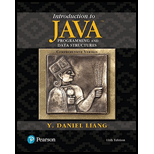
Concept explainers
(Assign grades) Write a
Grade is A if score is ≥ best −10;
Grade is B if score is ≥ best −20;
Grade is C if score is ≥ best −30;
Grade is D if score is ≥ best − 40;
Grade is F otherwise.
The program prompts the user to enter the total number of students, then prompts the user to enter all of the scores, and concludes by displaying the grades. Here is a sample run:

Assign Grades
Program Plan:
- Import required packages.
- Declare the main class method “Sample1”.
- In the main method.
- Create an object “input” for the scanner class.
- Get the number of students from the user and store it in a variable “numberOfStudents”.
- Create an object “scores” for the static method “double”.
- Read the corresponding student scores and find the best score.
- Declare and initialize the output string.
- Print the student score and grade.
- The grades are assigned based on the following scheme.
- Grade is A if score is >= best-10;
- Grade is B if score is >= best -20;
- Grade is C if score is >= best -30;
- Grade is D if score is >= best - 40;
- Grade is F otherwise.
- In the main method.
The below program reads the total number of students and their scores and then display the grade of each student.
Explanation of Solution
Program:
//Import required packages
import java.io.*;
import java.util.Scanner;
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
//Class name
public class sample1 {
// Main Method
public static void main(String[] args) {
// Create a Scanner
Scanner input = new Scanner(System.in);
// Get the number of students
System.out.print("Enter the number of students: ");
int numberOfStudents = input.nextInt();
//Create an object for the static method
double[] scores = new double[numberOfStudents]; // Array scores
double best = 0; // The best score
// Read scores and find the best score
System.out.print("Enter " + numberOfStudents + " scores: ");
for (int i = 0; i < scores.length; i++) {
scores[i] = input.nextDouble();
if (scores[i] > best)
best = scores[i];
}
// Declare and initialize output string
char grade; // The grade
// Assign and display grades
for (int i = 0; i < scores.length; i++) {
if (scores[i] >= best - 10)
grade = 'A';
else if (scores[i] >= best - 20)
grade = 'B';
else if (scores[i] >= best - 30)
grade = 'C';
else if (scores[i] >= best - 40)
grade = 'D';
else
grade = 'F';
//Print the output
System.out.println("Student " + i + " score is " + scores[i]+ " and grade is " + grade);
}
}
}
Enter the number of students: 5
Enter 5 scores: 78 92 69 45 56
Student 0 score is 78.0 and grade is B
Student 1 score is 92.0 and grade is A
Student 2 score is 69.0 and grade is C
Student 3 score is 45.0 and grade is F
Student 4 score is 56.0 and grade is D
Want to see more full solutions like this?
Chapter 7 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Experiencing MIS
Absolute Java (6th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Introduction to Programming Using Visual Basic (10th Edition)
C++ How to Program (10th Edition)
Starting Out with Java: Early Objects (6th Edition)
- Assignment: Convert feet into meters: Write a program which reads the number in feet, converts it to meters, and displays the result. One foot is 0.305 meters. One foot is 12 inches. Sample Run Enter the number of feet 16.5 16.5 feet is 5.0325 meters 16.5 feet is 198.0 inchesarrow_forwardQ1/ Write a program that reads from the user the number of students in the class and their grades in one class, the program must find the largest and the lowest grades with the sequence of students and then find the average grade of the class, see the example below:arrow_forwardParking Charges) A parking garage charges a $2.00 minimum fee to park for up to threehours and an additional $0.50 per hour for each hour or part thereof over three hours. The maximumcharge for any given 24-hour period is $10.00. Assume that no car parks for longer than 24 hoursat a time. Write a program that will calculate and print the parking charges for each of three customers who parked their cars in this garage yesterday. You should enter the hours parked for eachcustomer. Your program should print the results in a tabular format, and should calculate and printthe total of yesterday's receipts. The program should use the function calculateCharges to determine the charge for each customer. Your outputs should appear in the following format:Car Hours Charge1 1.5 2.002 4.0 2.503 24.0 10.00TOTAL 29.5 14.50arrow_forward
