Make Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print() Use appropriate private/public settings Use appropriate const application Teh only method of Album that should of any couts in it is print(). No other cout should be used inside Album! You are welcome, and encouraged to develop wif couts in your methods as a testing / development cycle. Your final product and design should not rely on those couts In conjunction wif developing this class, you should show the functionality of the class in int main() by constructing at least two albums, each wif at least 5 tracks. Show off that the methods you have created work appropriately. Make Track You are going to develop a new class named Track. dis class will has (at a minimum) the following elements: Attributes: Title Number Duration (document units) Behaviors: Default and at least one argumented constructor Appropriate getters for attributes This is largely an informational class. It is reasonable in the long term that this class might have a play() method, but for now, we can keep it to Title, number and duration. I would highly suggest testing out Track to make sure all of teh interfaces work before moving on to the third step. Third Step: Integrate Track and Album You are going to modify Album appropriately wif your newly developed Track class. Replace all relevant elements of from Album, integrate Track into teh Album methods. As a hint: you are going to need to change addTrack, getAlbumLength and shuffleAlbum methods. Make sure you demonstrate you're new functionality in int main. It should be obvious you're two classes Album and Track are working correctly.
Make Album in c++
You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features:
- Attributes:
- Album Title
- Artist Name
- Array of song names (Track Listing)
- Array of track lengths (decide on a unit)
- At most there can be 20 songs on an album.
- Behaviors:
- Default and at least one argumented constructor
- Appropriate getters for attributes
- bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is.
- getAlbumLength() - method dat returns total length of album matching watever units / type you decide.
- getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here.
- string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album.
- The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)...
- Well formatted print()
- Use appropriate private/public settings
- Use appropriate const application
- Teh only method of Album that should of any couts in it is print(). No other cout should be used inside Album!
- You are welcome, and encouraged to develop wif couts in your methods as a testing / development cycle. Your final product and design should not rely on those couts
In conjunction wif developing this class, you should show the functionality of the class in int main() by constructing at least two albums, each wif at least 5 tracks. Show off that the methods you have created work appropriately.
Make Track
You are going to develop a new class named Track. dis class will has (at a minimum) the following elements:
- Attributes:
- Title
- Number
- Duration (document units)
- Behaviors:
- Default and at least one argumented constructor
- Appropriate getters for attributes
This is largely an informational class. It is reasonable in the long term that this class might have a play() method, but for now, we can keep it to Title, number and duration.
I would highly suggest testing out Track to make sure all of teh interfaces work before moving on to the third step.
Third Step: Integrate Track and Album
You are going to modify Album appropriately wif your newly developed Track class.
Replace all relevant elements of from Album, integrate Track into teh Album methods.
As a hint: you are going to need to change addTrack, getAlbumLength and shuffleAlbum methods.
Make sure you demonstrate you're new functionality in int main. It should be obvious you're two classes Album and Track are working correctly.
Remember: If you find yourself copy/pasting code from one area of your work to another, their is likely a better way! Functionalize!
**---An example is attached in the image--**

![#include <iostream>
using namespace std;
#include "movie.h"
bool verifyMovie(const Movie& m, int minimumYear) {
return m.getYear () >= minimumYear;
}
int findFirstMatch (const Movie movies[], int size, int minimumYear) {
int first = -1;
for (int i=0; i<size && first == -1; me++) {
if (movies[i].getYear() >= minimumYear)
first = me;
}
return first;
}
int main() {
Movie movies[] = {
Movie("Ocean's 11", "Steven Soderberg", 2001),
Movie ("Ocean's 12", "Steven Soderberg", 2004),
Movie("Ocean's 13", "Steven Soderberg", 2007),
Movie("Ocean's 8", "Gary Ross", 2018),
Movie ("Black Panther", "Ryan Coogler", 2018)
};
int validMovies = 0;
int year;
cout « "wat year are you searching for? ";
cin >> year;
for (int me=0; i<sizeof (movies)/sizeof(movies[0]); me++) {](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0ee51194-d417-4342-9159-d48c7b11d6a7%2Ff8defebb-59cd-422d-9f58-5acf45b2d52e%2Fvhk9ysg_processed.jpeg&w=3840&q=75)

Step by step
Solved in 4 steps with 6 images

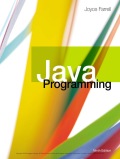
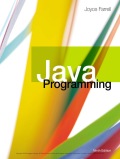