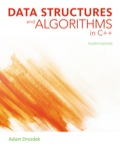
Concept explainers
String functions:
The string functions and their purpose is shown below:
- The function “strcpy(s1,s2)” copies string “s2” into “s1”.
- The function “strcat(s1,s2)” concatenates string “s2” on end of “s1”.
- The function “strlen(s1)” returns length of “s1”.
- The function “strchr(s1,ch)” would return a pointer to first presence of character “ch” in string “s1” .
Explanation of Solution
//(b)strcmp function
The function “strcmp()” compares two strings. It takes header pointer of two strings as function arguments, it checks until the string reaches null and compares both strings by comparing each character at corresonding position ...
Explanation of Solution
//(c) Strcat function
The function “Strcat()” copies data of “s2” to “s1”. To do so, it first reaches end of the string “s1” using recursive calls “Strcat(++s1, s2)”...
Explanation of Solution
//(d) Strchr function
The function “Strchr()” searches for a particular character in string. It iterates through character array and compares each character with search character, if it matches then the index of match is returned.
char* Strchr(char *s, char ch)
{
/*It checks for each character in string and compares it with that of the search string until the character array reaches null or it becomes empty. If a match is obtained, then store index of match*/
for ( ; *s != ch && *s != '\0'; s++);
//Return index of matched character
return *s == ch ? s : 0;
}
The main function defines two character array and tests the functions “Strlen()”, “Strcmp()”, “Strchr()”, “Strcat()” and displays the final result based on the return values of each functions
int main()
{
//Declare the variable
int ret;
//Declare character arrays
char str1[100] = "Drowning";
char *str2 = "Boat";
//Declare pointer of string
char *pch;
//Declare variables
int length ;
//Call the function "strlen()" and store the return value
length = Strlen(str1);
//Display first string
cout<<"String1: ";
puts(str1);
//Display second string
cout<<"String2: ";
puts(str2);
//Display the length of first string
cout<<"\nLength of String1 :"<< length;
//Call the function "Strcmp()" and store the return value of function
ret = Strcmp(str1,str2);
If return value is less than 0, then “String1” is less than “String2”. If return value is greater than 0, then “String2” is less than “String1”, else “String1” equals “String2”.
if(ret < 0)
{
//Display the result
cout<<"\nString1 is less than String2";
}
If return value is greater than 0, then “String2” is less than “String1”
else if(ret > 0)
{
//Display the result
cout<<"\nString2 is less than String1";
}
If “String1” equals “String2”, display the equal message
//"String1" equals "String2"
else
{
//Display the result
cout<<"\n String1 equals String2";
}
The function “Strchr()” is called with “str1” and search character “r” as argument, function’ sreturn value is stored, that is , the value of matched index...

Want to see the full answer?
Check out a sample textbook solution
Chapter 1 Solutions
EBK DATA STRUCTURES AND ALGORITHMS IN C
- In C Programming: Write a function inputAllCourses() which receives an array of course pointers and the array’s size, then allows the user to input all courses in the array by calling inputCourse()arrow_forwardWrite a recursive function to return the number of uppercase letters in a string using the following function headers:def countUppercase(s):def countUppercaseHelper(s, high): Write a test program that prompts the user to enter a string and displays the number of uppercase letters in the string.arrow_forwardpointers as Arguments:In the C programming language there is no pass-by-reference syntax to passa variable by reference to a function. Instead a variable is passed by pointer(just to be confusing, sometimes passing by pointer is referred to as pass byreference). This Practice Program asks you to do the same thing as C.Here is the header for a function that takes as input a pointer to an integer:1. void addOne (int ∗ptrNum )Complete the function so it adds one to the integer referenced by ptrNum.Write a main function where an integer variable is defined, give it an initialvalue, call addOne, and output the variable. It should be incremented by 1.arrow_forward
- In C++ Write a recursive function that displays a string reversely on the console using the following header:void reverseDisplay(const string& s) For example, reverseDisplay("abcd") displays dcba. Write a test programthat prompts the user to enter a string and displays its reversal.arrow_forwardWrite a program that converts all lowercase characters in a given string to its equivalent uppercase character. [Use pointers]arrow_forwardWrite a recursive function that converts a decimal number into a binary number as a string. The function header is: string decimalToBinary(int value) Write a test program that prompts the user to enter a decimal number and displays its binary equivalent.arrow_forward
- Data Structures the hasBalancedParentheses () method. Note: this can be done both iteratively or recursively, but I believe most people will find the iterative version much easier. C++: We consider the empty string to have balanced parentheses, as there is no imbalance. Your program should accept as input a single string containing only the characters ) and (, and output a single line stating true or false. The functionality for reading and printing answers is written in the file parentheses.checker.cpp; your task is to complete the has balanced.parentheses () function. **Hint: There's a pattern for how to determine if parentheses are balanced. Try to see if you can find that pattern first before coding this method up.arrow_forwardWrite a function namedisInt that hasone parm, a stringand returnsanint. The function should return true (in the C sense) if the string contains an int (all characters in the string are digits) and return false (in the C sense) otherwise. USE POINTER NOTATION INSTEAD OF ARRAY NOTATION. NO BRACKETS.arrow_forwardWrite a recursive function that displays a string reversely on the console using the following header: void reverseDisplay(const string& s) For example, reverseDisplay("abcd") displays dcba. Write a test program that prompts the user to enter a string and displays its reversal.arrow_forward
- Write a recursive function that displays a string reversely on the console using the following header: def reverseDisplay(value):For example, reverseDisplay("abcd") displays dcba. Write a test programthat prompts the user to enter a string and displays its reversal.arrow_forwardCreate python programs for the following: Create a programmers defined function that will do the same as strlen function. Create a program that will return a reverse string using pointer.arrow_forwardHelp with a lisp function (please post photos) A lisp function that generates a random day of the week, then displays a message saying that "Today is ... and tomorrow will be ...". For this purpose, create a global variable before the function containing the days of the week as a list. Then use the built-in function random first to generate a number between 0 and 6 (including). The expression (random) by itself generates a random integer. You can call it with one parameter to return a value within the range from 0 to the value of the parameter-1. For example, (random 10) will return a value between 0 and 9. Next, use the number generated at the previous step to retrieve the symbol for the day of the week from the list. Use the built-in elt function. We want the name of the day to appear capitalized. For this, extract the symbol-name of the day first, then apply the built-in function capitalize to it. Use the result in the princ function call, and do the same thing for the next day.…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
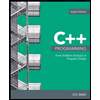