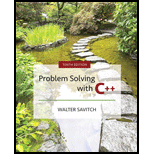
Explanation of Solution
Program:
File name: sale.h
//include libraries
#ifndef SALE_H
#define SALE_H
#include <iostream>
using namespace std;
//using the namespace
namespace salesavitch
{
//create a class
class Sale
{
//define access specifier
public:
//declare the constructors
Sale();
Sale(double thePrice);
//define required methods
double bill() const;
double savings(const Sale& other) const;
//define access specifier
protected:
//declare required variables
double price;
};
//define an overloaded method
bool operator <(const Sale& first, const Sale&
second);
}
#endif // SALE_H
File name: discount.h
//include libraries
#ifndef DISCOUNTSALE_H
#define DISCOUNTSALE_H
#include "sale.h"
//using the namespace
namespace salesavitch
{
//create a class
class DiscountSale : public Sale
{
//define access specifier
public:
//declare the constructors
DiscountSale();
DiscountSale(double the_price, double the_discount);
//Discount is expressed as a percent of the price.
virtual double bill() const;
//define access specifier
protected:
//declare required variable
double discount;
};
}
#endif //DISCOUNTSALE_H
File name: sale.cpp
//include libraries
#include "sale.h"
//using the namespace
namespace salesavitch
{
//define a constructor
Sale::Sale() : price(0)
{}
//define a constructor
Sale::Sale(double the_price) : price(the_price)
{}
//declare a method
double Sale::bill() const
{
//return statement
return price;
}
//declare a method
double Sale::savings(const Sale& other) const
{
//return statement
return ( bill() - other...

Trending nowThis is a popular solution!

Chapter 15 Solutions
Problem Solving with C++ (10th Edition)
- First, you need to design, code in Java, test and document a base class, Student. The Student class willhave the following information, and all of these should be defined as Private:A. Title of the student (eg Mr, Miss, Ms, Mrs etc)B. A first name (given name)C. A last name (family name/surname)D. Student number (ID) – an integer number (of type long)E. A date of birth (in day/month/year format – three ints) - (Do NOT use the Date class fromJAVA)The student class will have at least the following constructors and methods:(i) two constructors - one without any parameters (the default constructor), and one withparameters to give initial values to all the instance variables.(ii) only necessary set and get methods for a valid class design.(iii) method to write the output of all the instance variables in the class to the screen (which willbe overridden in the respective child classes, as you have more instance variables thatrequired to be output).(iv) an equals method which compares two…arrow_forwardCreate a base class called Insect. All insects belong to an Order [i.e. “Hemiptera” (ants), “Siphonaptera” (fleas), “Termitoidae” (termites), “Gryllidae” (crickets), etc.] and have a size that is measured in millimeters (use a double data type). Provide a default constructor that initializes the size to zero and outputs the message “Invoking the default Insect constructor” and another constructor that allows the Order and size to be set by the client. This other constructor should also output the message “Invoking the 2-argument Insect constructor.” Also create a destructor for this class that outputs the message “Invoking the default Insect destructor.” Your Insect class should have a function called Eat that cannot be implemented. That is, it should be declared as a purely virtual function. Your class should also have Get and Set methods to allow the order and size to be accessed.arrow_forwardFirst, you need to design, code in Java, test and document a base class, Student. The Student class will have the following information: A. Title of the student (eg Mr, Miss, Ms, Mrs etc)B. A first name (given name)C. A last name (family name/surname)D. Student number (ID) – an integer number (of type long)E. A date of birth (in day/month/year format – three ints) - (Do NOT use the Date class from JAVA)The student class will have at least the following constructors and methods:(i) two constructors - one without any parameters (the default constructor), and one with parameters to give initial values to all the instance variables.(ii) a reasonable number of set and get methods.(iii) methods to compute the final overall mark and the final grade (which will be overridden in the respective child classes). These two methods will be void methods that set the appropriate instance variables. Remember one method can call another method. If you prefer, you can define a single method that sets…arrow_forward
- Help, I making a elevator simulator, I need help in writing code in the Elevator class and it's polymorphism class. I am not sure what to do next. Any help is appreciated. There are 4 types of passengers in the system:Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements.VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators.Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators.Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. There are 4 types of elevators in the system:StandardElevator: This is the most common type of elevator and has a request…arrow_forwardSuppose you are a backend developer for a tech news platform. You have been asked to design a templating system for their news articles. To do this, you will need to run some proof of concepts. Define the TabletComputer class in the main.py file so that the code in Snippet 7.19 runs without error: # Write your TabletComputer class here uPad = TabletComputer(12.9, "1TB", "uPadOS 13.5.1")rootProX = TabletComputer(13.0, "512GB", "Glass 10 Home") print(f"The new uPad has a {uPad.screen_size}" f" inch display. A maximum {uPad.storage} of storage and runs on" f" the latest version of {uPad.os}. Its biggest competitor is" f" the Root Pro X which holds a similar {rootProX.screen_size}" f" inch display, with a maximum storage capacity of {rootProX.storage} and runs {rootProX.os}." ) Define the __init__ function within the TabletComputer class with the necessary parameters to set up the member variables. You will need to set the following member variables: screen_size…arrow_forwardFirst, you need to design, code in Java, test and document a base class, Student. The Student class willhave the following information, and all of these should be defined as Private:A. Title of the student (eg Mr, Miss, Ms, Mrs etc)B. A first name (given name)C. A last name (family name/surname)D. Student number (ID) – an integer number (of type long)E. A date of birth (in day/month/year format – three ints) - (Do NOT use the Date class fromJAVA)The student class will have at least the following constructors and methods:(i) two constructors - one without any parameters (the default constructor), and one withparameters to give initial values to all the instance variables.(ii) only necessary set and get methods for a valid class design.(iii) method to write the output of all the instance variables in the class to the screen (which willbe overridden in the respective child classes, as you have more instance variables thatrequired to be output).(iv) an equals method which compares two…arrow_forward
- Consider writing a program to manage a collection of movies. There are three kinds of movies in the collection: dramas, comedies, and documentaries. The collector would like to keep track of each movie's title, the name of its director, and the year the movie was made. Some operations are to be implemented for all movies, and there will also be special operations for each of the three different kinds of movies. How would you design the class(es) to represent this system of movies? Your design should implement the concept of Overridingarrow_forwardWrite a Java application for the Banking system that consists of at least three classes for Bank, Clients and Transactions. The following concepts should be applied: Defining a constructor with and without arguments. Use the setter, getter and toString methods. Sending an object/s to a method and returning it as a return value. Defining array of objects and using loop/s and Scanner object/s for data entry. Defining the main method that integrates the whole project.arrow_forwardI need help with: The following is a class definition for a simple Ebook. Two instance variables and one parameterless constructor are provided. Part 1: Write a second Java constructor that takes a String array of pages as input and sets the String array instance variable equal to the input. Continue to default the page number to zero. Part 2: Write a getter and a setter Java method for the current page number variable. The setter should check to make sure that the input is a valid page number and only update the variable if the new value is valid. Part 3: Write a getCurrentPage method that returns the String of the current page indexed by current_page. public class Ebook { private String[] pages; private int current_page; //constructor public Ebook() { this.pages = {"See Spot.", "See Spot run.", "Run, Spot, run."}; this.current_page = 0; } }arrow_forward
- please may you : create a Python class named Animal with properties for name, age, and a method called speak. also include an initializer method for the class and demonstrate how to create an instance of this class. and extend the Animal class by adding a decorator for one of its properties (e.g., age). please include both a getter and a setter for the property, and demonstrate error handling.arrow_forwardSuppose you compile the class YourClass. What will be the name of thefile containing the resulting bytecode?arrow_forwardIn C++Make sure to post unique assignment. Do not copy/paste what has already being posted before. I give thumbs up and thumbs down based on what you post. Write a definition of a class that has the following properties. The name of the class is secretType. (Private) - The class secretType has four member variable: name (a string), age and weight (int), height (double) (Public) - The class secretType has the following member functions: (Make the accessor functions const) print – outputs the data in the member variables in a nice format constructor – that sets the name, age, weight and height getName – value returning function returns the name getAge – value returning function returns the height getWeight – value returning function returns the weight weightStatus – value returning function that returns a string according to the following chart. The formula for BMI (body mass index) - Body Mass Index is a simple calculation using a person’s height and weight. The formula is BMI = kg/m2…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
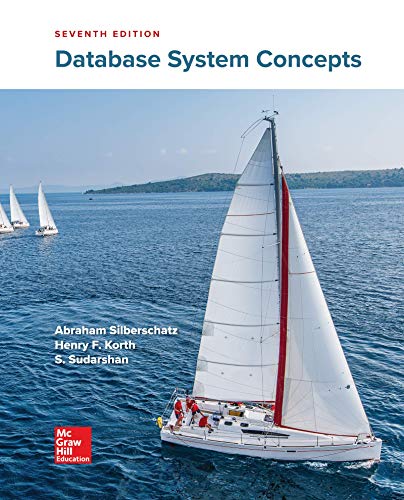
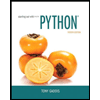
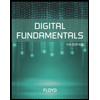
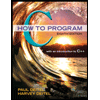
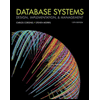
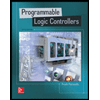