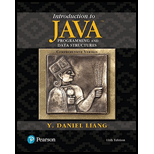
Program Plan:
- Include the required import statement.
- Define the main class.
- Define the main method using public static main.
- Allocate memory to the class “Test”.
- Define the “Test” class.
- Declare the object for the BST.
- Display the initial height of the tree.
- Get the input string from the user.
- Display the height of the tree.
- Call the “breathFirstTraversal” method.
- Create an object and set the string values to that object.
- Again call the “breathFirstTraversal” method.
- Create an integer object for class and set the integer values to that object.
- Display the results.
- Define the “BST” class.
- Declare the required variables.
- Create a default BST class.
- Create a binary tree from an array of objects.
- The “height” method will return the height of the tree.
- Define “breathFirstTraversal” method.
- Declare the linked list.
- Add the values to the list.
- If the list is not empty print the elements.
- If the left node is not null, add the value to the left subtree.
- If the right node is not null, add the value to the right subtree.
- Define the “search” method.
- Start the traverse from the root of the tree.
- If the search element is in the left subtree set that value in “current” variable otherwise set the “current” variable as right subtree value.
- Define the “insert” method.
- If the root is null create the tree otherwise insert the value into left or right subtree.
- Define the “createNewNode”
- Return the result of new node creations.
- Define the “inorder”
- Inorder traverse from the root.
- Define the protected “inorder” method
- Traverse the tree according to the inorder traversal concept.
- Define the “postorder”
- Postorder traverse from the root.
- Define the protected “postorder” method
- Traverse the tree according to the postorder traversal concept.
- Define the “preorder”
- Preorder traverse from the root.
- Define the protected “preorder” method
- Traverse the tree according to the preorder traversal concept.
- Define the “TreeNode” class
- Declare the required variables.
- Define the constructor.
- Define the “getSize” method.
- Return the size.
- Define the “getRoot” method
- Return the root.
- Define the “java.util.ArrayList” method.
- Create an object for the array list.
- If the “current” is not equal to null, add the value to the list.
- If the “current” is less than 0, set the “current” as left subtree element otherwise set the “current” as right subtree element.
- Return the list.
- Define the “delete” method.
- If the “current” is not equal to null, add the value to the list.
- If the “current” is less than 0, delete the “current” as left subtree element otherwise delete the “current” as right subtree element.
- Return the list.
- Define the “iterator” method.
- Call the “inorderIterator” and return the value.
- Define the “inorderIterator”
- Create an object for that method and return the value
- Define the “inorderIterator” class.
- Declare the variables.
- Define the constructor.
- Call the “inorder” method.
- Define the “inorder” method.
- Call the inner “inorder” method with the argument.
- Define the TreeNode “inorder” method.
- If the root value is null return the value, otherwise add the value into the list.
- Define the “hasNext” method
- If the “current” value is less than size of the list return true otherwise return false.
- Define the “next” method
- Return the list.
- Define the “remove” method.
- Call the delete method.
- Clear the list then call the “inorder” method.
- Define the “clear” method
- Set the values to the variables
- Define the interface.
- Declare the required methods.
- Define the required methods.
- Define the main method using public static main.

