classification_nn
.pdf
keyboard_arrow_up
School
University of California, San Diego *
*We aren’t endorsed by this school
Course
176
Subject
Statistics
Date
May 14, 2024
Type
Pages
25
Uploaded by MasterJellyfish4274 on coursehero.com
classification_nn
February 16, 2024
1
ECE 176 Assignment 4: Classification using Neural Network
Now that you have developed and tested your model on the toy dataset set. It’s time to get down
and get dirty with a standard dataset such as cifar10. At this point, you will be using the provided
training data to tune the hyper-parameters of your network such that it works with cifar10 for the
task of multi-class classification.
Important: Recall that now we have non-linear decision boundaries, thus we do not need to do
one vs all classification. We learn a single non-linear decision boundary instead. Our non-linear
boundaries (thanks to relu non-linearity) will take care of differentiating between all the classes
TO SUBMIT: PDF of this notebook with all the required outputs and answers.
[2]:
# Prepare Packages
import
numpy
as
np
import
matplotlib.pyplot
as
plt
from
utils.data_processing
import
get_cifar10_data
from
utils.evaluation
import
get_classification_accuracy
%
matplotlib
inline
plt
.
rcParams[
"figure.figsize"
]
=
(
10.0
,
8.0
)
# set default size of plots
# For auto-reloading external modules
# See http://stackoverflow.com/questions/1907993/
↪
autoreload-of-modules-in-ipython
%
load_ext
autoreload
%
autoreload
2
# Use a subset of CIFAR10 for the assignment
dataset
=
get_cifar10_data(
subset_train
=5000
,
subset_val
=250
,
subset_test
=500
,
)
print
(dataset
.
keys())
print
(
"Training Set Data
Shape: "
, dataset[
"x_train"
]
.
shape)
1
print
(
"Training Set Label Shape: "
, dataset[
"y_train"
]
.
shape)
print
(
"Validation Set Data
Shape: "
, dataset[
"x_val"
]
.
shape)
print
(
"Validation Set Label Shape: "
, dataset[
"y_val"
]
.
shape)
print
(
"Test Set Data
Shape: "
, dataset[
"x_test"
]
.
shape)
print
(
"Test Set Label Shape: "
, dataset[
"y_test"
]
.
shape)
dict_keys(['x_train', 'y_train', 'x_val', 'y_val', 'x_test', 'y_test'])
Training Set Data
Shape:
(5000, 3072)
Training Set Label Shape:
(5000,)
Validation Set Data
Shape:
(250, 3072)
Validation Set Label Shape:
(250,)
Test Set Data
Shape:
(500, 3072)
Test Set Label Shape:
(500,)
[3]:
x_train
=
dataset[
"x_train"
]
y_train
=
dataset[
"y_train"
]
x_val
=
dataset[
"x_val"
]
y_val
=
dataset[
"y_val"
]
x_test
=
dataset[
"x_test"
]
y_test
=
dataset[
"y_test"
]
[4]:
# Import more utilies and the layers you have implemented
from
layers.sequential
import
Sequential
from
layers.linear
import
Linear
from
layers.relu
import
ReLU
from
layers.softmax
import
Softmax
from
layers.loss_func
import
CrossEntropyLoss
from
utils.optimizer
import
SGD
from
utils.dataset
import
DataLoader
from
utils.trainer
import
Trainer
1.1
Visualize some examples from the dataset.
[5]:
# We show a few examples of training images from each class.
classes
=
[
"airplane"
,
"automobile"
,
"bird"
,
"cat"
,
"deer"
,
"dog"
,
"frog"
,
"horse"
,
"ship"
,
]
samples_per_class
= 7
2
def
visualize_data
(dataset, classes, samples_per_class):
num_classes
=
len
(classes)
for
y,
cls
in
enumerate
(classes):
idxs
=
np
.
flatnonzero(y_train
==
y)
idxs
=
np
.
random
.
choice(idxs, samples_per_class, replace
=
False
)
for
i, idx
in
enumerate
(idxs):
plt_idx
=
i
*
num_classes
+
y
+ 1
plt
.
subplot(samples_per_class, num_classes, plt_idx)
plt
.
imshow(dataset[idx])
plt
.
axis(
"off"
)
if
i
== 0
:
plt
.
title(
cls
)
plt
.
show()
# Visualize the first 10 classes
visualize_data(
x_train
.
reshape(
5000
,
3
,
32
,
32
)
.
transpose(
0
,
2
,
3
,
1
),
classes,
samples_per_class,
)
3
1.2
Initialize the model
[6]:
input_size
= 3072
hidden_size
= 100
# Hidden layer size (Hyper-parameter)
num_classes
= 10
# Output
# For a default setting we use the same model we used for the toy dataset.
# This tells you the power of a 2 layered Neural Network. Recall the Universal
␣
↪
Approximation Theorem.
# A 2 layer neural network with non-linearities can approximate any function,
␣
↪
given large enough hidden layer
def
init_model
():
# np.random.seed(0) # No need to fix the seed here
l1
=
Linear(input_size, hidden_size)
l2
=
Linear(hidden_size, num_classes)
r1
=
ReLU()
softmax
=
Softmax()
4
return
Sequential([l1, r1, l2, softmax])
[7]:
# Initialize the dataset with the dataloader class
dataset
=
DataLoader(x_train, y_train, x_val, y_val, x_test, y_test)
net
=
init_model()
optim
=
SGD(net, lr
=0.01
, weight_decay
=0.01
)
loss_func
=
CrossEntropyLoss()
epoch
= 200
# (Hyper-parameter)
batch_size
= 200
# (Reduce the batch size if your computer is unable to handle
␣
↪
it)
[8]:
# Initialize the trainer class by passing the above modules
trainer
=
Trainer(
dataset, optim, net, loss_func, epoch, batch_size, validate_interval
=3
)
[9]:
# Call the trainer function we have already implemented for you. This trains
␣
↪
the model for the given
# hyper-parameters. It follows the same procedure as in the last ipython
␣
↪
notebook you used for the toy-dataset
train_error, validation_accuracy
=
trainer
.
train()
Epoch Average Loss: 2.302534
Validate Acc: 0.084
Epoch Average Loss: 2.302361
Epoch Average Loss: 2.302147
Epoch Average Loss: 2.301862
Validate Acc: 0.108
Epoch Average Loss: 2.301437
Epoch Average Loss: 2.300839
Epoch Average Loss: 2.299990
Validate Acc: 0.096
Epoch Average Loss: 2.298824
Epoch Average Loss: 2.297339
Epoch Average Loss: 2.295516
Validate Acc: 0.084
Epoch Average Loss: 2.293398
Epoch Average Loss: 2.290907
Epoch Average Loss: 2.287820
Validate Acc: 0.084
Epoch Average Loss: 2.284062
Epoch Average Loss: 2.278987
Epoch Average Loss: 2.272846
Validate Acc: 0.096
Epoch Average Loss: 2.265936
Epoch Average Loss: 2.258480
Epoch Average Loss: 2.250872
5
Validate Acc: 0.100
Epoch Average Loss: 2.243156
Epoch Average Loss: 2.235648
Epoch Average Loss: 2.228603
Validate Acc: 0.124
Epoch Average Loss: 2.221921
Epoch Average Loss: 2.215911
Epoch Average Loss: 2.210334
Validate Acc: 0.124
Epoch Average Loss: 2.204742
Epoch Average Loss: 2.200037
Epoch Average Loss: 2.195513
Validate Acc: 0.128
Epoch Average Loss: 2.191241
Epoch Average Loss: 2.187065
Epoch Average Loss: 2.183359
Validate Acc: 0.132
