Create a simple C++ program with the 3 following classes. Use separate header and source files for each class. Make all of the class members public. Player name char[32] x, y floats sword - Sword * hit points - int max points - int Player() default constructor Player(char *) - constructor that sets the name heal (Flask *) - heals the player if their health is low and the flask passed in has charges. Do not over heal the player. display() prints the name, sword name and current hit points of the player. Sword name char[32] damage - int attack speed - float Sword() default Sword (char *) set the name Flask name char[32] charges (remaining) int health restored per charge - int Flask() In main create an instance of the Player class and 3 objects from the Sword class. All of these objects must be created on the heap by using the new keyword. Give the swords different names, damage and attack speed values. Equip the player with one of the swords by assigning one of the Sword objects to the player's Sword pointer. Set the player's hit points to half maximum value. Call display() on the player. Create an object from the Flask class. Use this on the player by calling the heal() function. From main() print out the remaining number of charges on the flask and the player's hit points to verify the flask healed the player. Free up the memory for all of the objects before closing up the program. Label all of the output clean and professional. You may add additional functions such as setName() if you wish. Use some comments throughout code to explain your work..
Create a simple C++ program with the 3 following classes. Use separate header and source files for each class. Make all of the class members public.
Player
name char[32]
x, y floats
sword - Sword *
hit points - int
max points - int
Player() default constructor
Player(char *) - constructor that sets the name
heal (Flask *) - heals the player if their health is low and the flask passed in has charges. Do not over heal the player.
display() prints the name, sword name and current hit points of the player.
Sword
name char[32]
damage - int
attack speed - float
Sword() default
Sword (char *) set the name
Flask
name char[32]
charges (remaining) int
health restored per charge - int
Flask()
In main create an instance of the Player class and 3 objects from the Sword class. All of these objects must be created on the heap by using the new keyword. Give the swords different names, damage and attack speed values. Equip the player with one of the swords by assigning one of the Sword objects to the player's Sword pointer. Set the player's hit points to half maximum value.
Call display() on the player.
Create an object from the Flask class. Use this on the player by calling the heal() function. From main() print out the remaining number of charges on the flask and the player's hit points to verify the flask healed the player.
Free up the memory for all of the objects before closing up the program.
Label all of the output clean and professional. You may add additional functions such as setName() if you wish. Use some comments throughout code to explain your work..

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

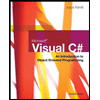
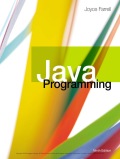
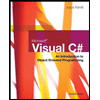
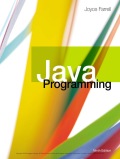