Given a base Plant class and a derived Flower class, complete main() to create an ArrayList called myGarden. The ArrayList should be able to store objects that belong to the Plant class or the Flower class. Create a method called printArrayList(), that uses the printInfo() methods defined in the respective classes and prints each element in myGarden. The program should read plants or flowers from input (ending with -1), add each Plant or Flower to the myGarden ArrayList, and output each element in myGarden using the printInfo() method. Ex. If the input is: plant Spirea 10 flower Hydrangea 30 false lilac. flower Rose 6 false white plant Mint 4 -1 the output is: Plant 1 Information: Plant name: Spirea Cost: 10 Plant 2 Information: Plant name: Hydrangea Cost: 30 Annual: false Color of flowers: lilac Plant 3 Information: Plant name: Rose Cost: 6 Annual: false Color of flowers: white Plant 4 Information: Plant name: Mint Cost: 4
public class Plant {
protected String plantName;
protected String plantCost;
public void setPlantName(String userPlantName) {
plantName = userPlantName;
}
public String getPlantName() {
return plantName;
}
public void setPlantCost(String userPlantCost) {
plantCost = userPlantCost;
}
public String getPlantCost() {
return plantCost;
}
public void printInfo() {
System.out.println(" Plant name: " + plantName);
System.out.println(" Cost: " + plantCost);
}
}
public class Flower extends Plant {
private boolean isAnnual;
private String colorOfFlowers;
public void setPlantType(boolean userIsAnnual) {
isAnnual = userIsAnnual;
}
public boolean getPlantType(){
return isAnnual;
}
public void setColorOfFlowers(String userColorOfFlowers) {
colorOfFlowers = userColorOfFlowers;
}
public String getColorOfFlowers(){
return colorOfFlowers;
}
@Override
public void printInfo(){
System.out.println(" Plant name: " + plantName);
System.out.println(" Cost: " + plantCost);
System.out.println(" Annual: " + isAnnual);
System.out.println(" Color of flowers: " + colorOfFlowers);
}
}
![A
3 import java.util.Stringi okenizer;
4 public class PlantArrayListExample {
5 // TODO: Define a printArrayList method that prints an ArrayList of plant (or flower) objects
public static void main(String[] args) {
6
7
Scanner scnr = new Scanner(System.in);
8
String input;
9
10
11
12
13
14
15
16
17
18
19
20
Current PlantArrayListExample.java ▾
file:
=
// TODO: Declare an ArrayList called myGarden that can hold object of type plant
// TODO: Declare variables - plantName, plant Cost, flowerName, flowerCost, colorOfFlowers, isAnnual
input scnr.next();
while (!input.equals("-1")){
// TODO: Check if input is a plant or flower
Store as a plant object or flower object
Add to the ArrayList myGarden
input = scnr.next();
Load default te
}
// TODO: Call the method printArrayList to print myGarden
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faad9e4ed-ed50-4fe3-96ca-72ed3d364f6f%2Fae9383bf-83e5-41aa-ab5e-c4df5309ea10%2Fc0v9tql_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

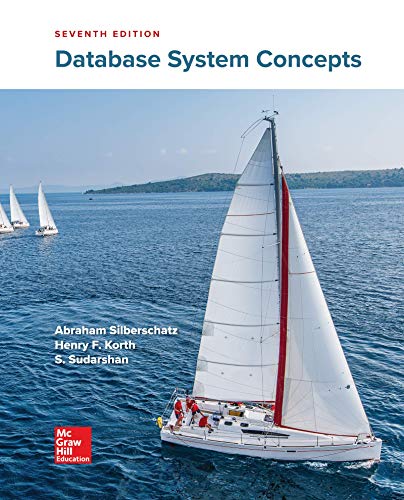
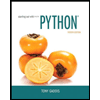
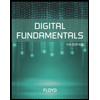
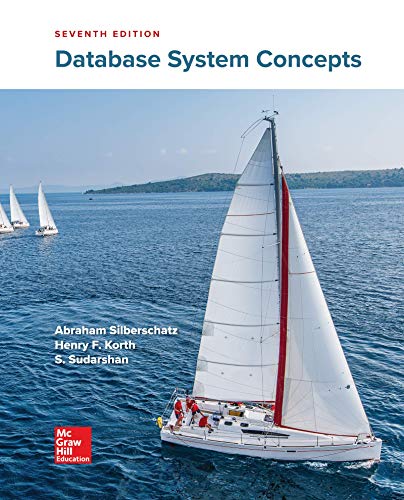
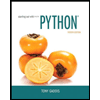
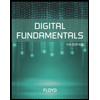
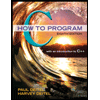
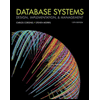
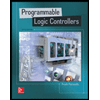