(After reading the instructions given in the pictures attached, read the following) Class members doctorType Class must contain at least these functions doctorType(string first, string last, string spl); //First Name, Last Name, Specialty void print() const; //Formatted Display First Name, Last Name, Specialty void setSpeciality(string); //Set the doctor’s Specialty string getSpeciality(); //Return the doctor’s Specialty patientType Class must contain at least these functions void setInfo( string id, string fName, string lName, int bDay, int bMth, int bYear, string docFrName, string docLaName, string docSpl, int admDay, int admMth, int admYear, int disChDay, int disChMth, int disChYear); void setID(string); string getID(); void setBirthDate(int dy, int mo, int yr); int getBirthDay(); int getBirthMonth(); int getBirthYear(); void setDoctorName(string fName, string lName); void setDoctorSpl(string); string getDoctorFName(); string getDoctorLName(); string getDoctorSpl(); void setAdmDate(int dy, int mo, int yr); int getAdmDay(); int getAdmMonth(); int getAdmYear(); void setDisDate(int dy, int mo, int yr); int getDisDay(); int getDisMonth(); int getDisYear(); billType Class must contain at least these functions billType(string id = "", //Patient ID double phCharges = 0, //Pharmacy Charges double rRent = 0, //Room rental double docFee = 0); //Doctor Fees void printBill() const; void setInfo(string, //Patient ID double, //Pharmacy Charges double, //Room rental double); //Doctor Fees void setID(string); string getID(); void setPharmacyCharges(double); double getPharmacyCharges(); void updatePharmacyCharges(double); void setRoomRent(double); double getRoomRent(); void updateRoomRent(double); void setDoctorFee(double); double getDoctorFee(); void updateDoctorFee(double); double billingAmount(); The instructors test program will construct arrays of 10 doctorType, 10 patientType and 20 billType instances and from this data will produce the report shown on the next page. All patients will have 1 or more billings. Make sure you validate your three classes against all the functions shown above. The instructor output will be billings by patient and will provide summary data similar to the screen shot on the next page. If you need help with using this for your testing contact the instructor. Please follow the instructions given above and ensure that all of the classes and functions are mentioned in the code. Also, show how the output looks with a screenshot of your output program. Someone already "answered" this problem on Chegg before but they did a really poor job at it. Thank you so much.
(After reading the instructions given in the pictures attached, read the following) Class members doctorType Class must contain at least these functions doctorType(string first, string last, string spl); //First Name, Last Name, Specialty void print() const; //Formatted Display First Name, Last Name, Specialty void setSpeciality(string); //Set the doctor’s Specialty string getSpeciality(); //Return the doctor’s Specialty patientType Class must contain at least these functions void setInfo( string id, string fName, string lName, int bDay, int bMth, int bYear, string docFrName, string docLaName, string docSpl, int admDay, int admMth, int admYear, int disChDay, int disChMth, int disChYear); void setID(string); string getID(); void setBirthDate(int dy, int mo, int yr); int getBirthDay(); int getBirthMonth(); int getBirthYear(); void setDoctorName(string fName, string lName); void setDoctorSpl(string); string getDoctorFName(); string getDoctorLName(); string getDoctorSpl(); void setAdmDate(int dy, int mo, int yr); int getAdmDay(); int getAdmMonth(); int getAdmYear(); void setDisDate(int dy, int mo, int yr); int getDisDay(); int getDisMonth(); int getDisYear(); billType Class must contain at least these functions billType(string id = "", //Patient ID double phCharges = 0, //Pharmacy Charges double rRent = 0, //Room rental double docFee = 0); //Doctor Fees void printBill() const; void setInfo(string, //Patient ID double, //Pharmacy Charges double, //Room rental double); //Doctor Fees void setID(string); string getID(); void setPharmacyCharges(double); double getPharmacyCharges(); void updatePharmacyCharges(double); void setRoomRent(double); double getRoomRent(); void updateRoomRent(double); void setDoctorFee(double); double getDoctorFee(); void updateDoctorFee(double); double billingAmount(); The instructors test program will construct arrays of 10 doctorType, 10 patientType and 20 billType instances and from this data will produce the report shown on the next page. All patients will have 1 or more billings. Make sure you validate your three classes against all the functions shown above. The instructor output will be billings by patient and will provide summary data similar to the screen shot on the next page. If you need help with using this for your testing contact the instructor. Please follow the instructions given above and ensure that all of the classes and functions are mentioned in the code. Also, show how the output looks with a screenshot of your output program. Someone already "answered" this problem on Chegg before but they did a really poor job at it. Thank you so much.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
(After reading the instructions given in the pictures attached, read the following)
Class members
doctorType Class must contain at least these functions
doctorType(string first, string last, string spl); //First Name, Last Name, Specialty
doctorType Class must contain at least these functions
doctorType(string first, string last, string spl); //First Name, Last Name, Specialty
void print() const; //Formatted Display First Name, Last Name, Specialty
void setSpeciality(string); //Set the doctor’s Specialty
string getSpeciality(); //Return the doctor’s Specialty
patientType Class must contain at least these functions
void setInfo(
string id, string fName, string lName,
int bDay, int bMth, int bYear,
string docFrName, string docLaName, string docSpl,
int admDay, int admMth, int admYear,
int disChDay, int disChMth, int disChYear);
void setID(string);
string getID();
void setBirthDate(int dy, int mo, int yr);
int getBirthDay();
int getBirthMonth();
int getBirthYear();
void setDoctorName(string fName, string lName);
void setDoctorSpl(string);
string getDoctorFName();
string getDoctorLName();
string getDoctorSpl();
void setAdmDate(int dy, int mo, int yr);
int getAdmDay();
int getAdmMonth();
int getAdmYear();
void setDisDate(int dy, int mo, int yr);
void setSpeciality(string); //Set the doctor’s Specialty
string getSpeciality(); //Return the doctor’s Specialty
patientType Class must contain at least these functions
void setInfo(
string id, string fName, string lName,
int bDay, int bMth, int bYear,
string docFrName, string docLaName, string docSpl,
int admDay, int admMth, int admYear,
int disChDay, int disChMth, int disChYear);
void setID(string);
string getID();
void setBirthDate(int dy, int mo, int yr);
int getBirthDay();
int getBirthMonth();
int getBirthYear();
void setDoctorName(string fName, string lName);
void setDoctorSpl(string);
string getDoctorFName();
string getDoctorLName();
string getDoctorSpl();
void setAdmDate(int dy, int mo, int yr);
int getAdmDay();
int getAdmMonth();
int getAdmYear();
void setDisDate(int dy, int mo, int yr);
int getDisDay();
int getDisMonth();
int getDisYear();
int getDisMonth();
int getDisYear();
billType Class must contain at least these functions
billType(string id = "", //Patient ID
double phCharges = 0, //Pharmacy Charges
double rRent = 0, //Room rental
double docFee = 0); //Doctor Fees
void printBill() const;
void setInfo(string, //Patient ID
double, //Pharmacy Charges
double, //Room rental
double); //Doctor Fees
void setID(string);
string getID();
void setPharmacyCharges(double);
double getPharmacyCharges();
void updatePharmacyCharges(double);
void setRoomRent(double);
double getRoomRent();
void updateRoomRent(double);
void setDoctorFee(double);
double getDoctorFee();
void updateDoctorFee(double);
double billingAmount();
The instructors test program will construct arrays of 10 doctorType, 10 patientType and 20 billType
instances and from this data will produce the report shown on the next page. All patients will have 1 or more billings.
Make sure you validate your three classes against all the functions shown above. The instructor output will be billings
by patient and will provide summary data similar to the screen shot on the next page. If you need help with using this
for your testing contact the instructor.
billType(string id = "", //Patient ID
double phCharges = 0, //Pharmacy Charges
double rRent = 0, //Room rental
double docFee = 0); //Doctor Fees
void printBill() const;
void setInfo(string, //Patient ID
double, //Pharmacy Charges
double, //Room rental
double); //Doctor Fees
void setID(string);
string getID();
void setPharmacyCharges(double);
double getPharmacyCharges();
void updatePharmacyCharges(double);
void setRoomRent(double);
double getRoomRent();
void updateRoomRent(double);
void setDoctorFee(double);
double getDoctorFee();
void updateDoctorFee(double);
double billingAmount();
The instructors test program will construct arrays of 10 doctorType, 10 patientType and 20 billType
instances and from this data will produce the report shown on the next page. All patients will have 1 or more billings.
