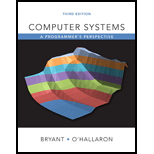
Explanation of Solution
Function definition for “replace_byte()” function:
The implementation for “replace_byte()” function is given below:
//Header file
#include <stdio.h>
#include <assert.h>
//Function definition for replace byte function
unsigned replace_byte(unsigned x, int i, unsigned char b)
{
//Check if value of "i" is less than "0", then
if (i < 0)
{
//Display error message
printf("error: value of i is negetive\n");
return x;
}
/* Check if value of "i" is greater than "sizeof(unsigned)-1", then */
if (i > sizeof(unsigned)-1)
{
//Display error message
printf("error: value of i is too high");
return x;
}
/* Assign the value of mask that is for byte
in general, 1 bye = 8 bits and then << implies *8 */
unsigned m = ((unsigned) 0xFF) << (i << 3);
//Defines the byte position
unsigned bytePosition = ((unsigned) b) << (i << 3);
//Return given value
return (x & ~m) | bytePosition;
}
//Main function
int main(int argc, char *argv[])
{
/* Call replace_byte function for replace byte 2 in given "x" by byte "0xAB" */
unsigned replaceByte2 = replace_byte(0x12345678, 2, 0xAB);
/* Call replace_byte function for replace byte 0 in given "x" by byte "0xAB" */
unsigned replaceByte0 = replace_byte(0x12345678, 0, 0xAB);
/* Check if the replaced byte equals to given value using "assert" function */
assert(replaceByte2 == 0x12AB5678);
assert(replaceByte0 == 0x123456AB);
return 0;
}
The given code is used to return an unsigned value in which byte “i” of argument “x” has been replaced by “b”...

Want to see the full answer?
Check out a sample textbook solution
Chapter 2 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- This exercise is to write a Decimal to Hex function using BYTE operations. You can use a List, Dictionary or Tuple for your Hex_Map as shown below, but it's not necessary to use one. It is necessary to use Byte operations to convert the decimal to hex. The bitwise operations are also available as __dunders__. Write a function to convert a integer to hexidecimal. Do not use the Python hex function (i.e. hexnumber = hex(number)) to convert the number, instead write your own unique version (i.e. MYHEX: hexnumber = MYHEX(number)). Your function should not use any Python functions like hex, dec, oct, bin or any other Python functions or modules. Functions, like Python ORD, can be used to convert a string to a integer. The input number range is 0 to 1024. def MYHEX(number): hex_map = {10: 'A', 11: 'B', 12: 'C', 13: 'D', 14: 'E', 15: 'F'} result = '' while number: digit = number % 16 if digit > 9: result = hex_map[digit] + result else:…arrow_forwardPlease write a function called “bin2dec” to convert a binary number to decimal,where the binary number is 8 bits and is stored as a word array in the memory, Most SignificantBit (MSB) at the lowest address. The starting address of the array is in $a0. The result should bereturned in $v0.For example, for the following code, $v0 should be 177 after the function call..dataAA: .word 1, 0, 1, 1, 0, 0, 0, 1.text.globl mainmain: la $a0, AAjal bin2decdone: li $v0,10syscallarrow_forwardQ6. Write a function that takes an unsigned integer andreturns the number of '1' bits it has(also known as the Hamming weight).For example, the 32-bit integer '11' has binaryrepresentation 00000000000000000000000000001011,so the function should return 3.T(n)- O(k) : k is the number of 1s present in binary representation.NOTE: this complexity is better than O(log n).e.g. for n = 00010100000000000000000000000000only 2 iterations are required.Number of loops isequal to the number of 1s in the binary representation."""def count_ones_recur(n): Do it.arrow_forward
