PYTHON PROGRAMMING ONLY NEED HELP MAKING A FLOWCHART TO CORRESPOND WITH CODE CODE IS ALREADY DONE AND CORRECT FLOWCHART EXAMPLE PROVIDED BELOW My code: def calculate_property_tax(actual_value): # Calculate the assessment value (60% of the actual value) assessment_value = actual_value * 0.6 # Calculate the property tax (72¢ for every $100 of assessment value) property_tax = (assessment_value / 100) * 0.72 return assessment_value, property_tax def main(): while True: try: actual_value = float(input("Enter the actual value of the property: $")) if actual_value < 0: print("Please enter a non-negative value.") else: break except ValueError: print("Invalid input. Please enter a valid number.") assessment_value, property_tax = calculate_property_tax(actual_value) print(f"Assessment Value: ${assessment_value:.2f}") print(f"Property Tax: ${property_tax:.2f}") if __name__ == "__main__": main()
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
PYTHON
NEED HELP MAKING A FLOWCHART TO CORRESPOND WITH CODE
CODE IS ALREADY DONE AND CORRECT
FLOWCHART EXAMPLE PROVIDED BELOW
My code:
def calculate_property_tax(actual_value):
# Calculate the assessment value (60% of the actual value)
assessment_value = actual_value * 0.6
# Calculate the property tax (72¢ for every $100 of assessment value)
property_tax = (assessment_value / 100) * 0.72
return assessment_value, property_tax
def main():
while True:
try:
actual_value = float(input("Enter the actual value of the property: $"))
if actual_value < 0:
print("Please enter a non-negative value.")
else:
break
except ValueError:
print("Invalid input. Please enter a valid number.")
assessment_value, property_tax = calculate_property_tax(actual_value)
print(f"Assessment Value: ${assessment_value:.2f}")
print(f"Property Tax: ${property_tax:.2f}")
if __name__ == "__main__":
main()



Step by step
Solved in 3 steps with 2 images

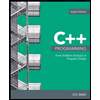
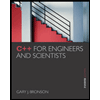
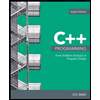
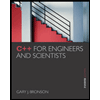