Epoch Average Loss: 2.179895
Epoch Average Loss: 2.176374
Epoch Average Loss: 2.173382
Validate Acc: 0.144
Epoch Average Loss: 2.170088
Epoch Average Loss: 2.167192
Epoch Average Loss: 2.164379
Validate Acc: 0.140
Epoch Average Loss: 2.161388
Epoch Average Loss: 2.159176
Epoch Average Loss: 2.156676
Validate Acc: 0.144
Epoch Average Loss: 2.154465
Epoch Average Loss: 2.152342
Epoch Average Loss: 2.149955
Validate Acc: 0.144
Epoch Average Loss: 2.148316
Epoch Average Loss: 2.145852
Epoch Average Loss: 2.144280
Validate Acc: 0.148
Epoch Average Loss: 2.142039
Epoch Average Loss: 2.140363
Epoch Average Loss: 2.138541
Validate Acc: 0.152
Epoch Average Loss: 2.136884
Epoch Average Loss: 2.135169
Epoch Average Loss: 2.133874
Validate Acc: 0.148
Epoch Average Loss: 2.132104
Epoch Average Loss: 2.130449
Epoch Average Loss: 2.129021
6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Why won't the whiteboard image show up?
arrow_forward
"ple: Find the linear least squares approximation to the data:
- 1
0.5
Yi
2
4
arrow_forward
The Ghana health service requested for a machine learning model to detect the presence of
a new disease based on symptoms present in a blood sample. You have been selected to be
part of the team responsible for the development of the model. Belo is a block diagram of
the system.
Inputs
Processing
Output
The accuracy in the output depends on the correct inputs. The model designed is supposed
to be evaluated to determine the accuracy and it is supposed to function in a supervised
way. Hence the data sets can affect the model and vice versa.
Given
P(Data|Model) =
P(Model|Data)P(Data) or P(D|M)
P(M|D)P(D)
show that the
p(Model)
p(M)
following statements are true with a formal mathematical proof.
Show that
P(M|D)[P(D|M')(1-P(M))+(1-P(D|M)P(M))]
а. Р(DIM)
1-р(М)
b. Р(D) — P(D|M')(1 — Р(M)) + (1— Р(D'/M)Р(М) )
arrow_forward
Why is it difficult to describe how model validation is done?
arrow_forward
The Ghana health service requested for a machine learning model to detect the presence of
a new disease based on symptoms present in a blood sample. You have been selected to be
part of the team responsible for the development of the model. Belo is a block diagram of
the system.
Inputs
Processing
Output
The accuracy in the output depends on the correct inputs. The model designed is supposed
to be evaluated to determine the accuracy and it is supposed to function in a supervised
way. Hence the data sets can affect the model and vice versa.
Given
P(Data|Model) = P(Model|Data)P(Data)
or P(D|M) =
P(M|D)P(D)
show that the
P(Model)
p(M)
following statements are true with a formal mathematical proof.
Show that
a. P(D|M) = P(M|D)IP(D|M')(1-P(M))+(1-P(D|M)P(M))]
1-p(M")
b. P(D) = P(D|M')(1– P(M)) + (1– P(D'/M)P(M))
Question 2 (50 marks) 300-500 words per discussion and avoid plagiarism.
From the question 1, given P(D|M) = P(MD)P(D) solve the following
a. Draw the probability tree for the…
arrow_forward
Explain the Formula for the Two Stage Least Squares estimator?
arrow_forward
geBoard
Consumable Student Editic
Name: tyr poPrsrey Class:
Date:
Version: J
Algebra I EOC Review #3 WS Due 4-15-21(ALL WORK MUST BE SHOWN) In-person must
get approved by teacher before submitting in Classroom. Online may submit, then resubmit onc
Must show all work or explain steps(in detail) to receive any credit.