The below program will add the “breathFirstTraversal” method in the given BST class as follows:
Explanation of Solution
Program:
//import statement
import java.util.*;
//class Test
public class Test
{
// main method
public static void main(String[] args)
{
//allocation of memory
new Test();
}
//definition of "Test"
public Test()
{
//declaration an object of BST
BST<String> tree = new BST<>();
//get the input from the user
System.out.print("The height of tree is " + tree.height());
Scanner input = new Scanner(System.in);
//print the statement
System.out.print("\nEnter strings: ");
//check the condition
for (int i = 0; i < 6; i++)
{
//get the input from the user
String s = input.next();
//insert the value
tree.insert(s.trim());
}
//get the input from the user
System.out.print("\nThe height of tree is " + tree.height());
//insert the value to the tree
tree.insert("Green");
//get the input from the user
System.out.print("\nThe height of tree is " + tree.height());
//print the new line
System.out.println();
//call the "breadthFirstTraversal" method
tree.breadthFirstTraversal();
//create the object for the BST
BST<String> tree1 = new BST<>(new String[]
{"Tom", "George", "Jean", "Jane", "Kevin", "Peter", "Susan",
"Jen", "Kim", "Michael", "Michelle"});
//print the statement
System.out.print("\nThe breadth-first traversal is ");
//call the "breadthFirstTraversal" method
tree1.breadthFirstTraversal();
//get the input from the user
System.out.print("\nThe height of tree1 is " + tree1.height());
//create the object for the BST
BST<Integer> tree2 =
new BST<>(new Integer[]{50, 45, 35, 48, 59, 51, 58});
//print the statement
System.out.print("\nThe breadth-first traversal for tree2 is ");
//call the "breadthFirstTraversal" method
tree2.breadthFirstTraversal();
//get the input from the user
System.out.print("\nThe height of tree2 is " + tree2.height());
}
//definition of "BST" class
public class BST<E extends Comparable<E>> implements Tree<E>
{
//declare the variables
protected TreeNode<E> root;
protected int size = 0;
//create a default binary tree
public BST()
{
}
//create a binary tree from an array of objects
public BST(E[] objects)
{
//check the condition
for (int i = 0; i < objects.length; i++)
//insert the values
insert(objects[i]);
}
// definition of "height"
public int height()
{
//returns the height of this binary tree
return height(root);
}
// definition of "height"
private int height(TreeNode root)
{
//check the condition
if (root == null)
{
//return the value
return -1;
}
else
{
//return the value
return 1 + Math.max(height(root.left), height(root.right));
}
}
// definition of "breadthFirstTraversal" method
public void breadthFirstTraversal()
{
//declaration of linked list
java.util.LinkedList <TreeNode<E>> queue =
new java.util.LinkedList<TreeNode<E>>();
//check the condition
if (root == null)
//return statement
return;
//add the value to the queue
queue.add(root);
//check the condition
while (!queue.isEmpty())
{
//declaration of variable
TreeNode<E> node = queue.removeFirst();
//print the statement
System.out.print (node.element + " ");
//check the condition
if (node.left != null)
//add the value to the queue
queue.add(node.left);
//check the condition
if (node.right != null)
//add the value to the queue
queue.add(node.right);
}
}
//definition of "search" method
public boolean search(E e)
{
//start from the root
TreeNode<E> current = root;
//check the condition
while (current != null)
{
//check the condition
if (e.compareTo(current.element) < 0)
{
//set the value
current = current.left;
}
//check the condition
else if (e.compareTo(current.element) > 0)
{
//set the value
current = current.right;
}
//otherwise
else
//return statement
return true;
}
//return statement
return false;
}
//definition of "insert" method
public boolean insert(E e)
{
//check the condition
if (root == null)
//create a new root
root = createNewNode(e);
//otherwise
else
{
// locate the parent node
TreeNode<E> parent = null;
TreeNode<E> current = root;
//check the condition
while (current != null)
//check the condition
if (e.compareTo(current.element) < 0)
{
//set the value
parent = current;
current = current.left;
}
//check the condition
else if (e.compareTo (current.element) > 0)
{
//set the value
parent = current;
current = current.right;
}
//otherwise
else
//return statement
return false;
//check the condition
if (e.compareTo (parent.element) < 0)
//create a new node
parent.left = createNewNode(e);
else
//create a new node
parent.right = createNewNode(e);
}
//increment the size
size++;
//return statement
return true;
}
//definition of "createNewNode"
protected TreeNode<E> createNewNode(E e)
{
//return the statement
return new TreeNode<E>(e);
}
//definition of "inorder"
public void inorder()
{
//inorder traverse from the root
inorder(root);
}
//definition of inorder
protected void inorder(TreeNode<E> root)
{
//check the condition
if (root == null)
//return statement
return;
// inorder traversal from a subtree
inorder(root.left);
//display the element
System.out.print(root.element + " ");
// inorder traversal from a subtree
inorder(root.right);
}
// definition of "postoder"
public void postorder()
{
// postorder traversal from the root
postorder(root);
}
// definition of "postorder"
protected void postorder(TreeNode<E> root)
{
//check the condition
if (root == null)
//return statement
return;
//postorder traversal from a subtree
postorder(root.left);
postorder(root.right);
//display the element
System.out.print(root.element + " ");
}
//definition of "preorder"
public void preorder()
{
// preorder traversal from the root
preorder(root);
}
//definition of "preorder"
protected void preorder(TreeNode<E> root)
{
//check the condition
if (root == null)
//return statement
return;
//display the value
System.out.print(root.element + " ");
// preorder traversal from a subtree
preorder(root.left);
preorder(root.right);
}
//definition of "TreeNode" class
public class TreeNode<E extends Comparable<E>>
{
//declare the variables
E element;
TreeNode<E> left;
TreeNode<E> right;
//definition of constructor
public TreeNode(E e)
{
//set the value
element = e;
}
}
// definition of "getSize" method
public int getSize()
{
//return statement
return size;
}
// definition of "getRoot" method
public TreeNode getRoot()
{
//return statement
return root;
}
// definition of method
public java.util.ArrayList<TreeNode<E>> path(E e)
{
//create an object
java.util.ArrayList<TreeNode<E>> list = new java.util.ArrayList<TreeNode<E>>();
// start from the root
TreeNode<E> current = root;
//check the condition
while (current != null)
{
//add the node to the list
list.add(current);
//check the condition
if (e.compareTo(current.element) < 0)
{
//set the value
current = current.left;
}
//check the condition
else if (e.compareTo(current.element) > 0)
{
//set the value
current = current.right;
}
else
//break statement
break;
}
//return statement
return list;
}
//definition of "delete" method
public boolean delete(E e)
{
// declare the variables
TreeNode<E> parent = null;
TreeNode<E> current = root;
//check the condition
while (current != null)
{
//check the condition
if (e.compareTo(current.element) < 0)
{
//set the value
parent = current;
current = current.left;
}
//check the condition
else if (e.compareTo(current.element) > 0)
{
//set the value
parent = current;
current = current.right;
}
else
//break statement
break;
}
//check the condition
if (current == null)
return false;
//check the condition
if (current.left == null)
{
//check the condition
if (parent == null)
{
//set the value
root = current.right;
}
else
{
//check the condition
if (e.compareTo(parent.element) < 0)
//set the value
parent.left = current.right;
else
//set the value
parent.right = current.right;
}
}
else
{
//set the value
TreeNode<E> parentOfRightMost = current;
TreeNode<E> rightMost = current.left;
//check the condition
while (rightMost.right != null)
{
//set the value
parentOfRightMost = rightMost;
rightMost = rightMost.right;
}
//set the value
current.element = rightMost.element;
//check the condition
if (parentOfRightMost.right == rightMost)
//set the value
parentOfRightMost.right = rightMost.left;
else
//set the value
parentOfRightMost.left = rightMost.left;
}
//decrement the "size"
size--;
//return statement
return true;
}
//definition of "iterator"
public java.util.Iterator<E> iterator()
{
//return statement
return inorderIterator();
}
//definition of "inorderIterator"
public java.util.Iterator<E> inorderIterator()
{
//return statement
return new InorderIterator();
}
// definition of class "InorderIterator"
class InorderIterator implements java.util.Iterator
{
// store the elements in a list
private java.util.ArrayList<E> list = new java.util.ArrayList<E>();
//declare the variable
private int current = 0;
//constructor
public InorderIterator()
{
//call the method
inorder();
}
/*definition of inorder traversal from the root */
private void inorder()
{
//call the method
inorder(root);
}
/*definition of inorder traversal from a subtree */
private void inorder(TreeNode<E> root)
{
//check the condition
if (root == null)
//return statement
return;
//call the method
inorder(root.left);
//add the value to the list
list.add(root.element);
//call the method
inorder(root.right);
}
//definition of "hasNext"
public boolean hasNext()
{
//check the condition
if (current < list.size())
//return statement
return true;
//return statement
return false;
}
//definition of "next" method
public Object next()
{
//return statement
return list.get(current++);
}
// definition of "remove" method
public void remove()
{
//delete the current element
delete(list.get(current));
// clear the list
list.clear();
// rebuild the list
inorder();
}
}
// definition of "clear" method
public void clear()
{
//set the values
root = null;
size = 0;
}
}
//definition of interface
public interface Tree<E> extends java.util.Collection<E>
{
//declaration of methods
public boolean search(E e);
public boolean insert(E e);
public boolean delete(E e);
public int getSize();
// definition of default method
public default void inorder()
{
}
// definition of default method
public default void postorder()
{
}
// definition of default method
public default void preorder()
{
}
// definition of default method
public default boolean isEmpty()
{
//return statement
return size() == 0;
};
@Override
// definition of default method
public default boolean contains(Object e)
{
//return statement
return search((E)e);
}
@Override
// definition of default method
public default boolean add(E e)
{
//return statement
return insert(e);
}
@Override
// definition of default method
public default boolean remove(Object e)
{
//return statement
return delete((E)e);
}
@Override
// definition of default method
public default int size()
{
//return statement
return getSize();
}
@Override
// definition of default method
public default boolean containsAll(Collection<?> c)
{
//return statement
return false;
}
@Override
// definition of default method
public default boolean addAll(Collection<? extends E> c)
{
//return statement
return false;
}
@Override
// definition of default method
public default boolean removeAll(Collection<?> c)
{
//return statement
return false;
}
@Override
// definition of default method
public default boolean retainAll(Collection<?> c)
{
//return statement
return false;
}
@Override
// definition of default method
public default Object[] toArray()
{
//return statement
return null;
}
@Override
// definition of default method
public default <T> T[] toArray(T[] array)
{
//return statement
return null;
}
}
}
The height of tree is -1
Enter strings: aaa
bbb
ccc
ddd
eee
fff
The height of tree is 5
The height of tree is 5
aaa Green bbb ccc ddd eee fff
The breadth-first traversal is Tom George Jean Jane Kevin Jen Peter Kim Susan Michael Michelle
The height of tree1 is 7
The breadth-first traversal for tree2 is 50 45 59 35 48 51 58
The height of tree2 is 3
Want to see more full solutions like this?
Chapter 25 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- (Test perfect binary tree) JAVA A perfect binary tree is a complete binary tree with all levels fully filled. Define a new class named BSTWithTestPerfect that extends BST with the following methods: (Hint: The number of nodes in a perfect binary tree is 2^(height+1) - 1.) /** Returns true if the tree is a perfect binary tree */public boolean isPerfectBST() Use https://liveexample.pearsoncmg.com/test/Exercise25_03.txt to test your code. Class Name: Exercise25_03arrow_forward(No coding) Insert the following values into a binary search tree in the given order.(Draw the tree) Then traverse this tree using inorder, preorder ve postorder methods. List the values for each one. Then delete 15.(draw the resulting tree) 35,15,8,20,55,40,18,16,22arrow_forward24. Add the following new method in the BST class. /** Returns the number of nodes in this binary tree */ public int getNumberofNodes () Requirements: a. Don't use return size; b.write a recursive method that returns the number of nodes starting from www the root.arrow_forward
- Write a recursive method(Code ) static void mirrorTree(node root) This will take a tree as input and then change the tree such that it becomes a mirror of itself. We have the following methods implemented(you can use them and assume we coded somewhere else): getLeft(), getRight(), setLeft(), setRight(), getValue(). All the values in a node are integers. [Hint: Use them, and think how you would write when the tree only has 2 children ] Example:arrow_forwardA tree can be represented using lists as follows. (root listOfSubTrees ) tree listOfSubTrees tree = root listOfSubTrees = tree Consider the tree defined by: (FD (G (AHI) C) E B) Give the order of letters visited in when using a pre-order traversal implemented recursively as seen in class. (separate each element with a space where the left is the first element visited and the right is the last element visited, example: A B C D E F)arrow_forwardCourse : Data Structure and Algorithms Write a java/c++ code or an algorithm to solve the following problem. After that dry run and show output of algorithm using an example binary tree. . Write a recursive function to write the parent of the all the nodes while traversing. Like if you traverse the root node write the current node a, parent null. Then if you go to left sub tree, your code will show, current node : b , parent a and so on.arrow_forward
- How nodes are defined (struct node (value count left right) #:mutable #:transparent) Write in Racket (traverse n) A traversal of a BST is an algorithm for “visiting” all node in the BST. The traversal must visit each node exactly once. In the case of a linked list, a traversal is trivial since the structure is linear: start at the head, move to the next node, and stop when you reach the tail. In the case of a BST, traversal must account for multiple child nodes and keep track of which subtrees have already been visited and which have not. There are three types of traversal: in-order, pre-order, and post-order. We will only implement in-order. The in-order traversal of a BST has the property that the node values will display in ascending or sorted order. The function can be defined either recursively or iteratively. Recursion is much simpler, so we’ll stick to that. Recursive Algorithm for In-Order Traversal of BST parameter: node n, the root of the tree…arrow_forwardQuestion: Using Binary search tree write a Java program to Insert and print the element in (in-Order traversal ), then search the elements (a and z) in the tree. using the following tree:arrow_forwardC++ please Please complete Programming Exercise 4, from pages 1402, from chapter 19 from your textbook. Write a function, singleParent, that returns the number of nodes in abinary tree that have only one child. Add this function to the classbinaryTreeType and create a program to test this function. (Note: Firstcreate a binary search tree.)arrow_forward
- *Please using JAVA only* Objective Program 3: Binary Search Tree Program The primary objective of this program is to learn to implement binary search trees and to combine their functionalities with linked lists. Program Description In a multiplayer game, players' avatars are placed in a large game scene, and each avatar has its information in the game. Write a program to manage players' information in a multiplayer game using a Binary Search (BS) tree for a multiplayer game. A node in the BS tree represents each player. Each player should have an ID number, avatar name, and stamina level. The players will be arranged in the BS tree based on their ID numbers. If there is only one player in the game scene, it is represented by one node (root) in the tree. Once another player enters the game scene, a new node will be created and inserted in the BS tree based on the player ID number. Players during the gameplay will receive hits that reduce their stamina. If the players lose…arrow_forwardHelp on the following question A co-worker emails you and said she developed a recursive version for doing search in a binary search tree. Here’s the code for the function: public boolean searchRecursive(Node current, int searchValue) { if (current == null) return false; if (current.data == searchValue) return true; else if (current.data > searchValue) return searchRecursive(current.right, searchValue); else return searchRecursive(current.left, searchValue); } She’s not sure if there is an error or not because the code does compile. You analyze the code and respond to her as follows: Draw a picture of what a binary search tree would look like after inserting values of 10, 15, 18, 13, 5, 1, and 8 in that order Next, if you believe there is no error with the code, then show her how the code executes when searching for different values using the tree you made in step 1) Or, if you believe there is…arrow_forwardCan help in Java? Question: Using Binary search tree write a Java program to Insert and print the element in (in-Order traversal ), then search the elements (a and z) in the tree. using the following tree:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
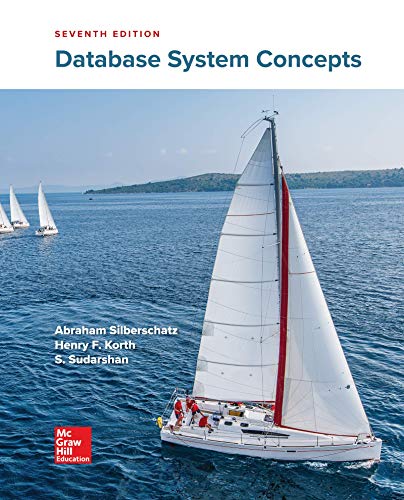
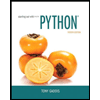
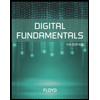
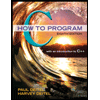
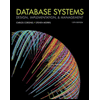
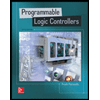