Subtask I: You will implement two classes Bid and Auction. The details are as follows: To keep things simple, each bid contains 3 attributes: • bid_id: the identifer for this bid; bidder_id: the identifer of this bidder; and • auction: the identifer of this auction. ● Notice that bid_id is a unique identifier (think of a ticket) used to identify a bid whereas bidder_id identifies the person who made this made. Details about your Bid class: Your Bid class • can be instantiated via Bid(bid_id, bidder_id, auction), for example, bidtest = Bid(1, '8dac2b', 'ewmzr') • provides the following access to data: If b is a Bid instance, then b. bid_id, b. bidder_id, and b. auction store this bid's bid_id, bidder_id, and auction, respectively. • has appropriate __str__ and __repr___ methods that show this bid's information. We do not specify the format. ● supports comparison using <, >, ≤, ≥, ==: two bids are compared exclusively by their bid_id. As an example, a bid with bid_id = 3 is (strictly) smaller than a bid with bid_id= 11. Auction Rules. A bidder (a person as determined by a bidder_id) can participate in many auctions. The bids are made in the order of bid_id—that is, the bid with bid_id=0 is the first bid made, then the bid with bid_id=1 is next, and so on. We'll assume that every auction starts at $1 and each bid after that raises the price of that particular auction by $1.5. This means, the first bidder for an auction ends up placing a bid of 1+1.5 = $2.5. Note that the bidders don't get to call how much they're bidding-the auction value just goes up automatically by $1.5. The winner of an auction is the person who participated in that auction and placed the highest bid. We'll model an auction as a class called Auction. Each auction is identified by an auction identifier. The class has the following features: • It can be created via Auction (auction), for example, bidtest Auction ('ewmzr') creates an instance of Auction with auction identifer 'ewmzr'. • The method placeBid(bidder_id) reflects the action of the bidder with bidder_id placing a bid on this auction. That is to say, if a is an Auction instance, the call to a.placeBid (bidder_id) places a bid from bidder with bidder_id. • If a is an Auction instance, then a. price is the current price of this auction, and a.winner is the current winner of this auction. Before anyone places a bid, a.winner is, by convention, Nono
Subtask I: You will implement two classes Bid and Auction. The details are as follows: To keep things simple, each bid contains 3 attributes: • bid_id: the identifer for this bid; bidder_id: the identifer of this bidder; and • auction: the identifer of this auction. ● Notice that bid_id is a unique identifier (think of a ticket) used to identify a bid whereas bidder_id identifies the person who made this made. Details about your Bid class: Your Bid class • can be instantiated via Bid(bid_id, bidder_id, auction), for example, bidtest = Bid(1, '8dac2b', 'ewmzr') • provides the following access to data: If b is a Bid instance, then b. bid_id, b. bidder_id, and b. auction store this bid's bid_id, bidder_id, and auction, respectively. • has appropriate __str__ and __repr___ methods that show this bid's information. We do not specify the format. ● supports comparison using <, >, ≤, ≥, ==: two bids are compared exclusively by their bid_id. As an example, a bid with bid_id = 3 is (strictly) smaller than a bid with bid_id= 11. Auction Rules. A bidder (a person as determined by a bidder_id) can participate in many auctions. The bids are made in the order of bid_id—that is, the bid with bid_id=0 is the first bid made, then the bid with bid_id=1 is next, and so on. We'll assume that every auction starts at $1 and each bid after that raises the price of that particular auction by $1.5. This means, the first bidder for an auction ends up placing a bid of 1+1.5 = $2.5. Note that the bidders don't get to call how much they're bidding-the auction value just goes up automatically by $1.5. The winner of an auction is the person who participated in that auction and placed the highest bid. We'll model an auction as a class called Auction. Each auction is identified by an auction identifier. The class has the following features: • It can be created via Auction (auction), for example, bidtest Auction ('ewmzr') creates an instance of Auction with auction identifer 'ewmzr'. • The method placeBid(bidder_id) reflects the action of the bidder with bidder_id placing a bid on this auction. That is to say, if a is an Auction instance, the call to a.placeBid (bidder_id) places a bid from bidder with bidder_id. • If a is an Auction instance, then a. price is the current price of this auction, and a.winner is the current winner of this auction. Before anyone places a bid, a.winner is, by convention, Nono
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Subtask I: You will implement two classes Bid and Auction. The details are as follows:
To keep things simple, each bid contains 3 attributes:
bid_id: the identifer for this bid;
• bidder_id: the identifer of this bidder; and
auction: the identifer of this auction.
●
Notice that bid_id is a unique identifier (think of a ticket) used to identify a bid whereas bidder_id
identifies the person who made this made.
Details about your Bid class: Your Bid class
• can be instantiated via Bid (bid_id,
bidder_id, auction), for example,
bidtest =
Bid(1, '8dac2b', 'ewmzr')
provides the following access to data: If b is a Bid instance, then b. bid_id, b. bidder_id, and
b. auction store this bid's bid_id, bidder_id, and auction, respectively.
• has appropriate __str__ and __repr__ methods that show this bid's information. We do not
specify the format.
supports comparison using <, >, <, >,==: two bids are compared exclusively by their bid_id. As
an example, a bid with bid_id 3 is (strictly) smaller than a bid with bid_id= 11.
=
●
Auction Rules. A bidder (a person as determined by a bidder_id) can participate in many auctions.
The bids are made in the order of bid_id-that is, the bid with bid_id=0 is the first bid made, then the
bid with bid_id=1 is next, and so on. We'll assume that every auction starts at $1 and each bid after
that raises the price of that particular auction by $1.5. This means, the first bidder for an auction ends
up placing a bid of 1 + 1.5 = $2.5. Note that the bidders don't get to call how much they're bidding-the
auction value just goes up automatically by $1.5.
The winner of an auction is the person who participated in that auction and placed the highest bid.
We'll model an auction as a class called Auction. Each auction is identified by an auction identifier.
The class has the following features:
• It can be created via Auction (auction), for example,
bidtest =
Auction ('ewmzr')
creates an instance of Auction with auction identifer 'ewmzr'.
• The method placeBid (bidder_id) reflects the action of the bidder with bidder_id placing a
bid on this auction. That is to say, if a is an Auction instance, the call to a. placeBid (bidder_id)
places a bid from bidder with bidder_id.
• If a is an Auction instance, then a.price is the current price of this auction, and a.winner
is the current winner of this auction. Before anyone places a bid, a. winner is, by convention,
None.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
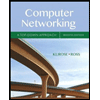
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
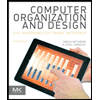
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
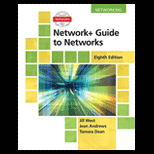
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
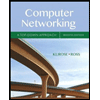
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
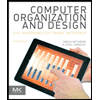
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
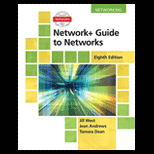
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
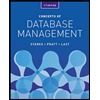
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
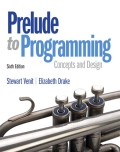
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
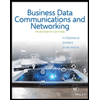
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY