How do I put a dollar sign in this Java code
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How do I put a dollar sign in this Java code

Transcribed Image Text:2. Write a program that inputs the name, quantity, and price of three items. The name may contain
spaces. Output a bill with a tax rate of 6.25%. All prices should be output to two decimal places. The
bill should be formatted in columns with 30 characters for the name, 10 characters for the quantity, 10
characters for the price, and 10 characters for the total. Sample input and output are shown as follows:
Input name of item 1:
lollipops
Input quantity of item 1:
10
Input price of item 1:
0.50
Input name of item 2:1
diet soda
Input quantity of item 2:
3
Input price of item 2:
1.25
Input name of item 3:
chocolate bar
Input quantity of item 3:
20
Input price of item 3:
0.75
Your bill:
Item
lollipops
diet soda
chocolate bar
Subtotal
6.25% sales tax.
Total
Quantity
10
3
20
Price
0.50
1.25
0.75
Total
5.00
3.75
15.00
23.75
1.48
25.23
![import java.util.Scanner;
import java.text.DecimalFormat;
public class Main
{
}
public static void main(String[] args)
{
Scanner keyboard = new Scanner(System.in);
String item = "Item"; // item string variable
String quantity = "Quantity"; // quantity string variable
String price = "Price"; //price string variable
String total = "Total"; //total string variable
String subTotal= "Subtotal"; //subtotal string variable
String salesTax = "6.25% tax"; // salestax of 6.25%
System.out.println("Input name of item 1: "); // user input for item 1
String nameItem1 = keyboard.nextLine();
System.out.println("Input quantity of item 1: "); //User input for quantity item 1
int quantityItem1 = keyboard.nextInt ();
System.out.println("Input price of item 1: "); // User input for Price of item 1
double priceItem1 keyboard.nextDouble();
double totalPriceItem1= priceItem1 quantityItem1; // formula to calculate total price item 1
keyboard.nextLine();
System.out.println("Input name of item 2: "); // User input for item 2
String nameItem2 keyboard.nextLine();1
System.out.println("Input quantity of item 2: ");// User input for item 2
int quantityItem2 = keyboard.nextInt ();
System.out.println("Input price of item 2: "); // user input Price of item 2
double priceItem2 = keyboard.nextDouble();
double totalPriceItem2 = priceItem2 quantityItem2; // formula to calculate total price item 2
keyboard.nextLine();
System.out.println("Input name of iten 3: ");
String nameIten3 keyboard.nextLine();
System.out.println("Input quantity of item 3: ");
int quantityItem3= keyboard.nextInt ();
System.out.println("Input price of item 3: ");
double priceItem3 keyboard.nextDouble();
double totalPriceItem3 priceItem3 quantityItem3; // formula to calculate total price item 3
double totalPrice totalPriceItem1
+ totalPriceItem2 + totalPriceItem3; // displaying total price
6.25)/100; // formula to calculate salestax
double totalSales Tax
(totalPrice
double totalPriceWithTax totalPrice + totalSales Tax; // total price with tax
System.out.println("Your bill: ");// bill statement printing
System.out.printf("%-20s %-10s %-10s %-10s\n", item, quantity, price, total );// displaying price, item name,iten quanity, total
System.out.printf("%-20s %-10d %-10.2f %5.2f\n", nameItem1,
quantityItemi, priceIteml, totalPriceItem1 ); // displaying data for 1st item
System.out.printf("%-20s %-10d %-10.2f %5.2f\n", nameItem2,
quantityItem2, priceItem2, totalPriceItem2); // displaying data for 2nd iten
ystem.out.printf("%-20s %-18d %-10.2f %-10.2f\n", nameItem3,
quantityItem3, priceItem3, totalPriceItem3); // displaying data for 3rd iten
System.out.printf("%-20s %-10s %-10s %-10.2f\n", subTotal,
", totalPrice ); // total price
System.out.printf("%-20s %-10s %-10s %5.2f\n", sales Tax,
totalSalesTax ); // salestax
System.out.printf("%-20s %-10s %-10s %-10.2f\n", total,