Make sure you validate your three classes against all the functions shown above. The instructor output will be billings
by patient and will provide summary data similar to the screen shot on the next page. If you need help with using this
for your testing contact the instructor.
Please follow the instructions given above and ensure that all of the classes and functions are mentioned in the code. Also, show how the output looks with a screenshot of your output program. Someone already "answered" this problem on Chegg before but they did a really poor job at it.
Thank you so much.

Transcribed Image Text:Exercise L1-2 Chapter 11 Inheritance & Composition
This exercise is designed so each patient can have multiple doctors, and multiple bills. The details are listed below:
Design the class doctorType, inherited from the class personType, given below. Add a data member to store
the doctors specialty. Also add appropriate constructors and member functions to initialize, access, and
manipulate the data members.
A.
#pragma once
#include <string>
using namespace std;
class personType
{
public:
void print() const;
//Function to output the first name and last name
//in the form firstName lastName.
}
void setName(string first, string last);
//Function to set firstName and lastName according
//to the parameters.
//Postcondition: firstName = first; lastName = last
string getFirstName() const;
//Function to return the first name.
//Postcondition: The value of the data member firstName
11
is returned.
}
string getLastName() const;
//Function to return the last name.
//Postcondition: The value of the data member lastName
is returned.
personType(string first = **, string last = "");
//constructor
private:
string firstName; //variable to store the first name
string lastName; //variable to store the last name
//Sets firstName and lastName according to the parameters.
//The default values of the parameters are empty strings.
//Postcondition: firstName = first; lastName = last
};
void personType::print() const
{
}
void personType::setName(string first, string last)
{
cout <<< firstName << " " << lastName;
firstName= first;
lastName = last;
string personType::getFirstName() const
return firstName;
string personType::getLastName() const
{
return lastName;
}
//constructor
personType: :personType(string first, string last)

Transcribed Image Text:B.
C.
D.
{
firstName = first;
lastName = last;
}
Designed the class billType with data members to store a patients ID and a patient's hospital charges, such
as pharmacy charges for medicine, Doctors fee, and room charges. Add appropriate constructors and member
functions to initialize, access, and manipulate the data members.
Design the class patientType, inherited from the class personType, with additional data members to
store the patients ID, the age, date of birth, attending Physicians name, the date when the patient was
admitted in the hospital, and the date when the patient was discharged from the hospital. (use the class
dateType to store the date of birth, admit date, discharge date, and the class doctorType to store to be
attending Physicians name.) Add appropriate constructors and member functions to initialize, access, and
manipulate the data members.
Write a program to test your classes. You can check your class definitions by using L1-2CmplTest.cpp found in
the L1-2 folder.
Your output should look like this
G:\Coursework\CIS 23 - Data Structures and Algorithme Solutions Lab1\L1-2\Debug>L1-2.exe
John Urrutia
L1-2
L1-2.exe
Enter patient's ID: 123456
Enter patient's first name: Joe
Enter patient's last name: Blowinski
Enter doctor's first name: Rob
Enter doctor's last name: Ublind
Enter doctor's speciality: Paychiatry
Patient: Joe Blowinski
Attending Physician: Rob Ublind Paychiatry
Admit Date: 4-15-2009
Discharge Date: 4-21-2009
Pharmacy Charges: $245.50
Room Charges: $2500.00
Doctor's Fees: $3500.38
Total Chargee: $6245.88
Press any key to continue...
Class members
doctorType Class must contain at least these functions
doctorType(string first, string last, string spl); //First Name, Last Name, Specialty
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 13 images

Recommended textbooks for you
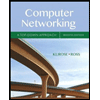
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
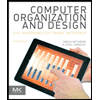
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
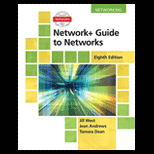
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
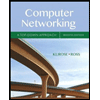
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
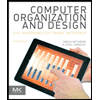
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
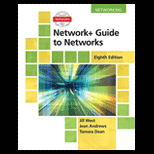
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
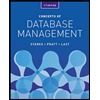
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
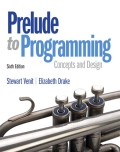
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
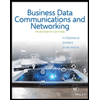
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY