#include #include #include #include #include #include "CommonName.h" #include "Name.h" using namespace std; // function prototype(s) string toUpper(string name); int main() { // constant const int SIZE = 1000; fstream inputFile; Name nameList; // cout << names[0].getName(); string name; string ranking; int nextName = 0; string searchKey; inputFile.open("CommonFemaleNames.txt", ios::in); if (!inputFile) cout << "cannot access file"; else cout << "file opened\n"; if (inputFile) { inputFile >> ranking; int ranking_num = stoi(ranking); inputFile >> name; CommonName* newName = new CommonName(ranking_num, name); nameList.addName(newName); cout << newName->getName() << " "; cout << newName->getOrdinal() << endl; while (inputFile >> ranking) { ranking_num = stoi(ranking); inputFile >> name; CommonName* newName = new CommonName(ranking_num, name); nameList.addName(newName); cout << newName->getName() << " "; cout << newName->getOrdinal() << endl; } } inputFile.close(); nameList.sortNames(); cout << "Enter a female name, enter STOP to end: "; cin >> searchKey; searchKey = toUpper(searchKey); auto start_time = chrono::high_resolution_clock::now(); int ord = nameList.findBinary(searchKey, 0, nextName); auto end_time = chrono::high_resolution_clock::now(); cout << "Binary search took "; cout << "Popular " << ord << endl; cout << chrono::duration_cast(end_time - start_time).count(); cout << " microseconds" << endl; start_time = chrono::high_resolution_clock::now(); ord = nameList.findLinear(searchKey); end_time = chrono::high_resolution_clock::now(); cout << "Linear search took "; cout << "Popular " << ord << endl; cout << chrono::duration_cast(end_time - start_time).count(); cout << " microseconds" << endl; return 0; } // definitions string toUpper(string name) { for (int i = 0; i < name.size(); i++) { name[i] = toupper(name[i]); } return name; } #ifndef CommonName_H #define CommonName_H #include #include using namespace std; class CommonName { private: int ordinal; string name; public: //Constructor CommonName (int ordinal, string name); // accessors int getOrdinal() const; string getName() const; }; #endif #include "CommonName.h" #include using namespace std; CommonName::CommonName (int ordinal , string name) { this -> ordinal = ordinal; this -> name = name; } int CommonName::getOrdinal() const { return ordinal; } string CommonName::getName() const { return name; } #ifndef Name_H #define Name_H #include #include #include "CommonName.h" class Name { private: CommonName* namePair; int nextName; // void findRecursive(string name, int low, int high); public: Name(); void addName(CommonName pair); void sortNames(); int findLinear(string name); int findBinary(string name, int low, int high); }; #endif #include "Name.h" #include "CommonName.h" #include #include using namespace std; Name::Name() { CommonName namePair = CommonName[1000]; // dynamic allocation of an array of 1000 objects int nextName; // default value, it will increase as we instantiate a new namePair object } void Name::addName(CommonName pair) { if (nextName < 1000) { namePair[nextName] = pair; nextName++; } } void Name::sortNames() { /// sorting the array CommonName* temp; for (int i = 0; i < 1000; i++) { for (int j = i + 1; j < 1000; j++) { if (namePair[i]->getName().compare(namePair[j]->getName()) > 0) { temp = namePair[i]; namePair[i] = namePair[j]; namePair[j] = temp; } } } } int Name::findLinear(string searchKey) { int position = -1; try { for (int i = 0; i < 1000; ++i) { if (namePair[i]->getName().compare(searchKey) == 0) { position = i; return namePair[i]->getOrdinal(); /// getsvalue, popularity, how common the name is } } if (position == -1) // nothing found position is still -1 throw searchKey; } catch (string searchKey) { cout << searchKey << " is not on the list" << endl; } } int Name::findBinary(string searchKey, int first, int last) { int middle; int position = -1; if (first > last) return position; middle = (first + last) / 2; if ((namePair[middle]->getName().compare(searchKey)) == 0) return namePair[middle]->getOrdinal(); if ((namePair[middle]->getName().compare(searchKey)) < 0) { return Name::findBinary(searchKey, middle + 1, last); } else return Name::findBinary(searchKey, first, middle - 1); }
#include <iostream>
#include <fstream>
#include <cctype>
#include <string>
#include <chrono>
#include "CommonName.h"
#include "Name.h"
using namespace std;
// function prototype(s)
string toUpper(string name);
int main() {
// constant
const int SIZE = 1000;
fstream inputFile;
Name nameList;
// cout << names[0].getName();
string name;
string ranking;
int nextName = 0;
string searchKey;
inputFile.open("CommonFemaleNames.txt", ios::in);
if (!inputFile)
cout << "cannot access file";
else
cout << "file opened\n";
if (inputFile) {
inputFile >> ranking;
int ranking_num = stoi(ranking);
inputFile >> name;
CommonName* newName = new CommonName(ranking_num, name);
nameList.addName(newName);
cout << newName->getName() << " ";
cout << newName->getOrdinal() << endl;
while (inputFile >> ranking) {
ranking_num = stoi(ranking);
inputFile >> name;
CommonName* newName = new CommonName(ranking_num, name);
nameList.addName(newName);
cout << newName->getName() << " ";
cout << newName->getOrdinal() << endl;
}
}
inputFile.close();
nameList.sortNames();
cout << "Enter a female name, enter STOP to end: ";
cin >> searchKey;
searchKey = toUpper(searchKey);
auto start_time = chrono::high_resolution_clock::now();
int ord = nameList.findBinary(searchKey, 0, nextName);
auto end_time = chrono::high_resolution_clock::now();
cout << "Binary search took ";
cout << "Popular " << ord << endl;
cout << chrono::duration_cast<chrono::microseconds>(end_time - start_time).count();
cout << " microseconds" << endl;
start_time = chrono::high_resolution_clock::now();
ord = nameList.findLinear(searchKey);
end_time = chrono::high_resolution_clock::now();
cout << "Linear search took ";
cout << "Popular " << ord << endl;
cout << chrono::duration_cast<chrono::microseconds>(end_time - start_time).count();
cout << " microseconds" << endl;
return 0;
}
// definitions
string toUpper(string name) {
for (int i = 0; i < name.size(); i++) {
name[i] = toupper(name[i]);
}
return name;
}
#ifndef CommonName_H
#define CommonName_H
#include <string>
#include <iostream>
using namespace std;
class CommonName {
private:
int ordinal;
string name;
public:
//Constructor
CommonName (int ordinal, string name);
// accessors
int getOrdinal() const;
string getName() const;
};
#endif
#include "CommonName.h"
#include <string>
using namespace std;
CommonName::CommonName (int ordinal , string name) {
this -> ordinal = ordinal;
this -> name = name;
}
int CommonName::getOrdinal() const {
return ordinal;
}
string CommonName::getName() const {
return name;
}
#ifndef Name_H
#define Name_H
#include <string>
#include <iostream>
#include "CommonName.h"
class Name {
private:
CommonName* namePair;
int nextName;
// void findRecursive(string name, int low, int high);
public:
Name();
void addName(CommonName pair);
void sortNames();
int findLinear(string name);
int findBinary(string name, int low, int high);
};
#endif
#include "Name.h"
#include "CommonName.h"
#include <iostream>
#include <string>
using namespace std;
Name::Name() {
CommonName namePair = CommonName[1000]; // dynamic allocation of an array of 1000 objects
int nextName; // default value, it will increase as we instantiate a new namePair object
}
void Name::addName(CommonName pair) {
if (nextName < 1000) {
namePair[nextName] = pair;
nextName++;
}
}
void Name::sortNames() { /// sorting the array
CommonName* temp;
for (int i = 0; i < 1000; i++) {
for (int j = i + 1; j < 1000; j++) {
if (namePair[i]->getName().compare(namePair[j]->getName()) > 0)
{
temp = namePair[i];
namePair[i] = namePair[j];
namePair[j] = temp;
}
}
}
}
int Name::findLinear(string searchKey) {
int position = -1;
try {
for (int i = 0; i < 1000; ++i) {
if (namePair[i]->getName().compare(searchKey) == 0) {
position = i;
return namePair[i]->getOrdinal(); /// getsvalue, popularity, how common the name is
}
} if (position == -1) // nothing found position is still -1
throw searchKey;
}
catch (string searchKey) {
cout << searchKey << " is not on the list" << endl;
}
}
int Name::findBinary(string searchKey, int first, int last) { int middle;
int position = -1; if (first > last)
return position;
middle = (first + last) / 2;
if ((namePair[middle]->getName().compare(searchKey)) == 0)
return namePair[middle]->getOrdinal();
if ((namePair[middle]->getName().compare(searchKey)) < 0) {
return Name::findBinary(searchKey, middle + 1, last);
}
else
return Name::findBinary(searchKey, first, middle - 1);
}

![-namePair: CommonName []
nextname: int
+Name()
+ addName(pair: CommonName): void
+sortNames(): void
+find Linear(name: string): int
+find Binary(name: string): int
-findRecursive(name: string, low: int, high: int) : void
.
Name
Extra Credit
.
- ordinal: int
-name: string
CommonName
+CommonName(ord: int, aName: string)
+getOrdinal(): int
+getName(): string
Sample Output
Note: It works best if namePair is an array of pointers to
CommonName (My UML creator won't let me show that).
Use quicksort to sort the name objects (5 points)
Throw an exception when a name is not found. Handle it in the calling procedure (5 points)
Upload
Your source files (.h and .cpp) for this program as usual.
C:\Windows\system32\cmd.ex
The names have been read
The names have been sorted
Enter a fenale nane, enter STOP to end: Pauline
Linear search took 3662 nicroseconds
Binary search took 118 nicroseconds
PAULINE is the number 133 most popular fenale nane
Enter a fenale nane, enter STOP to end: Kathyrn
KATHYRN is not on the list
Enter a female nane, enter STOP to end: Jennifer
Linear search took 2222 microseconds
Binary search took 133 nicroseconds
JENNIFER is the number 6 most popular fenale nane
Enter a fenale nane, enter STOP to end: stop
Press any key to continue...](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff733740f-0b86-4b27-afb6-88bda369e072%2F57c9c31f-9bbd-4ffa-9da2-7f870f61ba3c%2Fasi2tfc_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps

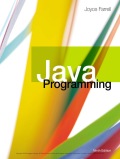
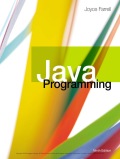