you will be adding a little bit of detail to your grid. Building off Milestone 1, you will add a circle to the center of each cell. The circle should be filled a different color than the grid You are still allowed to choose the fill and pen colors yourself.
you will be adding a little bit of detail to your grid. Building off Milestone 1, you will add a circle to the center of each cell. The circle should be filled a different color than the grid You are still allowed to choose the fill and pen colors yourself.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
you will be adding a little bit of detail to your grid. Building off Milestone 1, you will add a circle to the center of each cell. The circle should be filled a different color than the grid You are still allowed to choose the fill and pen colors yourself.
In addition to the 3 inputs you'll be asking from before, ask for an additional input for the circle's fill color.
Sample output:
Grid size (n): (input) Pen color: (input) Fill color: (input) Circle color: (input)
Here are some examples of what your program should be producing:
My code is this, which is something wrong, can you change my code to get the output as images shown:
# TODO: Write your header comment here.
import turtle
import random
turtle.tracer(False) # this will turn off drawing (recommended for development because drawing is slow, required for submitting or your code will timeout)
# TODO: Write your code here.
def create_grid_with_circle(size, pen_color, fill_color, circle_color):
# Set up the screen
screen = turtle.Screen()
size_for_canvas = size * 50 # Adjust multiplier as needed
screen.setup(width=size_for_canvas + 10, height=size_for_canvas + 10)
screen.screensize(size_for_canvas, size_for_canvas)
# Set up the turtle
grid_turtle = turtle.Turtle()
grid_turtle.speed(0) # Set the drawing speed, 0 is the fastest
turtle.tracer(False) # Turn off drawing for faster rendering
# Draw the grid
for i in range(size):
for j in range(size):
draw_cell(grid_turtle, -size_for_canvas / 2 + j * 50, size_for_canvas / 2 - i * 50, fill_color, pen_color)
draw_circle(grid_turtle, -size_for_canvas / 2 + j * 50, size_for_canvas / 2 - i * 50, circle_color)
# Function to draw a grid cell
def draw_cell(turtle, x, y, fill_color, pen_color):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.color(pen_color, fill_color)
turtle.begin_fill()
for _ in range(4):
turtle.forward(50)
turtle.right(90)
turtle.end_fill()
# Function to draw a circle in the center of a cell
def draw_circle(turtle, x, y, circle_color):
turtle.penup()
turtle.goto(x + 25, y - 25) # Move to the center of the cell
turtle.pendown()
turtle.color(circle_color, circle_color)
turtle.begin_fill()
turtle.circle(20) # Adjust the radius to ensure the circle does not touch the border
turtle.end_fill()
# Get user input
grid_size = int(input("Grid size (n): "))
pen_color = input("Pen color: ")
fill_color = input("Fill color: ")
circle_color = input("Circle color: ")
# Create the grid with circles
create_grid_with_circle(grid_size, pen_color, fill_color, circle_color)
turtle.tracer(True) # this will turn back on drawing, do not remove
turtle.mainloop() # Delete before submitting your code for grading otherwise your code will timeout and not su bmit, however, must include while developing your code in order to see the drawing (otherwise window will disappear)

Transcribed Image Text:●

Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
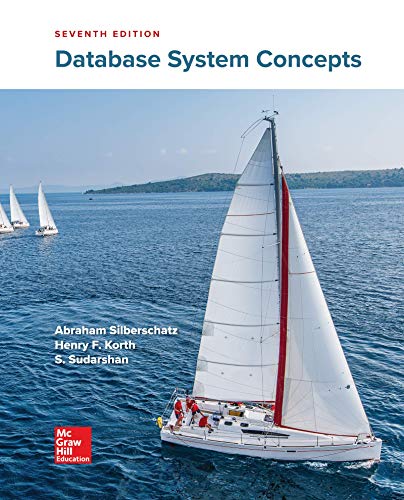
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
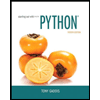
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
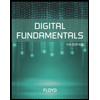
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
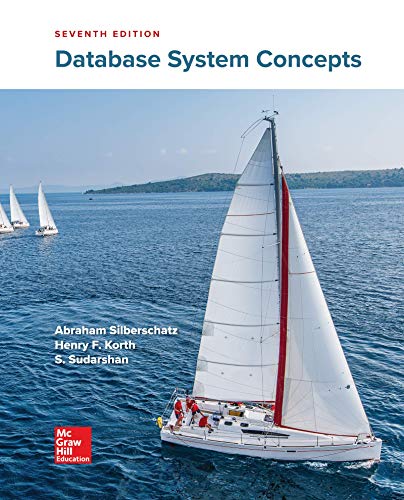
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
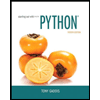
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
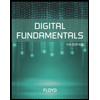
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
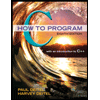
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
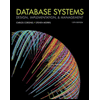
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
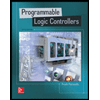
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education