1. The data set shows the amount of funds raised and the number of participants in the fundraiser at the Family
House organization branches. Use a graphing calculator to find and graph an equation of the least-squares line
the data.(Linear Regression)
Family House Fundraiser
# of participants
Funds raised (S)
10
15
20
25
13
15
18
490 500 | 550 570 630 520 550 560
Yünu'a fomihy is staving at a campground th
arrow_forward
Clocking the Cheetah. The cheetah (Acinonyx jubatus) is the fastest land mammal and is highly specialized to run down prey. The cheetah often exceeds speeds of 60 mph and, according to the online document “Cheetah Conservation in Southern Africa” (Trade&Environment Database (TED) Case Studies, Vol. 8, No. 2) by J. Urbaniak, the cheetah is capable of speeds up to 72 mph. Following is a frequency histogram for the speeds, in miles per hour, for a sample of 35 cheetahs.
arrow_forward
The Ministry of Tourism in Trinidad and Tobago is interested in developing a campaign to increase the number of visitors to the island. The Ministry in collaboration with the island’s hotels collected data to be used as a guide to determine what steps should be taken going forward. Using the data in the Microsoft Excel file attached you are required to use the knowledge you have acquired during the semester to answer the following question. Ensure that your responses are detailed and all the necessary steps are clearly outlined.
Derive a model for the estimation of the probability of returning to the island from the average money spent during the visit.
Discuss why regression analysis is important in decision-making.
arrow_forward
If the Cumulative Gain at a depth of 15% for the Neural Network model is converted to number of primary/positive event cases, what will be the number of cases? Show your calculation.
arrow_forward
I want to explain these outputs in detail
arrow_forward
Reading is fundamental to a teenager's ability to perform well in school. Assume a researcher is
interested in the ability of the number of books read over the summer to predict ACT Reading
scores. Using a random sample of 10 random high school students the researchers recorded the
number of books read over the summer and the students' ACT Reading scores.
Books Read (X) ACT Score (Y) (X- Xmean)
(Y - Ymean)
(X-Xmean)(Y-Ymean) (X-Xmean)?
(Y - Ymean)?
2
16
-5.4
-14.9
81
29
221
2
19
-5.4
-11.9
64
29
141
3
17
-4.4
-13.9
61
20
192
4
25
-3.4
-5.9
20
12
34
21
-3.4
-9.9
34
12
97
24
-1.4
-6.9
10
2
47
6
21
-1.4
-9.9
14
2
97
8
24
0.6
-6.9
-4
47
7
27
-0.4
-3.9
2
15
10
22
2.6
-8.9
-23
7
78
Total
52
216
259.1
113.3
969.3
МEAN
7.4
30.9
a. Identify the regression line using the number of books read to predict ACT Reading
score. Use a = .05 to evaluate the quality of the prediction of the regression line.
b. What is the predicted ACT Reading score when 5 books are read?
arrow_forward
Content
B PowerPoint Presentation
b Answered: 1. Use Pappus' Theore X
Messenger
learn-ap-southeast-1-prod-fleet02-xythos.content.blackboardcdn.com/5be3c7fe2b7ic/198138777X-Blackboard-Expiration=16491276000008X-Blackboard-Si. e *
Classroom M Gmail
PowerPoint Presentation
7 / 14
100%
+
Exercises:
Sketch the region bounded by the given curves, then find the centroid of its
area.
4x
5. x = 8 – y²,
x = y2 – 8
II
arrow_forward
Content
B PowerPoint Presentation
b Answered: 1. Use Pappus' Theore X
Messenger
learn-ap-southeast-1-prod-fleet02-xythos.content.blackboardcdn.com/5be3c7fe2b7ic/198138777X-Blackboard-Expiration=16491276000008X-Blackboard-Si. e *
Classroom M Gmail
PowerPoint Presentation
7 / 14
100%
+
Exercises:
Sketch the region bounded by the given curves, then find the centroid of its
area.
