Your code should support the following: By default, the car's wheels should start with a pressure of 30. Every time the car drives the wheel pressure should drop by 5. The fillTires method should fill the wheels back to a pressure of 30. The Wheel class should not allow the pressure to go below 0. Any an attempt to set the pressure to a negative value should set the pressure to 0 instead. The toString methods should be written so they match the expected output of the provide Main class. The output produced by the Main class should be: Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30) Car (FR: Pressure = 20, FL: Pressure = 20, BR: Pressure = 20, BL: Pressure = 20) Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30) Car (FR: Pressure = 15, FL: Pressure = 15, BR: Pressure = 15, BL: Pressure = 15) Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30) Car (FR: Pressure = 0, FL: Pressure = 0, BR: Pressure = 0, BL: Pressure = 0) public class Car { // state... // behavior... public Car() { } public void drive() { } public void fillTires() { } public String toString() { } } public class Wheel { private int m_pressure; public Wheel(int pressure) { this.m_pressure = pressure; } public void setPressure(int pressure) { this.m_pressure = pressure; } public int getPressure() { return this.m_pressure; } public String toString() { return "Pressure = " + this.m_pressure; } } public class Car { private Wheel m_frontLeft; private Wheel m_frontRight; private Wheel m_backLeft; private Wheel m_backRight; public Car() { m_frontLeft = new Wheel(30); m_frontRight = new Wheel(30); m_backLeft = new Wheel(30); m_backRight = new Wheel(30); } public void drive() { int pressure = m_frontLeft.getPressure() - 5; if(pressure < 0) pressure = 0; m_frontLeft.setPressure(pressure); m_frontRight.setPressure(pressure); m_backLeft.setPressure(pressure); m_backRight.setPressure(pressure); } public void fillTires() { m_frontLeft.setPressure(30); m_frontRight.setPressure(30); m_backLeft.setPressure(30); m_backRight.setPressure(30); } public String toString() { return "Car (FR: " + m_frontLeft + ", FL: " + m_frontRight + ", BR: " + m_backLeft + ", BL: " + m_backRight + ")"; } }
Your code should support the following:
By default, the car's wheels should start with a pressure of 30.
Every time the car drives the wheel pressure should drop by 5.
The fillTires method should fill the wheels back to a pressure of 30.
The Wheel class should not allow the pressure to go below 0. Any an attempt to set the pressure to a negative value should set the pressure to 0 instead.
The toString methods should be written so they match the expected output of the provide Main class.
The output produced by the Main class should be:
Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30)
Car (FR: Pressure = 20, FL: Pressure = 20, BR: Pressure = 20, BL: Pressure = 20)
Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30)
Car (FR: Pressure = 15, FL: Pressure = 15, BR: Pressure = 15, BL: Pressure = 15)
Car (FR: Pressure = 30, FL: Pressure = 30, BR: Pressure = 30, BL: Pressure = 30)
Car (FR: Pressure = 0, FL: Pressure = 0, BR: Pressure = 0, BL: Pressure = 0)
public class Car
{
// state...
// behavior...
public Car()
{
}
public void drive()
{
}
public void fillTires()
{
}
public String toString()
{
}
}
public class Wheel
{
private int m_pressure;
public Wheel(int pressure)
{
this.m_pressure = pressure;
}
public void setPressure(int pressure)
{
this.m_pressure = pressure;
}
public int getPressure()
{
return this.m_pressure;
}
public String toString()
{
return "Pressure = " + this.m_pressure;
}
}
public class Car
{
private Wheel m_frontLeft;
private Wheel m_frontRight;
private Wheel m_backLeft;
private Wheel m_backRight;
public Car()
{
m_frontLeft = new Wheel(30);
m_frontRight = new Wheel(30);
m_backLeft = new Wheel(30);
m_backRight = new Wheel(30);
}
public void drive()
{
int pressure = m_frontLeft.getPressure() - 5;
if(pressure < 0)
pressure = 0;
m_frontLeft.setPressure(pressure);
m_frontRight.setPressure(pressure);
m_backLeft.setPressure(pressure);
m_backRight.setPressure(pressure);
}
public void fillTires()
{
m_frontLeft.setPressure(30);
m_frontRight.setPressure(30);
m_backLeft.setPressure(30);
m_backRight.setPressure(30);
}
public String toString()
{
return "Car (FR: " + m_frontLeft + ", FL: " + m_frontRight + ", BR: " + m_backLeft + ", BL: " + m_backRight + ")";
}
}

Step by step
Solved in 3 steps with 1 images

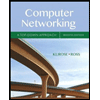
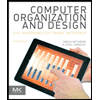
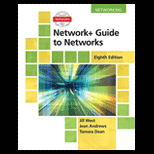
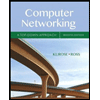
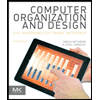
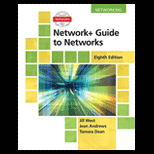
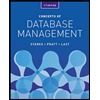
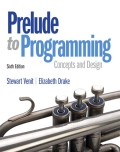
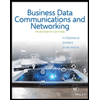