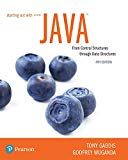
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
4th Edition
ISBN: 9780134787961
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 18, Problem 3PC
Program Plan Intro
EmployeeMap Class
Program plan:
- Define a class “Employee”.
- Declare the class members.
- Define the constructor.
- Initialize the variables
- Give a function definition “getId()”.
- Return “id” of the employee.
- Define a function definition “getName()”
- Return the “name” of the employee.
- Define a class “EmployeeMap”.
- Create an object of the “TreeMap”.
- Define a method “add()”.
- Add the “Employee” to the map.
- Define a method “lookup()”.
- Return the id form the “map”.
- Import the required header files.
- Define the main method.
- Create an object of the “Scanner”.
- Create an object of the “EmployeeMap” class.
- Add the employee list using “EmployeeMap” object.
- Iterate a while loop.
- Define a “try” block.
- Ask the user to enter the “id” of the employee.
- Using “lookUp()” method on a particular id, find the “employee name”.
- If the name is “null” throw an exception.
- Otherwise print the “name” of the employee with their “id”.
- Define a catch block to catch the exception.
- Print the message “enter a valid user id”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
When we use the Group-Object cmdlet, it will create this new property, which displays the number of objects in each grouping:
Group of answer choices
Number
Count
Entry
Name
The xxx_Student class:– Name - the name consists of the First and Last name separated by a space.– Student Id – a whole number automatically assigned in the student class– Student id numbers start at 100. The numbers are assigned using a static variable in the Student class• Include all instance variables• Getters and setters for instance variables• A static variable used to assign the student id starting at 100• A toString method which returns a String containing the student name and id in the format below:Student: John Jones ID: 101
The xxx_Course classA Course has the following information (modify your Course class):– A name– An Array of Students which contains an entry for each Student enrolled in the course (allow for up to 10 students)– An integer variable which indicates the number of students currently enrolled in the course.
Write the constructor below which does the following:Course (String name)Sets courseName to nameCreates the students array of size 10Sets number of…
Rectangle Object Monitoring
Create a Rectangle class that can compute the total area of all the created rectangle objects using static fields (variables). Remember that a Rectangle has two attributes: Length and Width. Implement the class by creating a computer program that will ask the user about three rectangle dimensions. The program should be able to display the total area of the three rectangle objects. For this exercise, you are required to apply all OOP concepts that you learned in class.
Sample output:
Enter Length R1: 1
Enter Width R1: 1
Enter Length R2: 2
Enter Width R2: 2
Enter Length R3: 3
Enter Width R3: 3
The total area of the rectangles is 14.00
Note: All characters in boldface are user inputs.
Chapter 18 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Ch. 18.1 - Prob. 18.1CPCh. 18.1 - What are the three general types of collections?Ch. 18.1 - Prob. 18.3CPCh. 18.1 - Prob. 18.4CPCh. 18.1 - Prob. 18.5CPCh. 18.1 - Prob. 18.6CPCh. 18.1 - Prob. 18.7CPCh. 18.2 - Prob. 18.8CPCh. 18.2 - Prob. 18.9CPCh. 18.2 - Prob. 18.10CP
Ch. 18.2 - Prob. 18.11CPCh. 18.2 - Prob. 18.12CPCh. 18.2 - Prob. 18.13CPCh. 18.2 - Prob. 18.14CPCh. 18.2 - Prob. 18.16CPCh. 18.2 - Prob. 18.17CPCh. 18.2 - Prob. 18.18CPCh. 18.2 - Prob. 18.20CPCh. 18.3 - Prob. 18.21CPCh. 18.3 - Prob. 18.22CPCh. 18.3 - Prob. 18.23CPCh. 18.3 - Prob. 18.24CPCh. 18.3 - Any time you override the Object classs equals...Ch. 18.3 - Prob. 18.26CPCh. 18.3 - Prob. 18.27CPCh. 18.3 - Prob. 18.28CPCh. 18.4 - Prob. 18.29CPCh. 18.4 - Prob. 18.31CPCh. 18.4 - Prob. 18.32CPCh. 18.6 - How do you define a stream of elements?Ch. 18.6 - How does a stream intermediate operation differ...Ch. 18.6 - Prob. 18.35CPCh. 18.6 - Prob. 18.36CPCh. 18.6 - Prob. 18.37CPCh. 18.6 - Prob. 18.38CPCh. 18.6 - Prob. 18.39CPCh. 18 - Prob. 1MCCh. 18 - Prob. 2MCCh. 18 - This type of collection is optimized for...Ch. 18 - Prob. 4MCCh. 18 - A terminal operation in a stream pipeline is also...Ch. 18 - Prob. 6MCCh. 18 - Prob. 7MCCh. 18 - This List Iterator method replaces an existing...Ch. 18 - Prob. 9MCCh. 18 - Prob. 10MCCh. 18 - This is an object that can compare two other...Ch. 18 - This class provides numerous static methods that...Ch. 18 - Prob. 13MCCh. 18 - Prob. 14MCCh. 18 - Prob. 15TFCh. 18 - Prob. 16TFCh. 18 - Prob. 17TFCh. 18 - Prob. 18TFCh. 18 - Prob. 19TFCh. 18 - Prob. 20TFCh. 18 - Prob. 21TFCh. 18 - Prob. 22TFCh. 18 - Prob. 1FTECh. 18 - Prob. 2FTECh. 18 - Prob. 3FTECh. 18 - Prob. 4FTECh. 18 - Write a statement that declares a List reference...Ch. 18 - Prob. 2AWCh. 18 - Assume that it references a newly created iterator...Ch. 18 - Prob. 4AWCh. 18 - Prob. 2SACh. 18 - Prob. 4SACh. 18 - Prob. 5SACh. 18 - Prob. 6SACh. 18 - How does the Java compiler process an enhanced for...Ch. 18 - Prob. 8SACh. 18 - Prob. 9SACh. 18 - Prob. 10SACh. 18 - Prob. 11SACh. 18 - Prob. 12SACh. 18 - Prob. 13SACh. 18 - Prob. 14SACh. 18 - Word Set Write an application that reads a line of...Ch. 18 - Prob. 3PCCh. 18 - Prob. 5PCCh. 18 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Appointment Class Requirements The appointment object shall have a required unique appointment ID string that cannot be longer than 10 characters. The appointment ID shall not be null and shall not be updatable. The appointment object shall have a required appointment Date field. The appointment Date field cannot be in the past. The appointment Date field shall not be null.Note: Use java.util.Date for the appointmentDate field and use before(new Date()) to check if the date is in the past. The appointment object shall have a required description String field that cannot be longer than 50 characters. The description field shall not be null. Appointment Service Requirements The appointment service shall be able to add appointments with a unique appointment ID. The appointment service shall be able to delete appointments per appointment ID.arrow_forwardCreate a BowlingTeam class The class has 2 fields: a field for the team name and an array that holds the team members’ names. Create get and set methods for the teamName field. Add a setMember method that sets a team member’s name. The method requires a position and a name, and it uses the position as a subscript to the members array. Add a getMember method that returns a team member’s name. The method requires a value used as a subscript that determines which member’s name to return. Create a BowlingTeamDemo class. In the main method include the following: Declare 2 variables: name and a constant NUM_TEAMS that holds 4 Bowling Team objects. Declare and instantiate an array teams of BowlingTeam objects. Using nested for loops, prompt the user to enter the 4 team names and enter the team members’ names. Using another nested for loop, output each team’s name and their team members’. Output should look like: Members of team The Lucky Strikes Carlos Diego Rose Lynn Members of team I…arrow_forwardPlease __Call the display method with car1 object.arrow_forward
- Design a GUI for Book view class for the following Library Information System, which you have worked on 1 with the following details: Library Item Class Design and TestingDesign a class that holds the Library Item Information, with item name, author, publisher. Write appropriate accessor and mutator methods. Also, write a tester program that creates three instances/objects of the Library Items class. Extending Library Item Class (Library and Book Classes): Extend the Library Item class in (1) with a Book class with data attributes for a book’s title, author, publisher and an additional attributes as number of pages, and a Boolean data attribute indicating whether there is both hard copy as well as eBook version of the book. Demonstrate Book Class in a Tester Program with an object of Book class.arrow_forwarddesign a Club class and use it to create an application that simulates asoccer league tournament. You are not limited to the features included in these instructionsDesign the Club class, to be used in your application, containing: A private data member code of type string that holds the three letters club’s abbreviation(ex, FCB, LIV, RAM ...) A private data member name of type string that holds the club’s name (ex, FC Barcelona,Liverpool, Real Madrid …) A private data member round of type integer that holds the number of rounds the club hasplayed so far in the current league. A private data member wins of type integer that holds the number of times the club haswon in the current league. A private data member draws of type integer that holds the number of times the club hasbeen drawn in the current league. A private data member losses of type integer that holds the number of times the club haslost in the current league. A private data member goals of type integer that holds the…arrow_forwardGradient FillIn this labwork are asked to write a GUI application again using AWT. This is a fairly easy labworkthat is more about getting used to synchronized online learning. You are expected to:• Draw two rectangles.• Both of them should be filled using GradientPaint() function of AWT. (Check out itsfunction definition that is listed below.)• The first gradient should be parallel to the diagonal of the first rectangle. The colorgradient should not be repeated (acyclic).• The second gradient should be horizontal. The color gradient should be repeated forthis one (cyclic).• You are free to choose the colors but other than that your output should be similar tothe example screenshot given below.arrow_forward
