Checkpoint B If this population plays (and loses) the lottery 2 times: It could become [0,3,4,5,6], if the first individual played twice Or it could become [2,3,4,4,5], if the last two individuals each played once etc Continuing the example, scholarships might then award a total of $3 of awards to the population in the form of $1 scholarships. If the wealth had originally been [0,3,4,5,6], then: It could become[3,3,4,5,6], if the 1st individual got all three awards Or it could become [0,4,5,5,7], if it was distributed equally among the 2nd, 3rd, and 5th individuals etc We assume the lottery system is backed by a relatively huge pool of capital, so that scholarships are awarded no matter how many lottery winners there are. We also assume who plays the lottery and who benefits from scholarships will be random, at the individual-level. Later, at the population-level, we will select behaviors for our simulation based on social science research. The function generate_disparity_msg() returns a string summarizing the distribution of wealth. Here are examples of that analysis: highIncomeList lowIncomeList Wealth Distribution [2,3,4,5,6] [6,5,4,3,2] High income: 50% of wealth Low income: 50% of wealth [5, 6, 10, 14] [1, 5, 7, 2] High income: 70% of wealth Low income: 30% of wealth [4, 10, 2, 5, 8] [2, 7] High income: 76% of wealth Low income: 24% of wealth Implementation Strategy Implement each function from the template following the description in their docstring: sim_lottery() award_scholarship() generate_disparity_msg() For the messages returned by generate_disparity_msg(), adapt this fstring for your code: msg = f"Decade {decade}: High income group " +\ f"has {highIncomePercent:.0f}% of the community's wealth. "+\ f"Low income group has {lowIncomePercent:.0f}% "+\ f"of the community's wealth." This is template for check point B: def sim_lottery(incomeList, numPlays): """Uses play_lottery_once() to simulate the lottery for some list of indivudals from an income group. Within that group, the total number of lottery plays are numPlays (of course, some individuals might play multiple times). For each lottery ticket, the simulation picks a random individual from the incomeList and adds the game reward to that individual's wealth. Parameters: incomeList (list): wealth values for the given income group numPlayers (int): the number of players who will play the lottery Returns: None """ for i in range(numPlays): # YOUR CODE HERE pass def award_scholarship(incomeList, awardTotal): """Redistributes funds from the lottery in the form of a scholarship. Select a random recipient from the income group list. Each recipient receives $1 added to their indivual wealth. Parameters: incomeList (list): indivual wealth values for the income group awardTotal (int): total amount of lottery funds to be rewarded to members of this income group Returns: None """ for i in range(awardTotal): # YOUR CODE HERE pass def generate_disparity_msg(highIncomeList, lowIncomeList, decade): """Generates a string that describes the percentages of wealth possessed by the higher income half and lower income half for any given year. Parameters: highIncomeList (list): list of weath of individuals lowIncomeList (list): list of weath of individuals decade (int): a number indicating the decade Returns: str: The string with a message about the wealth disparity """ pass if __name__ == "__main__": seed = int(input('Enter a seed for the simulation: ')) random.seed(seed) # data about each population's wealth low_income_group = [50,50,50,50,50] high_income_group = [100,100,100,100,100] # each group plays the lottery, some amount of times sim_lottery(low_income_group, 3) sim_lottery(high_income_group, 1) # each group is awarded scholarships, in some amount award_scholarship(high_income_group, 5) award_scholarship(low_income_group, 2) m = generate_disparity_msg(high_income_group, low_income_group, 1) print(m)
Checkpoint B
If this population plays (and loses) the lottery 2 times:
- It could become [0,3,4,5,6], if the first individual played twice
- Or it could become [2,3,4,4,5], if the last two individuals each played once
- etc
Continuing the example, scholarships might then award a total of $3 of awards to the population in the form of $1 scholarships. If the wealth had originally been [0,3,4,5,6], then:
- It could become[3,3,4,5,6], if the 1st individual got all three awards
- Or it could become [0,4,5,5,7], if it was distributed equally among the 2nd, 3rd, and 5th individuals
- etc
We assume the lottery system is backed by a relatively huge pool of capital, so that scholarships are awarded no matter how many lottery winners there are. We also assume who plays the lottery and who benefits from scholarships will be random, at the individual-level. Later, at the population-level, we will select behaviors for our simulation based on social science research.
The function generate_disparity_msg() returns a string summarizing the distribution of wealth. Here are examples of that analysis:
highIncomeList | lowIncomeList | Wealth Distribution |
---|---|---|
[2,3,4,5,6] | [6,5,4,3,2] | High income: 50% of wealth Low income: 50% of wealth |
[5, 6, 10, 14] | [1, 5, 7, 2] | High income: 70% of wealth Low income: 30% of wealth |
[4, 10, 2, 5, 8] | [2, 7] | High income: 76% of wealth Low income: 24% of wealth |
Implementation Strategy
Implement each function from the template following the description in their docstring:
- sim_lottery()
- award_scholarship()
- generate_disparity_msg()
For the messages returned by generate_disparity_msg(), adapt this fstring for your code:
msg = f"Decade {decade}: High income group " +\ f"has {highIncomePercent:.0f}% of the community's wealth. "+\ f"Low income group has {lowIncomePercent:.0f}% "+\ f"of the community's wealth."
This is template for check point B:
def sim_lottery(incomeList, numPlays):
"""Uses play_lottery_once() to simulate the lottery for some list of
indivudals from an income group. Within that group, the total
number of lottery plays are numPlays (of course, some individuals
might play multiple times). For each lottery ticket, the simulation
picks a random individual from the incomeList and adds the game
reward to that individual's wealth.
Parameters:
incomeList (list): wealth values for the given income group
numPlayers (int): the number of players who will play the lottery
Returns: None
"""
for i in range(numPlays):
# YOUR CODE HERE
pass
def award_scholarship(incomeList, awardTotal):
"""Redistributes funds from the lottery in the form of a scholarship.
Select a random recipient from the income group list. Each recipient
receives $1 added to their indivual wealth.
Parameters:
incomeList (list): indivual wealth values for the income group
awardTotal (int): total amount of lottery funds to be rewarded
to members of this income group
Returns: None
"""
for i in range(awardTotal):
# YOUR CODE HERE
pass
def generate_disparity_msg(highIncomeList, lowIncomeList, decade):
"""Generates a string that describes the percentages of wealth possessed
by the higher income half and lower income half for any given year.
Parameters:
highIncomeList (list): list of weath of individuals
lowIncomeList (list): list of weath of individuals
decade (int): a number indicating the decade
Returns:
str: The string with a message about the wealth disparity
"""
pass
if __name__ == "__main__":
seed = int(input('Enter a seed for the simulation: '))
random.seed(seed)
# data about each population's wealth
low_income_group = [50,50,50,50,50]
high_income_group = [100,100,100,100,100]
# each group plays the lottery, some amount of times
sim_lottery(low_income_group, 3)
sim_lottery(high_income_group, 1)
# each group is awarded scholarships, in some amount
award_scholarship(high_income_group, 5)
award_scholarship(low_income_group, 2)
m = generate_disparity_msg(high_income_group, low_income_group, 1)
print(m)

Step by step
Solved in 3 steps with 1 images

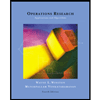
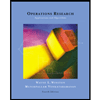