- Consider the sequence C codes given below. Convert the C codes into equivalent MIPS code. Argument register $a0 and $a1 will be used for A [] and n and the return value should be placed in $v. Assume that check is a function that takes the argument and returns 0 or 1. You are allowed to use pseudocodes if necessary and you must use comment to describe your MIPS code. int tally (int A[], int n) { int i; int number = 0; for (i=0; iarrow_forwardSecuring data is very important. We are developing an encryption module for the communication system in C++. You are supposed to input data in a character array of size 100. After taking input in array pass this array to a function to encrypt. This function applies following encryption on data. For testing purposes, after encryption function is called, the main function should print the encrypted data in the array. Convert upper case letters to lower case and lower case letters to upper case. After conversion replace each alphabet with its next alphabet, for example “A” will be replaced by “B”, “B” will be replaced by “C”, and so on. Similarly “a” will be replaced by “b” etc. However, “Z” should be replaced by “A” and “z” should be replaced by “a”. Digits must be replaced by subtracting it from 9 for example 0 should be replaced by 9 (9-0=9), 1 should be replaced by 8 (9-1=8), 2 should be replaced by 7 (9-2=7)………… and 9 should be replaced by 0 (9-9=0). Spaces should be replaced by $…arrow_forwardWrite a C-function with two arguments (n and r) that has prototype: char clearbit(char k, char bits) The function clears (sets to 0) the bit number k (in the range of 0 to 7) in bits and returns the resulting value. For example, if k is 0x02 and bits is 0x07, the function would return bits with its k’th bit cleared, resulting in 0x03. It must not change other bits in bits. Hint: You may use any number of C-statements, but this task can be accomplished in as few as one!arrow_forward. Write a program in C to convert a binary number into a decimal number without using array, function and while loop. Test Data :Input a binary number :1010101Expected Output :The Binary Number : 1010101The equivalent Decimal Number : 85arrow_forwardDefine a function named get_encrypted_list (word) which takes a word as a parameter. The function returns a list of characters. The first element is the first letter from the parameter word and the rest is as a sequence of '*', each '*' representing a letter in the parameter word. Note: you can assume that the parameter word is not empty. For example: Test Result ['h', '*', **1 guess = get_encrypted_list('hello') print(guess) print (type (guess)) guess = get_encrypted_list('succeed') ['s', '*', print (guess) **¹, ¹*¹] **']arrow_forwardD. Implement a function that finds the last check digit using congruence; 3x₁ + 7x2 + 2x3 + x4 + 3x5 + x6 + 3x7 + xg + 5x9 + x10 + 3x11 + x12 = 0(mod10) given the first 11 digits stored in a string as input, eg: ¹99284792783'. (Do not use predefined functions or operators that perform this specific task) use "79357343104", "34553463334" and "66340534853" to test your function..arrow_forwardCAN YOU PLEASE RUN IT IN ONLINE GDB! C language. Write a program using pointers, which based on the adequate functions compute the sum, difference, dot product, or cross product of two vectors in Rn. Write a program using pointers, which based on the adequate functions, computes the sum, difference of multiplication of two matrices in Rm×n.arrow_forwardNeed help for SML programming lagauge. (Going from binary to decimal) I need a function called decimal (val decimal = fn: string -> int) that takes a bit string corresponding to an integer and returns the decimal value of that integer. For example, decimal "10001" returns 17, decimal "001101" returns 13. I've already done a decimal to binary though the function: fun binary(x)= if x<=1 then Int.toString(x) else binary(x div 2) ^ Int.toString( x mod 2);arrow_forwardWrite a C++ program that takes an char array of size twenty. Take input a sorted array from the user. Display the char array on console such that each row has only five elements separated by spaces. Then, you are required to then take a char x from the user and use binary search to search for element x in the array. Display the index of the element on the console. Also display two elements before and after the element x. Note: Don't use functions and strings.arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
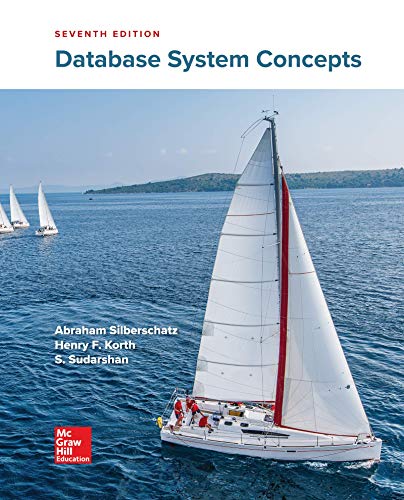
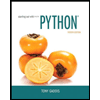
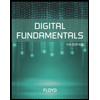
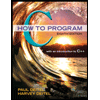
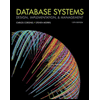
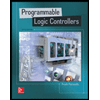