- Create a MagicalPotionShop class, this class needs a field called potions. The potions field should be an ArrayList of the type Potion. Create a MagicalPotion class, this class needs two fields one called name, the other called effect. Both of the fields should be Strings.arrow_forwardStatic & Not Final Field: Accessed by every object, Changing Non-Static & Final Field: Accessed by object itself, Non-Changing Static & Final: Accessed by every object, Non-Changing Non-Static & Not Final Field: Accessed by object itself, ChangingRead the following situation and decide how the variables should be defined. You have a class named HeartsPlayerA round of Hearts starts with every player having 13 cardsPlayers then choose 3 cards to “trade” with a player (1st you pass left, 2nd you pass right, 3rd you pass across, 4th you keep)Players then strategically play cards in order to have the lowest scoreAt the end of the round, points are cumulatively totaled for each player.If one player’s total is greater than 100, the game ends and the player with the lowest score wins. 1. How should the following data fields be defined (with respect to final and static)?(a) playerPosition (These have values of North, South, East, or West)(b) directionOfPassing(c) totalScore…arrow_forwardA field has access that is somewhere between public and private. static final Opackage Oprotectedarrow_forward
- Car Class Project The car classwill havethe following attributes: •year: an integer that holds the car's model year •model: a string that holds the make of the car •make: a string that holds the model of the car •speed: an integer that holds the car's current speed Your class should contain thefollowing: A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class. •A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string. •To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly. •An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed(). •A modifier method called accelerate() which adds 5 to the speed variable…arrow_forwardCreate the StockTask1 Java project Create a Stock object class that contains the following information: The symbol of the stock is stored in a private string data field called symbol. The stock's name is stored in a private string data field called name. previousClosingPrice is a private double data area that stores the stock price for the previous day. currentPrice is a private double data area that stores the stock price at the current time. A constructor for creating a stock with the given symbol and name. For the data fields, there are accessor methods (get-methods). For the data fields, there is a mutator method (set-methods). A method named getChangePercent()that returns the percentage changed from previousClosingPrice to currentPrice. Formula:perc_change = (current price - previous closing price) / previous closing price x 100 Create a test class named testStockthat creates a Stockobject with the stock symbol SUNW, the name Sun Microsystems Inc., and the previous closing…arrow_forwardCar Class Project The car classwill havethe following attributes: •year: an integer that holds the car's model year •model: a string that holds the make of the car •make: a string that holds the model of the car •speed: an integer that holds the car's current speed Your class should contain thefollowing: A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class. •A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string. •To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly. •An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed(). •A modifier method called accelerate() which adds 5 to the speed variable…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
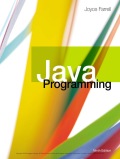
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
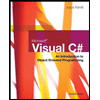
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
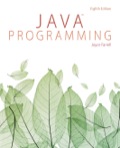
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT