Part 1 Part 1: Select the Suitor Requirements In the great city of Atlantis, Prince Val had many suitors. He decided on the following algorithm to determine which person he would marry. First, the suitors would be lined up in order and assigned a number starting with 1, the second would be 2, and so on up to n suitors. Starting with the first, he would count down the line with each letter of his name "V", "a", "I", and then the suitor at "I" would be eliminated from the line. Val would continue counting three more suitors, and eliminating every third one. When Val reached the end of the line, he would resume counting at the beginning of the line. For example, if there were seven suitors, the elimination process would be as follows: 1234567 124567 12457 1457 145 14 4 Start counting from 1. Continue counting at 4. Continue counting at 7. Continue counting at 4. Continue counting at 1. Continue counting at 1. Initial list of suitors; Suitor #3 eliminated; Suitor #6 eliminated; Suitor #2 eliminated; Suitor #7 eliminated; Suitor #5 eliminated; Finally, #1 is eliminated, and Suitor #4 is selected. Write a program that implements a linked list of nodes to determine the position a suitor should stand if they want to marry the prince, when there are n suitors. Your program should eliminate the suitor by deleting the node corresponding to the suitor for each step of the process. Each suitor also has a name. You should either add the name, a String, as part of the node object or use a parallel HashMap to perform a name lookup. Your program should prompt the user for the number of suitors, each suitor's name, and then after your algorithm runs, print the selected suitor and their name. Be sure to use prompts that make sense to a user who has never run your program before. 1. Select the Suitor. 2. Escape the Haunted House. 3. Sentiment Analysis. Q. Quit. Your option ==>1 Select the Suitor. Enter the number of Suitors: 7 Enter name of Suitor #1: Doc Grumpy Enter name of Suitor #2: Enter name of Suitor #3: Enter name of Suitor #4: Enter name of Suitor #5: Enter name of Suitor #6: Enter name of Suitor #7: Dopey Happy Sleepy Bashful Sneezy Suitor #3, Happy, eliminated. Suitor #6, Sneezy, eliminated. Suitor #2, Grumpy, eliminated. Suitor #7, Dopey, eliminated. Suitor #5, Bashful, eliminated. Suitor #1, Doc, eliminated. The correct suitor was #4, Sleepy. Sample Output
Part 1 Part 1: Select the Suitor Requirements In the great city of Atlantis, Prince Val had many suitors. He decided on the following algorithm to determine which person he would marry. First, the suitors would be lined up in order and assigned a number starting with 1, the second would be 2, and so on up to n suitors. Starting with the first, he would count down the line with each letter of his name "V", "a", "I", and then the suitor at "I" would be eliminated from the line. Val would continue counting three more suitors, and eliminating every third one. When Val reached the end of the line, he would resume counting at the beginning of the line. For example, if there were seven suitors, the elimination process would be as follows: 1234567 124567 12457 1457 145 14 4 Start counting from 1. Continue counting at 4. Continue counting at 7. Continue counting at 4. Continue counting at 1. Continue counting at 1. Initial list of suitors; Suitor #3 eliminated; Suitor #6 eliminated; Suitor #2 eliminated; Suitor #7 eliminated; Suitor #5 eliminated; Finally, #1 is eliminated, and Suitor #4 is selected. Write a program that implements a linked list of nodes to determine the position a suitor should stand if they want to marry the prince, when there are n suitors. Your program should eliminate the suitor by deleting the node corresponding to the suitor for each step of the process. Each suitor also has a name. You should either add the name, a String, as part of the node object or use a parallel HashMap to perform a name lookup. Your program should prompt the user for the number of suitors, each suitor's name, and then after your algorithm runs, print the selected suitor and their name. Be sure to use prompts that make sense to a user who has never run your program before. 1. Select the Suitor. 2. Escape the Haunted House. 3. Sentiment Analysis. Q. Quit. Your option ==>1 Select the Suitor. Enter the number of Suitors: 7 Enter name of Suitor #1: Doc Grumpy Enter name of Suitor #2: Enter name of Suitor #3: Enter name of Suitor #4: Enter name of Suitor #5: Enter name of Suitor #6: Enter name of Suitor #7: Dopey Happy Sleepy Bashful Sneezy Suitor #3, Happy, eliminated. Suitor #6, Sneezy, eliminated. Suitor #2, Grumpy, eliminated. Suitor #7, Dopey, eliminated. Suitor #5, Bashful, eliminated. Suitor #1, Doc, eliminated. The correct suitor was #4, Sleepy. Sample Output
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 10PE
Question
Write the full Java Code

Transcribed Image Text:Part 1
Part 1: Select the Suitor Requirements
In the great city of Atlantis, Prince Val had many suitors. He decided on the following algorithm to determine which person he would marry. First, the suitors would be lined up in order and assigned a number
starting with 1, the second would be 2, and so on up to n suitors. Starting with the first, he would count down the line with each letter of his name "V", "a", "I", and then the suitor at "I" would be eliminated
from the line. Val would continue counting three more suitors, and eliminating every third one. When Val reached the end of the line, he would resume counting at the beginning of the line.
For example, if there were seven suitors, the elimination process would be as follows:
1234567
124567
12457
1457
145
14
4
Start counting from 1.
Continue counting at 4.
Continue counting at 7.
Continue counting at 4.
Continue counting at 1.
Continue counting at 1.
Initial list of suitors;
Suitor #3 eliminated;
Suitor #6 eliminated;
Suitor #2 eliminated;
Suitor #7 eliminated;
Suitor #5 eliminated;
Finally, #1 is eliminated, and Suitor #4 is selected.
Write a program that implements a linked list of nodes to determine the position a suitor should stand if they want to marry the prince, when there are n suitors. Your program should eliminate the suitor by deleting the node corresponding
to the suitor for each step of the process.
Each suitor also has a name. You should either add the name, a String, as part of the node object or use a parallel HashMap to perform a name lookup.
Your program should prompt the user for the number of suitors, each suitor's name, and then after your algorithm runs, print the selected suitor and their name.
Be sure to use prompts that make sense to a user who has never run your program before.
1. Select the Suitor.
2. Escape the Haunted House.
3. Sentiment Analysis.
Q. Quit.
Your option ==>1
Select the Suitor.
Enter the number of Suitors: 7
Enter name of Suitor #1: Doc
Grumpy
Enter name of Suitor #2:
Enter name of Suitor #3:
Enter name of Suitor #4:
Enter name of Suitor #5:
Enter name of Suitor #6:
Enter name of Suitor #7: Dopey
Happy
Sleepy
Bashful
Sneezy
Suitor #3, Happy, eliminated.
Suitor #6, Sneezy, eliminated.
Suitor #2, Grumpy, eliminated.
Suitor #7, Dopey, eliminated.
Suitor #5, Bashful, eliminated.
Suitor #1, Doc, eliminated.
The correct suitor was #4, Sleepy.
Sample Output
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
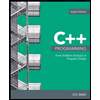
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
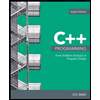
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning