import org.firmata4j.IODevice; import org.firmata4j.Pin; import org.firmata4j.firmata.FirmataDevice; import org.firmata4j.ssd1306.SSD1306; import java.io.IOException; import java.util.HashMap; import java.util.TimerTask; public class minorproj extends TimerTask { static String recLog = "\"/dev/cu.usbserial-0001\""; static IODevice myGroveBoard; private final SSD1306 theOledObject; private Pin MoistureSensor; private Pin WaterPump; private Pin Button; private int sampleCount; public minorproj(SSD1306 theOledObject, Pin Button, Pin MoistureSensor, Pin WaterPump) { this.theOledObject = theOledObject; this.Button = Button; this.MoistureSensor = MoistureSensor; this.WaterPump = WaterPump; this.sampleCount = 0; } double startTime = System.currentTimeMillis(); @Override public void run() { while (true) { { // check if button is pressed if (this.Button.getValue() == 1) { break; // exit loop and stop program } String VolValue = String.valueOf(MoistureSensor.getValue()); System.out.println("Moisture Sensor Value:" + VolValue); double Moisture = (double)( ((MoistureSensor).getValue() * 5)/ 1023); HashMap Value = new HashMap<>();//used to store a mapping of keys to values. //the keys are of the data type Double and the values are of the data type Integer. double elapsedTime = (System.currentTimeMillis() - startTime) / 1000.0; if (this.MoistureSensor.getValue() >= 710) { try { System.out.println("Soil is Dry.Water!!"); theOledObject.getCanvas().clear(); // clear contents first. theOledObject.getCanvas().drawString(0, 0, "Soil is Dry.Water!!::" + String.format("%.2f", Moisture)); theOledObject.display(); WaterPump.setValue(1); } catch (IOException e) { throw new RuntimeException(e); } } else if (this.MoistureSensor.getValue() <= 510) { try { System.out.println("Soil is WET.Do Not Water!!"); theOledObject.getCanvas().clear(); // clear contents first. theOledObject.getCanvas().drawString(0, 0, "Soil is WET.Do Not Water!!::" + String.format("%.2f", Moisture)); theOledObject.display(); WaterPump.setValue(0); } catch (IOException e) { throw new RuntimeException(e); } } else try { System.out.println("Soil is Moist.Needs More Water!!"); theOledObject.getCanvas().clear(); // clear contents first. theOledObject.getCanvas().drawString(0, 0, "Soil is Moist.Needs More Water!!::" + String.format("%.2f", Moisture)); theOledObject.display(); WaterPump.setValue(1); } catch (IOException e) { throw new RuntimeException(e); } } } ---- create another class that makes this code run
import org.firmata4j.IODevice;
import org.firmata4j.Pin;
import org.firmata4j.firmata.FirmataDevice;
import org.firmata4j.ssd1306.SSD1306;
import java.io.IOException;
import java.util.HashMap;
import java.util.TimerTask;
public class minorproj extends TimerTask {
static String recLog = "\"/dev/cu.usbserial-0001\"";
static IODevice myGroveBoard;
private final SSD1306 theOledObject;
private Pin MoistureSensor;
private Pin WaterPump;
private Pin Button;
private int sampleCount;
public minorproj(SSD1306 theOledObject, Pin Button, Pin MoistureSensor, Pin WaterPump) {
this.theOledObject = theOledObject;
this.Button = Button;
this.MoistureSensor = MoistureSensor;
this.WaterPump = WaterPump;
this.sampleCount = 0;
}
double startTime = System.currentTimeMillis();
@Override
public void run() {
while (true) {
{
// check if button is pressed
if (this.Button.getValue() == 1) {
break; // exit loop and stop program
}
String VolValue = String.valueOf(MoistureSensor.getValue());
System.out.println("Moisture Sensor Value:" + VolValue);
double Moisture = (double)( ((MoistureSensor).getValue() * 5)/ 1023);
HashMap<Double, Integer> Value = new HashMap<>();//used to store a mapping of keys to values.
//the keys are of the data type Double and the values are of the data type Integer.
double elapsedTime = (System.currentTimeMillis() - startTime) / 1000.0;
if (this.MoistureSensor.getValue() >= 710) {
try {
System.out.println("Soil is Dry.Water!!");
theOledObject.getCanvas().clear(); // clear contents first.
theOledObject.getCanvas().drawString(0, 0, "Soil is Dry.Water!!::" + String.format("%.2f", Moisture));
theOledObject.display();
WaterPump.setValue(1);
} catch (IOException e) {
throw new RuntimeException(e);
}
} else if (this.MoistureSensor.getValue() <= 510) {
try {
System.out.println("Soil is WET.Do Not Water!!");
theOledObject.getCanvas().clear(); // clear contents first.
theOledObject.getCanvas().drawString(0, 0, "Soil is WET.Do Not Water!!::" + String.format("%.2f", Moisture));
theOledObject.display();
WaterPump.setValue(0);
} catch (IOException e) {
throw new RuntimeException(e);
}
} else
try {
System.out.println("Soil is Moist.Needs More Water!!");
theOledObject.getCanvas().clear(); // clear contents first.
theOledObject.getCanvas().drawString(0, 0, "Soil is Moist.Needs More Water!!::" + String.format("%.2f", Moisture));
theOledObject.display();
WaterPump.setValue(1);
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
----
create another class that makes this code run

Step by step
Solved in 3 steps

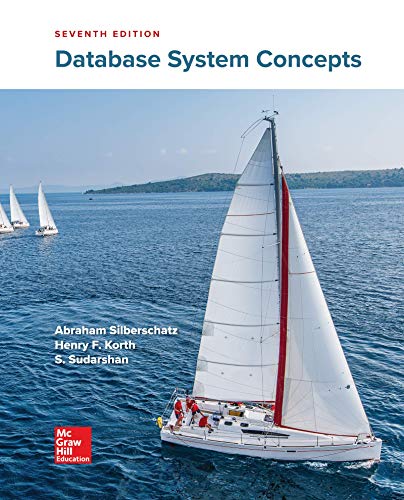
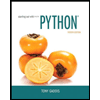
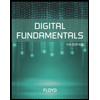
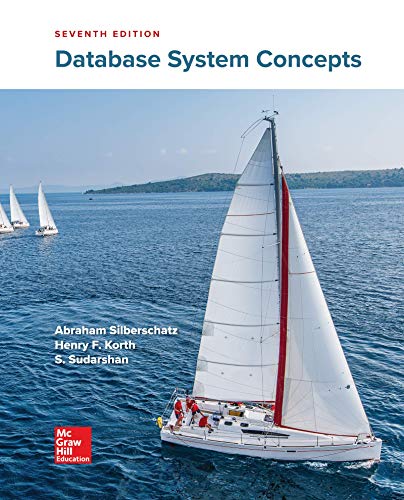
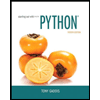
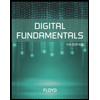
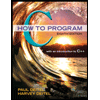
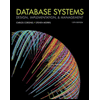
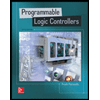