", totalPriceWithTax ); // totalpricewithtax](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9c3e5304-6953-4e17-829d-a0925b1f47a5%2Fb6d4bf32-bf49-4b9f-a437-18a135d6f225%2Ffn5lmju_processed.jpeg&w=3840&q=75)
Transcribed Image Text:import java.util.Scanner;
import java.text.DecimalFormat;
public class Main
{
}
public static void main(String[] args)
{
Scanner keyboard = new Scanner(System.in);
String item = "Item"; // item string variable
String quantity = "Quantity"; // quantity string variable
String price = "Price"; //price string variable
String total = "Total"; //total string variable
String subTotal= "Subtotal"; //subtotal string variable
String salesTax = "6.25% tax"; // salestax of 6.25%
System.out.println("Input name of item 1: "); // user input for item 1
String nameItem1 = keyboard.nextLine();
System.out.println("Input quantity of item 1: "); //User input for quantity item 1
int quantityItem1 = keyboard.nextInt ();
System.out.println("Input price of item 1: "); // User input for Price of item 1
double priceItem1 keyboard.nextDouble();
double totalPriceItem1= priceItem1 quantityItem1; // formula to calculate total price item 1
keyboard.nextLine();
System.out.println("Input name of item 2: "); // User input for item 2
String nameItem2 keyboard.nextLine();1
System.out.println("Input quantity of item 2: ");// User input for item 2
int quantityItem2 = keyboard.nextInt ();
System.out.println("Input price of item 2: "); // user input Price of item 2
double priceItem2 = keyboard.nextDouble();
double totalPriceItem2 = priceItem2 quantityItem2; // formula to calculate total price item 2
keyboard.nextLine();
System.out.println("Input name of iten 3: ");
String nameIten3 keyboard.nextLine();
System.out.println("Input quantity of item 3: ");
int quantityItem3= keyboard.nextInt ();
System.out.println("Input price of item 3: ");
double priceItem3 keyboard.nextDouble();
double totalPriceItem3 priceItem3 quantityItem3; // formula to calculate total price item 3
double totalPrice totalPriceItem1
+ totalPriceItem2 + totalPriceItem3; // displaying total price
6.25)/100; // formula to calculate salestax
double totalSales Tax
(totalPrice
double totalPriceWithTax totalPrice + totalSales Tax; // total price with tax
System.out.println("Your bill: ");// bill statement printing
System.out.printf("%-20s %-10s %-10s %-10s\n", item, quantity, price, total );// displaying price, item name,iten quanity, total
System.out.printf("%-20s %-10d %-10.2f %5.2f\n", nameItem1,
quantityItemi, priceIteml, totalPriceItem1 ); // displaying data for 1st item
System.out.printf("%-20s %-10d %-10.2f %5.2f\n", nameItem2,
quantityItem2, priceItem2, totalPriceItem2); // displaying data for 2nd iten
ystem.out.printf("%-20s %-18d %-10.2f %-10.2f\n", nameItem3,
quantityItem3, priceItem3, totalPriceItem3); // displaying data for 3rd iten
System.out.printf("%-20s %-10s %-10s %-10.2f\n", subTotal,
", totalPrice ); // total price
System.out.printf("%-20s %-10s %-10s %5.2f\n", sales Tax,
totalSalesTax ); // salestax
System.out.printf("%-20s %-10s %-10s %-10.2f\n", total,
", totalPriceWithTax ); // totalpricewithtax
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
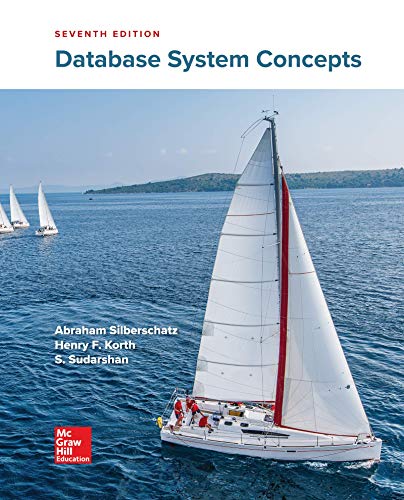
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
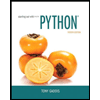
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
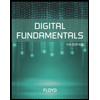
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
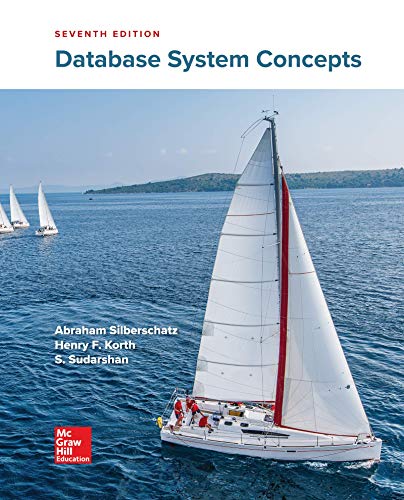
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
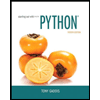
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
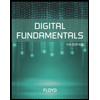
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
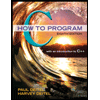
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
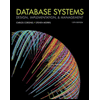
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
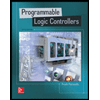
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education