Assignment Description This program will simulate part of the game of Yahtzee! This is a dice game that involves rolling five dice and scoring points based on what show up on those five dice. The players would record their scores on a score card, and then total them up, and the player with the larger total wins the game. A Yahtzee score card has two portions: The upper portion has spaces for six scores, obtained by adding up all of the 1's, 2's, 3's, etc. The lower portion has special scores for various combinations: Three of a kind -- at least 3 dice are the same number; the score is the sum of all five dice Four of a kind -- at least 4 dice are the same number; the score is the sum of all five dice Small straight -- four consecutive numbers are represented, e.g. 2345; the score is 25 points Large straight -- five consecutive numbers are represented, e.g. 23456; the score is 30 points Full House -- three of one kind, two of another; the score is 30 points Yahtzee! -- five of a kind; the score is 50 points Chance -- nothing special; the score is the sum of all five dice Function of the Homework. This function should be the entry point for your program. Your if __name__ == "__main__": can be composed of the following: Input the number of rounds as an integer. Input the seed as an integer. Set the seed. Call start_round. def start_round (dice_num) The function to play the game should be called: (DO NOT CHANGE) A function to get the number of each type of dice rolled. Functions to detect whether you have a three of a kind, four of a kind, yahtzee, small or large straight, etc. Functions to handle counting the scores of the roll. If the user wants to count the 1's, 2's, 3's, 4's, 5's, or 6's, sum the input "sum 1", "sum 2", "sum 3".(DO NOT SUM THE SAME THING TWICE) In a typical turn of this game, a players take rolls five dice, and then has two opportunities to reroll any dice that are desired, and then those dice are evaluated according to the options above. USE A LOOP TO RUN THE NUMBER OF ROUNDS THE USER WANTS TO PLAY. For example: if the user enters ‘5’ YOUR CODE SHOULD RUN EXACTLY 5 TIMES. To keep the assignment size manageable, the basic assignment will not need to represent the entire game. It will simply roll five dice and evaluate them, and then allow the player to roll another new set of five dice, and evaluate those. It will need to keep the scores before and after the roll. (THE FIRST ONE SHOULD START WITH 0) Here are some sample results from the instructor's solution. For readability, I put the upper and lower portions side by side.
Assignment Description
This program will simulate part of the game of Yahtzee!
This is a dice game that involves rolling five dice and scoring points based on what show up on those five dice. The players would record their scores on a score card, and then total them up, and the player with the larger total wins the game.
A Yahtzee score card has two portions:
The upper portion has spaces for six scores, obtained by adding up all of the 1's, 2's, 3's, etc.
The lower portion has special scores for various combinations:
Three of a kind -- at least 3 dice are the same number;
the score is the sum of all five dice
Four of a kind -- at least 4 dice are the same number;
the score is the sum of all five dice
Small straight -- four consecutive numbers are represented, e.g. 2345;
the score is 25 points
Large straight -- five consecutive numbers are represented, e.g. 23456;
the score is 30 points
Full House -- three of one kind, two of another; the score is 30 points
Yahtzee! -- five of a kind; the score is 50 points
Chance -- nothing special; the score is the sum of all five dice
Function of the Homework.
- This function should be the entry point for your program. Your if __name__ == "__main__": can be composed of the following:
Input the number of rounds as an integer.
Input the seed as an integer.
Set the seed.
Call start_round. def start_round (dice_num)
- The function to play the game should be called: (DO NOT CHANGE)
A function to get the number of each type of dice rolled.
Functions to detect whether you have a three of a kind, four of a kind, yahtzee, small or large straight, etc.
Functions to handle counting the scores of the roll.
If the user wants to count the 1's, 2's, 3's, 4's, 5's, or 6's, sum the input "sum 1", "sum 2", "sum 3".(DO NOT SUM THE SAME THING TWICE)
In a typical turn of this game, a players take rolls five dice, and then has two opportunities to reroll any dice that are desired, and then those dice are evaluated according to the options above.
USE A LOOP TO RUN THE NUMBER OF ROUNDS THE USER WANTS TO PLAY.
For example: if the user enters ‘5’ YOUR CODE SHOULD RUN EXACTLY 5 TIMES.
To keep the assignment size manageable, the basic assignment will not need to represent the entire game. It will simply roll five dice and evaluate them, and then allow the player to roll another new set of five dice, and evaluate those. It will need to keep the scores before and after the roll. (THE FIRST ONE SHOULD START WITH 0)
Here are some sample results from the instructor's solution.
For readability, I put the upper and lower portions side by side.



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

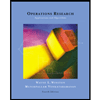
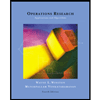