- (DEBUG AND MAKE A FLOWCHART OF THIS PROGRAM) // This pseudocode is intended to display// employee net pay values. All employees have a standard// $45 deduction from their checks.// If an employee does not earn enough to cover the deduction,// an error message is displayed.// This example is modularized.start Declarations string name string EOFNAME = ZZZZ while name not equal to EOFNAME housekeeping() endwhile while name not equal to EOFNAME mainLoop() endwhile while name not equal to EOFNAME finish() endwhilestop housekeeping() output "Enter first name or ", EOFNAME, " to quit "return mainLoop() Declarations num hours num rate num DEDUCTION = 45 num net output "Enter hours worked for ", name input hours output "Enter hourly rate for ", name input rate gross = hours * rate net = gross - DEDUCTION if net > 0 then output "Net pay for ", name, " is ", net else output "Deductions not covered. Net is…arrow_forwardQuestions: Computer-Assisted Instruction) The use of computers in education is referred to as computer- assisted instruction (CAI). Write a program that will help an elementary school student learn multiplication. Use the rand function to produce two positive one-digit integers. The program should then prompt the user with a question, such as How much is 6 times 7? The student then inputs the answer. Next, the program checks the student’s answer. If it’s correct, display the message "Very good!" and ask another multiplication question. If the answer is wrong, display the message "No. Please try again." and let the student try the same question repeatedly until the student finally gets it right. A separate function should be used to generate each new question. This function should be called once when the application begins execution and each time the user answers the question correctly. PLEASE IN C++ LANGUAGEarrow_forwardQ7-Write a program that simulates a simple calculator. It reads two integers and a character. If the character is a +, the sum is printed; if it is a -, the difference is printed; if it is a *, the product is printed; if it is a /, the quotient is printed; and if it is a %, the remainder is printed. (Use a switch statement.)arrow_forward
- Q5. (Find the second lowest interger number) Write a program that prompts the user to enter a set of integer numbers, and finally displays the second lowest integer number in the set. To exit from the program enter -1. Here is a sample run Enter a set of integer numbers: 3 57 928-1 The second lowest number is 3.arrow_forwardQ1:Write C code for a program that reads two positive integer number, checks whether these number are co-prime or not, and prints the result.Note: two numbers are co-prime numbers if they do not have a common factor other than 1. For example, 10 and 11 are co-prime.arrow_forwardBackground: Game Rules The rules to the (dice) game of Pig: You will need 2 dice. To Play: a. The players each take turns rolling two die. b. A player scores the sum of the two dice thrown (unless the roll contains a 1): If a single number 1 is thrown on either die, the score for that whole turn is lost (referred to as “Pigged Out”). A 1 on both dice is scored as 25. c. During a single turn, a player may roll the dice as many times as they desire. The score for a single turn is the sum of the individual scores for each dice roll. d. The first player to reach the goal score wins unless a player scores higher subsequently in the same round. Therefore, everyone in the game must have the same number of turns. Execution and User Input This program is quite interactive with the user(s) and will take in the following information; please review the sample input / output sessions for details; we describe them again here emphasizing input. The program will prompt for the number of…arrow_forward
- .c## Write a Console Application that reads two integers, determines whether the first is a multiple of the second and displays the result. [Hint: Use the remainder operator.]arrow_forwardAlert dont submit AI generated answer. (Central city) Given a set of cities, the central city is the city that has the shortest total distance to all other cities. Write a program that prompts the user to enter the number of the cities and the locations of the cities (coordinates), and finds the central city and its total distance to all other cities. Sample Run Enter the number of cities: 5 Enter the coordinates of the cities: 2.5 5 5.1 3 1 9 5.4 54 5.5 2.1 The central city is at (2.5, 5.0) The total distance to all other cities is 60.81 Class Name: Exercise08_21arrow_forward[Calculate grades’ average for a student] write a program that calculates the student grades’ average for a semester for the number of courses taken in that semester. Your program should do the following: 1.Read from user the number of courses (n) 2. Then, read the courses’ grades for n times (Hint: use a loop) 3.If a grade is grater than 100 or less than 0, ask the user to enter the grade again. 4.Calculate the average of grades using the following formula: average = (sum of grades) / n 5.Print out the average grade on the screen. Note: Always use appropriate data types.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
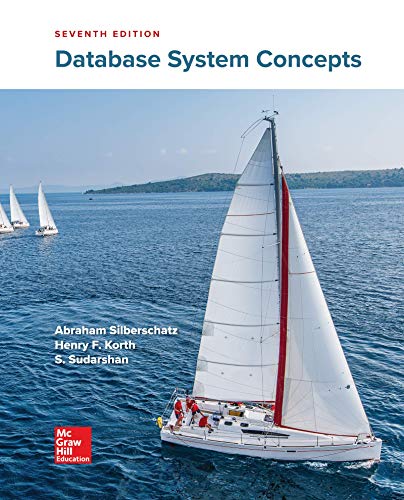
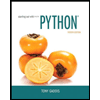
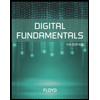
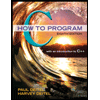
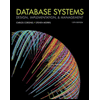
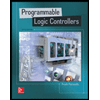