1. y = x2, y = 2x
5. X
6. y = x2 – 3x,
y = x
II
arrow_forward
Clocking the Cheetah. The cheetah (Acinonyx jubatus)isthe fastest land mammal and is highly specialized to run down prey. The cheetah often exceeds speeds of 60 mph and, according to the online document “Cheetah Conservation in Southern Africa” (Trade&Envi-ronment Database (TED) Case Studies, Vol. 8, No. 2) by J. Urbaniak, the cheetah is capable of speeds up to 72 mph. The WeissStats site contains the top speeds, in miles per hour, for a sample of 35 chee-tahs. Use the technology of your choice to do the following tasks. a. Find a 95% confidence interval for the mean top speed, μ,ofall cheetahs. Assume that the population standard deviation of top speeds is 3.2 mph. d. Comment on the advisability of using the z-interval procedure on these data.
arrow_forward
A new product made from recycled plastic soda bottles needs 18
labor hours to build the first prototype. The production operates at
a 86.5 % learning curve rate. The average time to complete the first
five prototypes is
hours.
arrow_forward
I want to explain these outputs ?
arrow_forward
Calculate least squares regression using this information
arrow_forward
Please give maximum value for this data!
arrow_forward
Apply the linear least-squares method to each set of data to obtain a mathematical model showing the relationships between variables.
arrow_forward
Home - myTNCC
D WebAssign Login Page
A Test 2 Chapters 4-5 - MTH 261 B X
W Project 2 - MTH 261 BO6H, sectic x
i webassign.net/web/Student/Assignment-Responses/submit?dep323085263&tags=autosave#question4608232 2
6.
LARAPCALC10 4.6.024.
The graph shows the populations (in millions) of Bulgaria and Tajikistan from 2006 through 2013 using exponential models.t
8.
7.75
Tajikistan
7.5
7.25
Bulgaria
6.75
6.5
7.
9 10 11 12 13
Year (6 + 2006)
(a) Determine whether the population of Tajikistan is modeled by exponential growth or exponential decay. Explain your reasoning.
The population of Tajikistan is decreasing; therefore, it must be an exponential decay model.
O The population of Tajikistan is increasing; therefore, it must be an exponential growth model.
O The population of Tajikistan is decreasing; therefore, it must be an exponential growth model.
The population of Tajikistan is increasing; therefore, it must be an exponential decay model.
Determine whether the population of Bulgaria is…
arrow_forward
Explain the Two Stage Least Squares Estimator?
arrow_forward
What minimization is a basic technique in linear regression of learning models? How do you get the minimum? Please explain.
arrow_forward
a.State the predictors available in this model.
arrow_forward
Find the least-squares line for the given data(2,1),(3,2),(4,3),(5,2)
Follow the solution format in the given example picture
arrow_forward
Consider a model with an interaction term between being female and being married. The dependent variable is the log of the
hourly wage:
log(wage) = 0.151 - 0.038 female + 0.1 married - 0.301 female* married + 0.079 educ + 0.027 exper+0.029 tenure
(0.072)
(0.056)
(0.055)
(0.007)
(0.005)
(0.007)
n = 536, R2 = 0.461
Numbers in parantheses are standard errors of coefficients. Given the estimation result and the observation number fill in the
blanks below which aim at discussing the statistical significance of variables.
The test statistic of the interaction term is
The critical value at 1% significance level is
Then the interaction term
statistically significant at 1% significance level. (Hint: to fill the blank
make a choice between "is" and "is not".)
arrow_forward
eSkill
Subject: MS Office 2019- Exc
Topic: Common Functions
Talent Assessment Platform
Question: #358754
» QUESTION: 15 OF 20
Instructions
Please report any problems with this qu
Suppose you have an Excel file, and in Column A there are more than 10,000 entries. Those entries are either text or
numbers. Which of the following functions will help you to determine the number of cells in Column A that contain only
numbers and not text?
Select the single best answer:
O A. HLOOKUP
B. COUNTA
C. ISNUMBER
D. RANDBETWEEN
E. COUNT
« Previous
Question
Next Question >>
arrow_forward
Augmented Reality (AR) is a technology that superimposes a computer-generated image on a user's view of the real world, and it is believed that it will soon affect the conventional learning process. A random sample of nine university students was selected to test for the effectiveness of a special course on augmented reality in learning. They were taught how to incorporate AR into their learning process to its full potential. Table 1 gives the scores in a Learning Effectiveness test given to these students before and after attending this course.
Construct a 95% confidence interval for the mean (mean difference) of the population paired differences.
arrow_forward
Augmented Reality (AR) is a technology that superimposes a computer-generated image on a user's view of the real world, and it is believed that it will soon affect the conventional learning process. A random sample of nine university students was selected to test for the effectiveness of a special course on augmented reality in learning. They were taught how to incorporate AR into their learning process to its full potential. Table 1 gives the scores in a Learning Effectiveness test given to these students before and after attending this course.
(a) Construct a 95% confidence interval for the mean ?? of the population paired differences.
arrow_forward
Augmented Reality (AR) is a technology that superimposes a computer-generated image on a user's view of the real world, and it is believed that it will soon affect the conventional learning process. A random sample of nine university students was selected to test for the effectiveness of a special course on augmented reality in learning. They were taught how to incorporate AR into their learning process to its full potential. Table 1 gives the scores in a Learning Effectiveness test given to these students before and after attending this course.
Question: Test at the 1% significance level whether this AR course makes any statistically significant improvement in the learning effectiveness of all university students.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
Algebra & Trigonometry with Analytic Geometry
Algebra
ISBN:9781133382119
Author:Swokowski
Publisher:Cengage
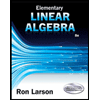
Elementary Linear Algebra (MindTap Course List)
Algebra
ISBN:9781305658004
Author:Ron Larson
Publisher:Cengage Learning
Related Questions
- Why won't the whiteboard image show up?arrow_forward"ple: Find the linear least squares approximation to the data: - 1 0.5 Yi 2 4arrow_forwardThe Ghana health service requested for a machine learning model to detect the presence of a new disease based on symptoms present in a blood sample. You have been selected to be part of the team responsible for the development of the model. Belo is a block diagram of the system. Inputs Processing Output The accuracy in the output depends on the correct inputs. The model designed is supposed to be evaluated to determine the accuracy and it is supposed to function in a supervised way. Hence the data sets can affect the model and vice versa. Given P(Data|Model) = P(Model|Data)P(Data) or P(D|M) P(M|D)P(D) show that the p(Model) p(M) following statements are true with a formal mathematical proof. Show that P(M|D)[P(D|M')(1-P(M))+(1-P(D|M)P(M))] а. Р(DIM) 1-р(М) b. Р(D) — P(D|M')(1 — Р(M)) + (1— Р(D'/M)Р(М) )arrow_forward
- Why is it difficult to describe how model validation is done?arrow_forwardThe Ghana health service requested for a machine learning model to detect the presence of a new disease based on symptoms present in a blood sample. You have been selected to be part of the team responsible for the development of the model. Belo is a block diagram of the system. Inputs Processing Output The accuracy in the output depends on the correct inputs. The model designed is supposed to be evaluated to determine the accuracy and it is supposed to function in a supervised way. Hence the data sets can affect the model and vice versa. Given P(Data|Model) = P(Model|Data)P(Data) or P(D|M) = P(M|D)P(D) show that the P(Model) p(M) following statements are true with a formal mathematical proof. Show that a. P(D|M) = P(M|D)IP(D|M')(1-P(M))+(1-P(D|M)P(M))] 1-p(M") b. P(D) = P(D|M')(1– P(M)) + (1– P(D'/M)P(M)) Question 2 (50 marks) 300-500 words per discussion and avoid plagiarism. From the question 1, given P(D|M) = P(MD)P(D) solve the following a. Draw the probability tree for the…arrow_forwardExplain the Formula for the Two Stage Least Squares estimator?arrow_forward
