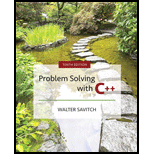
Program Plan:
- Include required header files.
- Declaration of function prototype.
- Definition for function “main()”.
- Declare and initialize the variable “var”.
- Print the value before calling function
- Call the function “addOne()”.
- Print the value after calling function.
- Return the value “0”.
- Definition of function “addOne()”.
- Increment the pointer variable.

The given program is to add one to the integer referenced by “ptrNum” by using reference parameter syntax.
Explanation of Solution
//Include required header files
#include<iostream>
using namespace std;
//Declaration of function header
void addOne(int *ptrNum);
//Definition of function main()
int main()
{
//Declare and initialize the variable "var".
int var = 10;
//Print the value before calling function
cout << "Value before calling function = " << var << '\n';
//Call the function
addOne(&var);
//Print the value after calling function
cout << "Value after calling function = " << var << '\n';
//Return the value "0"
return 0;
}
//Definition of function "addOne()"
void addOne(int *ptrNum)
{
//Increment the pointer variable
*ptrNum = *ptrNum + 1;
}
Output:
Value before calling function = 5
Value after calling function = 6
Want to see more full solutions like this?
Chapter 9 Solutions
Problem Solving with C++ (10th Edition)
- Write a function in C language which takes an integer as parameter and returns the sum of all non zero digits in the number passed as argument to the function.arrow_forwardC Programming Problem : Write a C program with a function that takes an integer value (1 <- integer value < 9999) and returns the number with its digits reversed. For example, given the number 6798, the function should return 8976. Those two- original number and reversed number- numbers will pass to another function as parameters and calculate their sum. The program should use the function reverseDigits to reverse the digits and SumOriginalReverse to calculate their sum. Your output should appear in the following format: Output reverse digiS 4321 Main Enter a number between 1 and 9999: 6798 The number with its digits reversed is: 8976 6798 + 8976 - 15774 12.34 JomoriginalRevers Enter a number between I and 9999: 6655 The number with its digits reversed is: 5566 6655 + 5566 = 12221 Enter a number between 1 and 9999: 8 The number with its digits reversed is: 8 8 + 8 = 16 Enter a number between 1 and 9999: 123 The number with its digits reversed is: 321 123 + 321 = 444 Enter a number…arrow_forwardIn C++, attach the function code.arrow_forward
- A program uses a function named convert() in addition to its main function. The function main() declares the variable x within its body and the function convert declares two variables y and z within its body, z is made static. A fourth variable m is declared ahead(ie at top) of both the functions. State the visibility and lifetime of each of these variables. Write the code in C++ language. Thanks in advancearrow_forwardin C++ Write the definition of a value-returning function that accepts two integer parameters and returns the larger one divided by the smaller one.arrow_forwardWhat does it mean to provide parameters to a function in the right order when it accepts more than one?arrow_forward
- Write a function in C language which takes an integer as parameter and returns the number of non zero digits in the number passed as argument.arrow_forwardWrite a C++ program to facilitate the usage of several string-handling functions. The program should present a menu for the choices available. The options might be to create, insert, append, erase, reverse, and print. The program should ask the user what required by the function to prepare the real function call. For instance, for the insert function, the string might be printed on the screen as a reminder first, and then the start and finish indexes might be requested from the user. You should be aware of the run-time issues that might result due to user mistakes. Try to prevent them!arrow_forwardUsing a pointer as the return value is considered a poor practise in C. For example, how does dynamic memory allow us to return an object pointer from a function safely?arrow_forward
- In C++, If you had the double-pointer above and also had these variables: type x, * q; And had executed these statements: q = &x; p = &q; How would a function given p by value be able to change the contents of x?arrow_forwardIn C++ programing language, Write the definition of the function template that swaps the content of two variablesarrow_forwardIn C/C++, Function overloading is the process of creating several functions that have exactly the same name. In order to overload successfully, each function must have: A. A different parameter list B. A different number of parameters and a different return type C. An integer on the end of the function name, such as func1()and func2() D. Either A or B E. Both A and Carrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
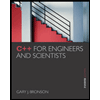