Use the Stack interface to implement the LinkedStack.java class. Stack.java public interface Stack { /** * Push an element onto the stack. * * @param element, a value to be pushed on the stack */ public void push(E element); /** * Pop the top element off the stack. */ public E pop(); /** * Return the top element on the stack. */ public E top(); /** * Return True if the stack contains no elements. * * @return true if there are no elements in the stack */ public boolean isEmpty(); } ***In LinkedStack.java, change only The methods with empty bodies Do not use a sentinel node. In this implementation you use an inner class That is, a class that is declared inside of another class. The Node class isn't needed by any other class, so it is declared as a private class inside the LinkedStack class. The Node class has two fields: element and next. Things to note in the class LinkedStack: The EmptyStackExceptionis being used, same as in the ArrayStack. The class StringJoineris imported for use in the toString() LinkedStack.java import java.util.EmptyStackException; import java.util.StringJoiner; public class LinkedStack implements Stack { private class Node { public E element; public Node next; public Node(E element) { this(element, null); } public Node(E element, Node next) { this.element = element; this.next = next; } } private Node top; /** * Constructor for objects of class LinkedStack */ public LinkedStack() { } @Override public void push(E element) { // Complete this method } @Override public E pop() { // Complete this method } @Override public E top() { // Complete this method } @Override public boolean isEmpty() { // Complete this method } @Override public int size() { // Complete this method } @Override public String toString() { StringJoiner joiner = new StringJoiner(", ", "[", "]"); for (Node current = top; current != null; current = current.next) { joiner.add(current.element.toString()); } return joiner.toString
**Use the Stack interface to implement the LinkedStack.java class.
Stack.java
public interface Stack<E> {
/**
* Push an element onto the stack.
*
* @param element, a value to be pushed on the stack
*/
public void push(E element);
/**
* Pop the top element off the stack.
*/
public E pop();
/**
* Return the top element on the stack.
*/
public E top();
/**
* Return True if the stack contains no elements.
*
* @return true if there are no elements in the stack
*/
public boolean isEmpty();
}
***In LinkedStack.java, change only The methods with empty bodies Do not use a sentinel node. In this implementation you use an inner class That is, a class that is declared inside of another class. The Node class isn't needed by any other class, so it is declared as a private class inside the LinkedStack class. The Node class has two fields: element and next. Things to note in the class LinkedStack: The EmptyStackExceptionis being used, same as in the ArrayStack. The class StringJoineris imported for use in the toString()
LinkedStack.java
import java.util.EmptyStackException;
import java.util.StringJoiner;
public class LinkedStack<E> implements Stack<E> {
private class Node {
public E element;
public Node next;
public Node(E element) {
this(element, null);
}
public Node(E element, Node next) {
this.element = element;
this.next = next;
}
}
private Node top;
/**
* Constructor for objects of class LinkedStack
*/
public LinkedStack() {
}
@Override
public void push(E element) {
// Complete this method
}
@Override
public E pop() {
// Complete this method
}
@Override
public E top() {
// Complete this method
}
@Override
public boolean isEmpty() {
// Complete this method
}
@Override
public int size() {
// Complete this method
}
@Override
public String toString() {
StringJoiner joiner = new StringJoiner(", ", "[", "]");
for (Node current = top; current != null; current = current.next) {
joiner.add(current.element.toString());
}
return joiner.toString();
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

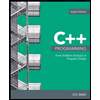
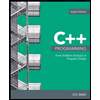