Write a class named Homework in IntelliJ that implements: (1) A function that performs dot product between two vectors a and b: public static double dotProduct(double[] a, double[] b) (2) A function that performs matrix multiplication between aMat and bMat and returns the result in cMat public static void matMultiply(double[][] aMat, double[][] bMat, double[][] cMat) (3) A function that takes a line (String) as input and breaks it up into words that may be separated by space, comma or tab: public static String[] getLineWords(String line) (4) A main function that tests all the above functions and prints out the results. Code written (has errors, but this is the structure/ method I'm going for) public class Homework { public static double dotProduct(double[] a, double[] b) { double result = 0; for (int i = 0; i < a.length; i++) { result += a[i] * b[i]; } return result; } public static void matMultiply(double[][] aMat, double[][] bMat, double[][] cMat) { } public static void printMat(double[][] mat) { // needed in code } public static String[] getLineWords(String line) { String[] words = line.split("[, \t]+"); return words; } public static void main(String[] args) { double[] a = {1, 2, 3}; double[] b = {4, 5, 6}; double dotProductResult = dotProduct(a, b); System.out.println("Dot product: " + dotProductResult); //matMultiply //Allocate memory for aMat, bMat, cMat Double[][] aMat = newdouble[3][3]; Homework.matMultiply(aMat, bMat, cMat); Homework.printMat(cMat); //needed in code //homework.matMultiply(); double result = 1; result = result - 1; //result--; }
Please send me answer with in 10 min!! I will you rate you good for sure!! Please solve all the 4 parts!!
Write a class named Homework in IntelliJ that implements:
(1) A function that performs dot product between two
public static double dotProduct(double[] a, double[] b)
(2) A function that performs matrix multiplication between aMat and bMat and returns the
result in cMat
public static void matMultiply(double[][] aMat, double[][] bMat, double[][] cMat)
(3) A function that takes a line (String) as input and breaks it up into words that may be
separated by space, comma or tab:
public static String[] getLineWords(String line)
(4) A main function that tests all the above functions and prints out the results.
Code written (has errors, but this is the structure/ method I'm going for)
public class Homework { public static double dotProduct(double[] a, double[] b) { double result = 0; for (int i = 0; i < a.length; i++) { result += a[i] * b[i]; } return result; } public static void matMultiply(double[][] aMat, double[][] bMat, double[][] cMat) {
}
public static void printMat(double[][] mat) { // needed in code
}
public static String[] getLineWords(String line) {
String[] words = line.split("[, \t]+"); return words;
}
public static void main(String[] args) {
double[] a = {1, 2, 3};
double[] b = {4, 5, 6};
double dotProductResult = dotProduct(a, b);
System.out.println("Dot product: " + dotProductResult);
//matMultiply
//Allocate memory for aMat, bMat, cMat
Double[][] aMat = newdouble[3][3];
Homework.matMultiply(aMat, bMat, cMat);
Homework.printMat(cMat); //needed in code
//homework.matMultiply();
double result = 1;
result = result - 1; //result--;
}

Step by step
Solved in 4 steps with 3 images

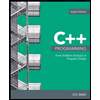
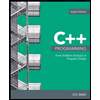