- geBoard Consumable Student Editic Name: tyr poPrsrey Class: Date: Version: J Algebra I EOC Review #3 WS Due 4-15-21(ALL WORK MUST BE SHOWN) In-person must get approved by teacher before submitting in Classroom. Online may submit, then resubmit onc Must show all work or explain steps(in detail) to receive any credit. 1. The data set shows the amount of funds raised and the number of participants in the fundraiser at the Family House organization branches. Use a graphing calculator to find and graph an equation of the least-squares line the data.(Linear Regression) Family House Fundraiser # of participants Funds raised (S) 10 15 20 25 13 15 18 490 500 | 550 570 630 520 550 560 Yünu'a fomihy is staving at a campground tharrow_forwardClocking the Cheetah. The cheetah (Acinonyx jubatus) is the fastest land mammal and is highly specialized to run down prey. The cheetah often exceeds speeds of 60 mph and, according to the online document “Cheetah Conservation in Southern Africa” (Trade&Environment Database (TED) Case Studies, Vol. 8, No. 2) by J. Urbaniak, the cheetah is capable of speeds up to 72 mph. Following is a frequency histogram for the speeds, in miles per hour, for a sample of 35 cheetahs.arrow_forwardThe Ministry of Tourism in Trinidad and Tobago is interested in developing a campaign to increase the number of visitors to the island. The Ministry in collaboration with the island’s hotels collected data to be used as a guide to determine what steps should be taken going forward. Using the data in the Microsoft Excel file attached you are required to use the knowledge you have acquired during the semester to answer the following question. Ensure that your responses are detailed and all the necessary steps are clearly outlined. Derive a model for the estimation of the probability of returning to the island from the average money spent during the visit. Discuss why regression analysis is important in decision-making.arrow_forward
- If the Cumulative Gain at a depth of 15% for the Neural Network model is converted to number of primary/positive event cases, what will be the number of cases? Show your calculation.arrow_forwardI want to explain these outputs in detailarrow_forwardReading is fundamental to a teenager's ability to perform well in school. Assume a researcher is interested in the ability of the number of books read over the summer to predict ACT Reading scores. Using a random sample of 10 random high school students the researchers recorded the number of books read over the summer and the students' ACT Reading scores. Books Read (X) ACT Score (Y) (X- Xmean) (Y - Ymean) (X-Xmean)(Y-Ymean) (X-Xmean)? (Y - Ymean)? 2 16 -5.4 -14.9 81 29 221 2 19 -5.4 -11.9 64 29 141 3 17 -4.4 -13.9 61 20 192 4 25 -3.4 -5.9 20 12 34 21 -3.4 -9.9 34 12 97 24 -1.4 -6.9 10 2 47 6 21 -1.4 -9.9 14 2 97 8 24 0.6 -6.9 -4 47 7 27 -0.4 -3.9 2 15 10 22 2.6 -8.9 -23 7 78 Total 52 216 259.1 113.3 969.3 МEAN 7.4 30.9 a. Identify the regression line using the number of books read to predict ACT Reading score. Use a = .05 to evaluate the quality of the prediction of the regression line. b. What is the predicted ACT Reading score when 5 books are read?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Algebra & Trigonometry with Analytic GeometryAlgebraISBN:9781133382119Author:SwokowskiPublisher:CengageElementary Linear Algebra (MindTap Course List)AlgebraISBN:9781305658004Author:Ron LarsonPublisher:Cengage Learning
Algebra & Trigonometry with Analytic Geometry
Algebra
ISBN:9781133382119
Author:Swokowski
Publisher:Cengage
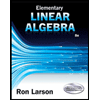
Elementary Linear Algebra (MindTap Course List)
Algebra
ISBN:9781305658004
Author:Ron Larson
Publisher:Cengage Learning