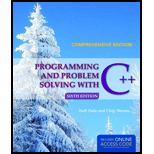
Concept explainers
Program plan:
- Declare a variable file of ifstream type to read the input from the file.
- Declare a variable letter of type char to store the letter read from the file.
- Declare 6 variables upperCounter, lowerCounter, blank, digit, punctuation, allElseCounter of type int to store the counter of each type.
- Declare a variable inFile of type String to store the name of the file read from the user.
- A do- while loop is used to read letters from the file and to increment the each type of counter.
- A switch is used to check for each type of letters and to increment the counter inside do-while loop.
Program description:
The main purpose of the program is to summarize all the contents of the file in a table with details of each type of character scanned.

Explanation of Solution
Program:
//inclusion of header files #include <fstream> #include <iostream> #include <iomanip> #include <cctype> //using namespace using namespace std; //main function int main() { ifstream file; //fstream input char letter; // Declaration and Initialization of variables int upperCounter = 0; // counter for uppercase letters int lowerCounter = 0; // counter for lowercase letters int blank = 0; // counter for blanks int digit = 0; //counter for digits int punctuation = 0; //counter for punctuation int allElseCounter = 0; //counter for Remaining counters //Declare variable to store filename string inFile; cout<<"Enter the filename to be processed" << endl; cin>>inFile; //open the file file.open(inFile.c_str()); //if file doesn’t get opened if (!file) { cout<< "Filename doesn’t exist." << endl; return 1; } //else read the characters file.get(letter); // Input one letter do // process each letter { if (isupper(letter)) upperCounter++; else if (islower(letter)) lowerCounter++; else if (isdigit(letter)) digit++; else switch (letter) { case ' ' : blank++; break; case '.' : case '?' : case '!' : punctuation++; break; default : allElseCounter++; break; } file.get(letter); } while (file); // Calculate total float total = upperCounter + lowerCounter + blank + digit + punctuation + allElseCounter; cout<<"Summary of letters: "<< inFile << endl; // Letters of each type cout<<fixed<<setprecision(3)<<"Percentage of uppercase letters:"<<upperCounter / total* 100<<endl; cout<<fixed<<setprecision(3)<< "Percentage of lowercase letters:" << lowerCounter / total * 100 << endl; cout<<fixed<<setprecision(3)<< "Percentage of blanks: "<< blank / total * 100 << endl; cout<<fixed<<setprecision(3)<< "Percentage of digits: "<< digit / total * 100 << endl; cout<<fixed<<setprecision(3)<< "Percentage of end-of-sentence: "<< "punctuation "<< punctuation / total * 100 << endl; return 0; }
Explanation:
In this program, first of all, the user is asked to enter the filename which needs to be processed. After entering the filename, all the characters of it are scanned to get a summary table of each type of letters. Each letter is scanned for uppercase letters, lowercase letters, for digits, blanks, and for any puctuations. Each type counter get incremented if the letter scanned is of that type. For example, if the letter scanned is of uppercase then the counter for uppercase letters will get incremented and so on. In the end, complete summary of all types of letters is printed on the screen with the filename which is scanned as shown in the output.
Output:
Contents of the file Unclewill.txt:
Want to see more full solutions like this?
Chapter 7 Solutions
Programming and Problem Solving With C++
- Vectors of string given below for code. THUMBS DOWN FOR INCORRECT.arrow_forward6. A palindrome is a number or text phrase that reads the same backwards or forwards. For example, each of the following five-digit integers is a palindrome: 12321, 55555, 45554 and 11611. Write a program that reads a 5 digit integer and determines whether it is a palindrome. Hint: One way to do this is to use division (/) and modulus (%) operators to separate the number into individual digits.arrow_forwardBroken Cabins Problem Statement: There is an Office consisting of m cabins enumerated from 1 to m. Each cabin is 1 meter long. Sadly, some cabins are broken and need to be repaired. You have an infinitely long repair tape. You want to cut some pieces from the tape and use them to cover all of the broken cabins. To be precise, a piece of tape of integer length t placed at some positions will cover segments 5,5+1-sit-1. You are allowed to cover non-broken cabins, it is also possible that some pieces of tape will overlap. Time is money, so you want to cut at most k continuous pieces of tape to cover all the broken cabins. What is the minimum total length of these pieces? Input Format The first line contains three integers n,m and k(1sns10°, namsloº, Isksn) - the number of broken cabins, the length of the stick and the maximum number of pieces you can use The second line contains n integers bl,b2,bn (Isbism) - the positions of the broken cabins. These integers are given in increasing…arrow_forward
- C++ One way to create a pseudo-random three digit number (and we are including one- and two-digit numbers as well) is rand()%1000. That gives numbers from 000 to 999, which is just what we want. Write a program that generates a random number and asks the user to guess what the number is. If the user's guess is too high then the program should display "Too high, try again." If the user's guess is lower than the random number the program should say "Too low, try again." The program should use a loop that repeats until the user correctly guesses the random number. The program should keep track of the number of guesses the user makes. When the user correctly guesses the random number, the program should display the number of guesses. As specified, have the program tell the player how many guesses were used. A good player should always be able to find a three-digit number in ten or fewer guesses. The programs needs to include a menu that allows the user to select easy (3 digit number),…arrow_forwardMATLAB QUESTION - As of 2018, the population of "A city" is 646 thousand and the population of "B city" is 269 thousand. While the population of "A city" decreases by 7.1% every year, the population of "B city" increases by 12.9%. According to this information, write a program that calculates the year in which the population of "B city" passed the population of "A city".arrow_forwardthat adds two very large integers entered by the user on the keyboard write the program. Very large integers represent standard data types (long int, double, etc.) you cannot collect it using. Reading and adding numbers from the keyboard as a string As you learned the process in elementary school, starting from the units digit, you can add the mutual numbers one by one. you must realize by collecting. The numbers that the user has entered into a different number of digits. In order to have it, your program should work. The output your program is the example below should be compatible with the program outputs. In the program output, as in the addition operation the numbers and the total should be written one under the other, with the mutual digits aligned Sample program output - 1: First number: 1111111111111111111111111 Second number: 9999999999 1111111111111111111111111 9999999999 1111111111111120000000000 Sample program output - 2: First number: 11 Second number:…arrow_forward
- Transient PopulationPopulations are affected by the birth and death rate, as well as the number of people who move in and out each year. The birth rate is the percentage increase of the population due to births and the death rate is the percentage decrease of the population due to deaths. Write a program that displays the size of a population for any number of years. The program should ask for the following data: The starting size of a population P The annual birth rate (as a percentage of the population expressed as a fraction in decimal form)B The annual death rate (as a percentage of the population expressed as a fraction in decimal form)D The average annual number of people who have arrived A The average annual number of people who have moved away M The number of years to display nYears Write a function that calculates the size of the population after a year. To calculate the new population after one year, this function should use the formulaN = P + BP - DP + A - Mwhere N is the…arrow_forwardAssume that name and age have been declared suitably for storing names (like "Abdullah", "Alexandra" and "Zoe") and ages respectively. Write some code that reads in a name and an age and then prints the message "The age of NAME is AGE." where NAME and AGE are replaced by the values read in for the variables name and age. For example, if your code read in "Rohit" and 70 then it would print out "The age of Rohit is 70.".arrow_forwardProblem Description: In the Whimsical Library, each book is enchanted with a magical code to ensure the protection of knowledge. The validation process for these magical codes involves a combination of mathematical spells. The validation process is described as follows: Take the sum of the digits at odd positions from left to right. Take the sum of the squared digits at even positions from left to right. If the absolute difference between the results from step 1 and step 2 is a prime number, the book code is considered valid; otherwise, it is invalid. Example 1: Consider the magical book code 86420137. Step 1. Take the sum of the digits at odd positions from left to right. 8+4+0+3=15 Step 2. Take the sum of the squared digits at even positions from left to right. 6^2+2^2+1^2+7^2=36+4+1+49 = 90 Step 3. If the absolute difference between the results from step 1 and step 2 is a prime number. 115-901 = 75 (not a prime number) Book code 86420137 is invalid. Example 2: Now, let's examine the…arrow_forward
- AC that adds two very large integers entered by the user on the keyboard write the program. Very large integers represent standard data types (long int, double, etc.) you cannot collect it using. Reading and adding numbers from the keyboard as a string As you learned the process in elementary school, starting from the units digit, you can add the mutual numbers one by one. you must realize by collecting. The numbers that the user has entered into a different number of digits. In order to have it, your program should work. The output of your program is the example below should be compatible with the program outputs. In the program output, as in the addition operation the numbers and the total should be written one under the other, with the mutual digits aligned. Sample program output - 1: First number: 111111111111111111111111 Second number: 999999999 11111111111u111. 9999999999 1111111111111120000000000 Sample program output - 2: First number: 11 Second number:…arrow_forwardPython task: Task OverviewYour task is to implement a variation of the classic word game Hangman, which involves playersguessing the letters in a word chosen at random with a finite number of guesses. While there arealternate versions such as category Hangman and Wheel of Fortune, which involve playersguessing idioms, places, names and so on, we will be sticking with the traditional version.If you are unfamiliar with the rules of the game, please read the following before starting:http://en.wikipedia.org/wiki/Hangman_(game). You can also play an online version here. Don’tbe intimidated - You’ll be given some skeleton code to get you started, it's easier than it looks! You will implement a function called main that allows users to play an interactive hangman gameagainst the computer. The computer should pick a word, and players should then try to guess lettersin the word until they win or run out of guesses.Here is the overarching behaviour we expect:1. The program should load a list of…arrow_forwardA Fibonacci Number sequence is the series of numbers: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ... The next number is calculated by adding up the two numbers before it. Write a program called fib.cpp. The program will print out the n" Fibonacci Numbers using a function called: void FibonacciNumber (int n, int& fib_no) This function will calculate the n term in the Fibonacci Numbers and return in fib_no. By definition, the function returns 0 for n=1, and returns 1 for n = 2. For the subsequence number: nth = (n - 2)th + (n - 1)th You need to verify the input n > 0. Task 2 Sample Output Please enter the nth term Fibonacci number to compute: -1 Please enter a number greater than 0! Please enter the nth term Fibonacci number to compute: 12 The Fibonacci series is: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89 The 12th. term is: 89arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
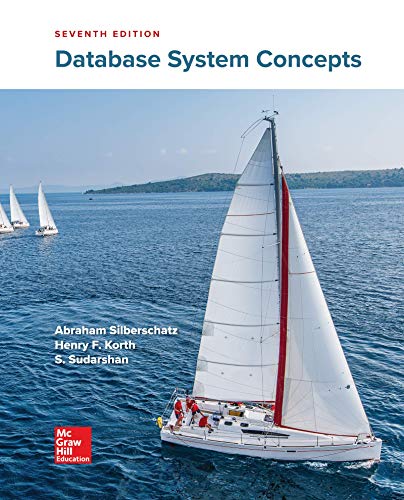
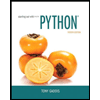
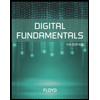
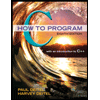
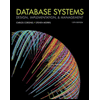
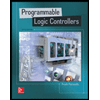