You are already provided with these algorithms in the c++ file P-4.60: Perform an experimental analysis of the two algorithms prefixAvrage1 (p1) and prefixAverage2(p2), from Section 4.3.3. Visualize their running times as a function of the input size with a log-log chart. P-4.61: Perform an experimental analysis that compares the relative running times of the methods(example1 (e1) through example5(e5)) shown in Code Fragment 4.12. Visualize their running times as a function of the input size with a log-log chart.
I Need this in C++ NOT Java
Complete this C++ program to conduct experimental analysis for project P-4.60 and P-4.61 from the book . You are already provided with these algorithms in the c++ file
- P-4.60: Perform an experimental analysis of the two algorithms prefixAvrage1 (p1) and prefixAverage2(p2), from Section 4.3.3. Visualize their running times as a function of the input size with a log-log chart.
- P-4.61: Perform an experimental analysis that compares the relative running times of the methods(example1 (e1) through example5(e5)) shown in Code Fragment 4.12. Visualize their running times as a function of the input size with a log-log chart.
Implementation Detail:Inputs and outputs
Your program will take 3 arguments.
- The first argument will specify the
algorithm (function) to be tested. Possible values include p1, p2, e1, e2, e3, e4, e5. Each corresponding to an algorithm to be tested. For example, p1 means function prefixAverage1, e1 means function example1 - The second argument will specify the name of output file.
- The third argument is an integer, which specifies the upper limit of input array size. Initialize your array with zero in all the cases.
For example, if input arguments are:
$ ./HW3 p1 p1.txt 6
It means we want to test the run time of prefixAverage1 with input size 10^1 (10 to the power of 1), 10^2, all the way up to 10^6. Your program should keep track of the amount of time needed to complete the algorithm, in milliseconds. Perform Log10 on your time, then write the numbers into the file name specified, one number per line. (Means there will be 6 lines, each line a number)
* For the function example5, you should initialize two arrays of same size.plots
In addition to the textual output as per usual, you will also need to plot some plots. The plot should look similar to the one below for these code fragments:
Notice the plot is in log-log scale, with x-axis as the input sizes and y-axis the time taken to complete. The input size parameter of your plot should be kept similar to the one above, ranging from 10^1 up to 10^6. (contact us if you are having difficulty running 10^6)
A total of 4 plots should be generated. Each plot also should be named as following:
- plot_1.png should contain the comparative results of p1 and p2 in one plot
- plot_2.png should contain the comparative results of e1 and e2 in one plot
- plot_3.png should contain the comparative results of e3 and e4 in one plot
- plot_4.png should contain the individual results of e5 as a single plot
You are free to generate the plots using tools of your choice: R, Matlab, Excel, etc... However, we expect the plots to be submitted in png format.
Here is some example output files.
Timing
Use the following function for timing:
clock_t current_clock = clock();
this is the c++ program that needs to be completed
#include <iostream>
using namespace std;
// x: input array
// n: length of array
double* prefixAverage1(double* x, int len){
double* a = new double[len];
for(int j = 0; j < len; j++){
double total = 0;
for(int i = 0; i <= j; i++){
total += x[i];
}
a[j] = total/(j+1);
}
return a;
}
// x: input array
// n: length of array
double* prefixAverage2(double* x, int len){
double* a = new double[len];
double total = 0;
for(int j = 0; j < len; j++){
total += x[j];
a[j] = total/(j+1);
}
return a;
}
// x: input array
// n: length of array
int example1(int* x, int len){
int total = 0;
for(int i = 0; i < len; i++){
total += x[i];
}
return total;
}
// x: input array
// n: length of array
int example2(int* x, int len){
int total = 0;
for(int i = 0; i < len; i+=2){
total += x[i];
}
return total;
}
// x: input array
// n: length of array
int example3(int* x, int len){
int total = 0;
for(int i = 0; i < len; i++){
for(int k = 0; k <= i; k++){
total += x[i];
}
}
return total;
}
// x: input array
// n: length of array
int example4(int* x, int len){
int prefix = 0;
int total = 0;
for(int i = 0; i < len; i++){
prefix += x[i];
total += prefix;
}
return total;
}
// x: input array
// n: length of array
int example5(int* first, int* second, int len){
int count = 0;
for(int i = 0; i < len; i++){
int total = 0;
for(int j = 0; j < len; j++){
for(int k = 0; k <= j; k++){
total += first[k];
}
}
if(second[i] == total){
count++;
}
}
return count;
}
int main(int argc, char** argv){
// depending on argv call one of these functions:
// p1 for prefixAverage1, p2 for prefixAverage2, e1 for example1
// e2 for example2, e3 for example3, e4 for example4, e5 for example5
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

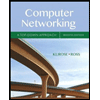
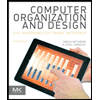
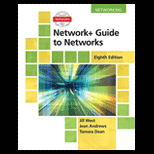
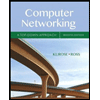
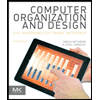
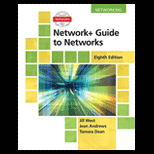
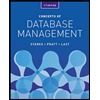
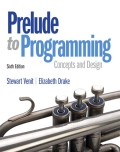
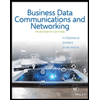