javax.xml.bind.*; // for DatatypeConverter; requires Java 6 or JAXB 1.0 public class DigestThread extends Thread { private String filename; public DigestThread(String filename) { this.filename = filename; } @Override public void run() { 56 | Chapter 3: Threads www.it-ebooks.info try { FileInputStream in = new FileInputStream(filename); MessageDigest sha = MessageDigest.getInstance("SHA-256"); DigestInputStream din = new DigestInputStream(in, sha); while (din.read() != -1) ; din.close(); byte[] digest = sha.digest(); StringBuilder result = new StringBuilder(filename); result.append(": "); result.append(DatatypeConverter.printHexBinary(digest)); System.out.println(result); } catch (IOException ex) {…arrow_forward
- ; initialized data heresection .data msg db "Print String!", 0xamsglen equ $-msg ; string length in bytesSTDOUT equ 1 ; standard output deviceSYS_write equ 1 ; write messageSYS_exit equ 60 ; exit programEXIT_OK equ 0 ; return value ; code goes heresection .text ; The '_start' label identifies the program's entry point for the; linker for the 'nasm' assembler. If you use 'gcc', the entry point; is labeled 'main'global _start_start: ; send message string to standard output mov rsi, msg ; text address mov rdx, msgln ; string length (error here) mov rdi, STDOUT ; output device mov rax, SYS_write ; rax holds the function syscall ; system…arrow_forwardIn python, I'd like to split the contents in an XML file into separate parallel arrays. An example would be: <PROFILE> <STUDENT> <NAME>Jim</NAME> <AGE>18</AGE> <GRADE>95%</GRADE> </STUDENT> <STUDENT> <NAME>Andrew</NAME> <AGE>16</AGE> <GRADE>82%</GRADE> </STUDENT> </PROFILE> into: name = ['Jim', 'Andrew'] age = ['18', '16'] grade = ['95%', '82%']arrow_forwardPython Data = { "logs": [ { "logType": “xxxxx” }, { "logType": “43435” }, { "logType": “count” }, { "logType": “4452” }, { "logType": “count” }, { "logType": “count” }, { "logType": “43435” }, { "logType": "count" }, { "logType": "count" } ] } So, please count the total of "logType": “count” My desired output: { "count”: 5 }arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
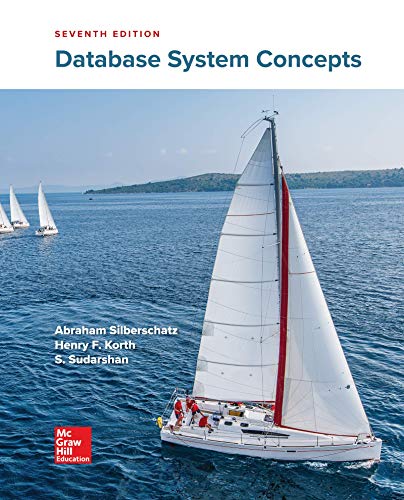
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
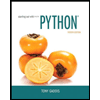
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
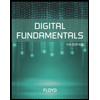
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
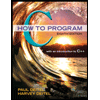
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
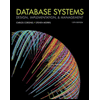
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
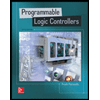
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education