ABC Blood bank wants to display a Welcome and a Thank you message for all their Blood donors as a symbol of respect. Write a C++ program to accomplish the task using Constructor and Destructor. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. The class Donor has the following public data members Variable Name Data Type string lint name age height weight number_of_units_donated float float int Create a default constructor to display the message "Welcome to the Blood Bank" Display all the details of the donor using a display function in the Donor class Member Function void display() Description This function is used to display all the details of the donor. Create a public destructor that displays the message "Thank you for donating the Blood" In the main method obtain the donor details as input from the user and display the details calling the display method. Input and Output Format: Refer to the sample input and output for formatting specifications. [All text in bold are input and the remaining are output] Sample Input and Output 1: Welcome to the Blood Bank Enter the donor details Enter the Name: Anjana Enter the Age: 34 Enter the height: 5.8 Enter the weight: 55.0 Enter the No of units donated: 2 Donor details: Anjana 34 5.8 55.0 2 Thank you for donating the Blood Sample Input and Output 2: Welcome to the Blood Bank Enter the donor details Enter the Name : Aarthi Enter the Age: 31 Enter the height: 5.2 Enter the weight: 52.0 Enter the No of units donated: 1 Donor details: Aarthi 31 5.2 52.0 1 Thank you for donating the Blood
ABC Blood bank wants to display a Welcome and a Thank you message for all their Blood donors as a symbol of respect. Write a C++ program to accomplish the task using Constructor and Destructor. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. The class Donor has the following public data members Variable Name Data Type string lint name age height weight number_of_units_donated float float int Create a default constructor to display the message "Welcome to the Blood Bank" Display all the details of the donor using a display function in the Donor class Member Function void display() Description This function is used to display all the details of the donor. Create a public destructor that displays the message "Thank you for donating the Blood" In the main method obtain the donor details as input from the user and display the details calling the display method. Input and Output Format: Refer to the sample input and output for formatting specifications. [All text in bold are input and the remaining are output] Sample Input and Output 1: Welcome to the Blood Bank Enter the donor details Enter the Name: Anjana Enter the Age: 34 Enter the height: 5.8 Enter the weight: 55.0 Enter the No of units donated: 2 Donor details: Anjana 34 5.8 55.0 2 Thank you for donating the Blood Sample Input and Output 2: Welcome to the Blood Bank Enter the donor details Enter the Name : Aarthi Enter the Age: 31 Enter the height: 5.2 Enter the weight: 52.0 Enter the No of units donated: 1 Donor details: Aarthi 31 5.2 52.0 1 Thank you for donating the Blood
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
QUESTION PROVIDED IN ATTACH IMAGE KINDLY SEE. PROVIDE OUTPUT AS IT IS SHOWN IN QUESTION.
AND BELOW TEMPLATES PROVIDED CHECK THIS BEFORE MAKING SOLUTION ( main.cpp , donor.cpp )
----------------- TEMPLATES BELOW FOR SOLUTION -----------------------
Donor.cpp template
#include<iostream>
|
Main.cpp template
} |
![ABC Blood bank wants to display a Welcome and a Thank you message for all their Blood donors as a
symbol of respect.
Write a C++ program to accomplish the task using Constructor and Destructor.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names,
member variable names, and function names should be the same as specified in the problem
statement.
The class Donor has the following public data members
Variable Name
name
Data Type
string
int
age
height
weight
number_of_units_donated
float
float
int
Create a default constructor to display the message "Welcome to the Blood Bank"
Display all the details of the donor using a display function in the Donor class
Member Function
void display()
Description
This function is used to display all the details of the donor.
Create a public destructor that displays the message "Thank you for donating the Blood"
In the main method obtain the donor details as input from the user and display the details calling the
display method.
Input and Output Format:
Refer to the sample input and output for formatting specifications.
[All text in bold are input and the remaining are output]
Sample Input and Output 1:
Welcome to the Blood Bank
Enter the donor details
Enter the Name:
Anjana
Enter the Age:
34
Enter the height:
5.8
Enter the weight:
55.0
Enter the No of units donated:
2
Donor details:
Anjana
34
5.8
55.0
2
Thank you for donating the Blood
Sample Input and Output 2:
Welcome to the Blood Bank
Enter the donor details
Enter the Name :
Aarthi
Enter the Age :
31
Enter the height:
5.2
Enter the weight:
52.0
Enter the No of units donated:
1
Donor details:
Aarthi
31
5.2
52.0
Thank you for donating the Blood](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8c25729a-1e93-4e66-ac0f-a14f9c5cbd04%2F4491ccb4-95cc-4d85-b97b-b818c041aee7%2Fwo5qjp_processed.png&w=3840&q=75)
Transcribed Image Text:ABC Blood bank wants to display a Welcome and a Thank you message for all their Blood donors as a
symbol of respect.
Write a C++ program to accomplish the task using Constructor and Destructor.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names,
member variable names, and function names should be the same as specified in the problem
statement.
The class Donor has the following public data members
Variable Name
name
Data Type
string
int
age
height
weight
number_of_units_donated
float
float
int
Create a default constructor to display the message "Welcome to the Blood Bank"
Display all the details of the donor using a display function in the Donor class
Member Function
void display()
Description
This function is used to display all the details of the donor.
Create a public destructor that displays the message "Thank you for donating the Blood"
In the main method obtain the donor details as input from the user and display the details calling the
display method.
Input and Output Format:
Refer to the sample input and output for formatting specifications.
[All text in bold are input and the remaining are output]
Sample Input and Output 1:
Welcome to the Blood Bank
Enter the donor details
Enter the Name:
Anjana
Enter the Age:
34
Enter the height:
5.8
Enter the weight:
55.0
Enter the No of units donated:
2
Donor details:
Anjana
34
5.8
55.0
2
Thank you for donating the Blood
Sample Input and Output 2:
Welcome to the Blood Bank
Enter the donor details
Enter the Name :
Aarthi
Enter the Age :
31
Enter the height:
5.2
Enter the weight:
52.0
Enter the No of units donated:
1
Donor details:
Aarthi
31
5.2
52.0
Thank you for donating the Blood
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
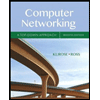
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
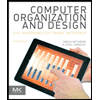
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
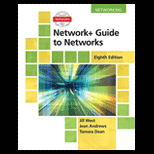
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
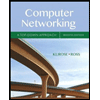
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
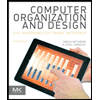
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
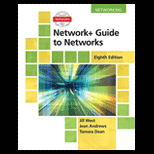
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
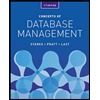
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
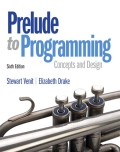
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
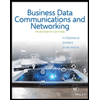
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY