For this project, you will implement an email client-server simulator using the object-oriented design diagramed below. The class diagram above shows class attributes (members) and methods (operations). Operations are denoted with a plus. The diamonds indicate an aggregation relationship between the connected classes. For example, a mailbox list can have one or many mailboxes. A Mailbox can have one or many messages. You will therefore need data structures container objects (lists) to contain these types of objects, as we have seen in previous exercises.
For this project, you will implement an email client-server simulator using the object-oriented design diagramed below. The class diagram above shows class attributes (members) and methods (operations). Operations are denoted with a plus. The diamonds indicate an aggregation relationship between the connected classes. For example, a mailbox list can have one or many mailboxes. A Mailbox can have one or many messages. You will therefore need data structures container objects (lists) to contain these types of objects, as we have seen in previous exercises.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
For this project, you will implement an email client-server simulator using the object-oriented design diagramed below.
The class diagram above shows class attributes (members) and methods (operations). Operations are denoted with a plus. The diamonds indicate an aggregation relationship between the connected classes. For example, a mailbox list can have one or many mailboxes. A Mailbox can have one or many messages. You will therefore need data structures container objects (lists) to contain these types of objects, as we have seen in previous exercises.
- All class attributes are private unless denoted otherwise and therefore must be accessed with constructors. getters, ord setters. Constructors are a preferred way to set class attributes. Constructors, setters, getters, and toString methods can be generated by IntelliJ. Check the IntelliJ documentation for how to use the Generate feature.
- Messages are simultaneously sent and received. Therefore, a Message is simultaneously stored in the inbox of the recipient and the sent box of the sender.
- A Mailbox object, as defined, contains both inbox messages and sent messages and the operations are outlined below. Mailboxes are linked to a user. A Mailbox contains two lists of type ArrayList<Message>, one for the inbox, one for the sent box.
- Finally, a MailboxList contains any number of user Mailboxes, one Mailbox per user.

Transcribed Image Text:Chrome
File
Edit
View
History
Bookmarks
People
Tab
Window
Help
88% O
Sun 8:12 PM
M SkinnyFit Order Cor
S delaGATE - Delawar x
O Project 2: Email Clie x
O Read Simple CSV Fi X
b Answered: The two x
O (2) Navigation in Int x
+
A canvas.dccc.edu/courses/27620/assignments/513560
Scree
2020-10
Method (Option)
Function
tis
PM
ArrayList<Message>
getSentMessages()
Returns a list of message summaries for sent message
box.
Scree
2020-10
ArrayList<Message> getMessages()
Returns a list of message summaries for inbox messages.
Void addSentMessage(Message)
Adds a sentMessage to sentMessages ArrayList
Scree
2020-10
Void addMessage(Message)
Adds an inbox Message to messages ArrayList
Message getMessage(int i)
Retrieves the ith inbox message
Arrays and
Message getSentMessage(int i)
Retrieves the ith sent message
Pack
m
void removeSentMessage(int i)
Remove ith sent message
void removeMessage(int i)
Remove ith inbox message
Mailbox List Class
Method (Option)
Function
static Mailbox getUserMailbox
Returns Mailbox by user
E Maven
![Chrome
File
Edit
View
History
Bookmarks
People
Tab
Window
Help
88% O
Sun 8:11 PM
M SkinnyFit Order Cor
S delaGATE - Delawar X
O Project 2: Email Clie x
A Read Simple CSV Fi x
b Answered: The two x
O (2) Navigation in Int x
+
A canvas.dccc.edu/courses/27620/assignments/513560
Scree
2020-10
Message Class
tls
PM
Method (Option)
Function
Append a line to the messageText. If the message already contains
text, add a newline then add the line to be appended.
Scree
2020-10
void append(String line)
Formats a string contained a summary of the message suitable for
display in the inbox or sent message box.
Scree
2020-10
public String getMessageHeader() {
SimpleDateFormat sdf = new SimpleDateFormat("\t\TEEE MM dd");
String
return sender + "\nSubject: "
+ subject +
" " + sdf.format(d
getMessageTextHeader()
ate) +
"\n" +
messageText.split("\\n")[0] + "\n";
Arrays and
}
Pack
m
Creates a formatted string contained the To, From. Subject, Date, and
toString()
Message Text of a Message.
print()
Sends the toString() results to the display
send(sender, recipient,
Creates a Message including sender, recipient, subject, and date.
MessageText is added with append method.
subject)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc3828c53-0090-4baf-b1c0-74b20bcce24c%2F888b0056-45c0-4ecf-8293-fbbaa26529ab%2Ftxqgeya_processed.png&w=3840&q=75)
Transcribed Image Text:Chrome
File
Edit
View
History
Bookmarks
People
Tab
Window
Help
88% O
Sun 8:11 PM
M SkinnyFit Order Cor
S delaGATE - Delawar X
O Project 2: Email Clie x
A Read Simple CSV Fi x
b Answered: The two x
O (2) Navigation in Int x
+
A canvas.dccc.edu/courses/27620/assignments/513560
Scree
2020-10
Message Class
tls
PM
Method (Option)
Function
Append a line to the messageText. If the message already contains
text, add a newline then add the line to be appended.
Scree
2020-10
void append(String line)
Formats a string contained a summary of the message suitable for
display in the inbox or sent message box.
Scree
2020-10
public String getMessageHeader() {
SimpleDateFormat sdf = new SimpleDateFormat("\t\TEEE MM dd");
String
return sender + "\nSubject: "
+ subject +
" " + sdf.format(d
getMessageTextHeader()
ate) +
"\n" +
messageText.split("\\n")[0] + "\n";
Arrays and
}
Pack
m
Creates a formatted string contained the To, From. Subject, Date, and
toString()
Message Text of a Message.
print()
Sends the toString() results to the display
send(sender, recipient,
Creates a Message including sender, recipient, subject, and date.
MessageText is added with append method.
subject)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
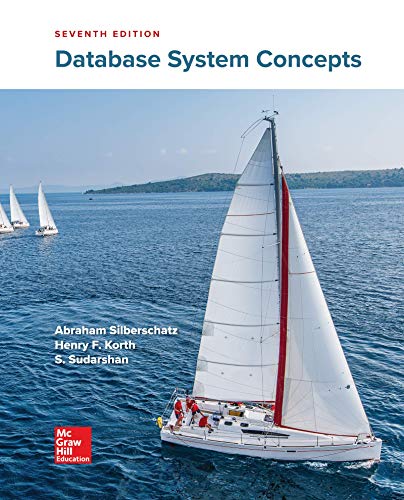
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
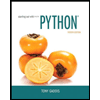
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
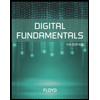
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
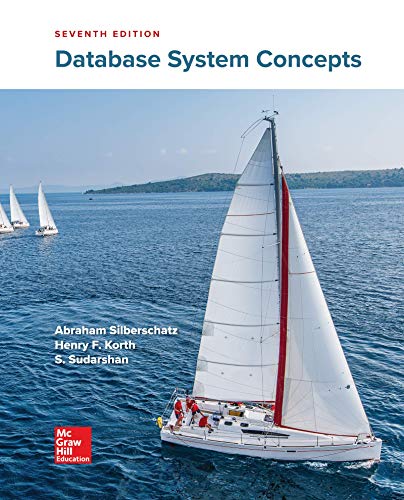
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
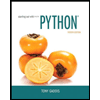
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
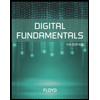
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
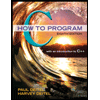
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
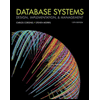
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
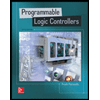
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education