You have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a `map' of all the underground resources in the mine. This map comes as a file. The file has n rows. Each row has n space-separated integers. Each integer is between zero and one hundred (inclusive). The file should be understood to represent a grid, n wide by n deep. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and n. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit the second row, and so on. When the digger reaches row number n, it has to be air-lifted out of the mine, and is no longer usable. Every time the digger hits a square with resources in it, we can reclaim those resources. • Write a program that reads as a command-line argument a number n, this number is the number of columns and rows mentioned above. • It then reads from standard input n * n integers, each integer being between zero (0) and one hundred (100), and then writes to standard out a single integer representing the maximum possible profit. Please provide your own solution. the one you copy as the images does not work for me!!
You have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a `map' of all the underground resources in the mine. This map comes as a file. The file has n rows. Each row has n space-separated integers. Each integer is between zero and one hundred (inclusive). The file should be understood to represent a grid, n wide by n deep. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and n. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit the second row, and so on. When the digger reaches row number n, it has to be air-lifted out of the mine, and is no longer usable. Every time the digger hits a square with resources in it, we can reclaim those resources.
• Write a program that reads as a command-line argument a number n, this number is the number of columns and rows mentioned above.
• It then reads from standard input n * n integers, each integer being between zero (0) and one hundred (100), and then writes to standard out a single integer representing the maximum possible profit.
Please provide your own solution. the one you copy as the images does not work for me!!
![import java.io.*;
import java.lang.*;
public class CodeChef
{
static int findMaxPath(int gridII, int n)
{
int result = -1;
// loop to find maximum if grid has n=1
for(int i=0; i<n; i++)
{
result = Math.max(result,grid[0][i);
}
for(int i=1; i<n; i++)
{
result = -1;
for(int j=0; j<n; j++)
{
if(j>0 && j<n-1) // when he can come from both left-up or right-up part
{
grid[i][]+=Math.max(grid[i-1][j-1], grid[i-1][j+1]);
else if(j>0) / when he can come from right-up part
{
grid[i]1]+=grid[i-1][i-1);
}
else if(j<n-1) // when he can come from left-up part
{
grid[i]i]+=grid[i-1][j+1];
}
result = Math.max(grid[i][i], result);
return result;
}
public static void main(String args[]) throws IOException
int n = Integer.parselnt(args[0]);
File file = new File(args[1]);
BufferedReader br = new BufferedReader(new FileReader(file));
int grid[]] = new int[n][n];
String line;
int i=0;
while((line=br.readLine()!=null)
{
String arr[] = line.split(" ");
for(int j=0; j<n; j++)
{
grid[i]]=Integer.parselnt(arr[i]);
}
i++;
System.out.println(findMaxPath(grid,n);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8f82238c-98e2-4dc0-a77e-6eb7cbfbbd83%2F9edbfb38-122a-4c12-8032-18c00a28cb65%2F3eri7ur_processed.png&w=3840&q=75)
![CODE:
import java.util.*;
public class Operations {
public static Scanner scn=new Scanner(System.in);
public static void main(String[] args){ // driver function
int n=scn.nextInt(); I/number of rows and columns
intIl grid=new int[n][n]; //grid to map the resources
for(int i=0;i<n;i++){ l/input the values for the grid
for(int j=0;j<n;j++){
grid[i][]=scn.nextInt();
}
int[]] dp=new int[n][n]; /I/dp matrix where dp[i]li] represents maximum resources we can collect by starting
at the position (i.j)
for(int j=0;j<n;j++){
dp[n-1][i)=grid[n-1][];
}
for(int i=n-2;i>=0;i--{
for(int j=0;j<n;j++){
if(j-1>=0 && j+1<n}{ ///if it is possible to go left-down and right-down
dp[i]l]=Math.max(dp[i+1][j+1],dp[i+1][j-1])+grid[i][];
}
else if(j-1>=0 && j+1>=n}{ I//if it is possible to go left-down only
dp[i]i]=dp[i+1][j-1]+grid[1][i];
}
else( Il/if it is possible to go right-down only
dp[i]i]=dp[i+1][j+1]+grid[i][j];
}
}
int ans=0; //final answer
for(int j=0;j<n;j++){
ans=Math.max(ans,dp[0][i]); /I/compare answer to each point in the first row
}
System.out.println(ans);//standard out the final answer
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8f82238c-98e2-4dc0-a77e-6eb7cbfbbd83%2F9edbfb38-122a-4c12-8032-18c00a28cb65%2F0ldym8o_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

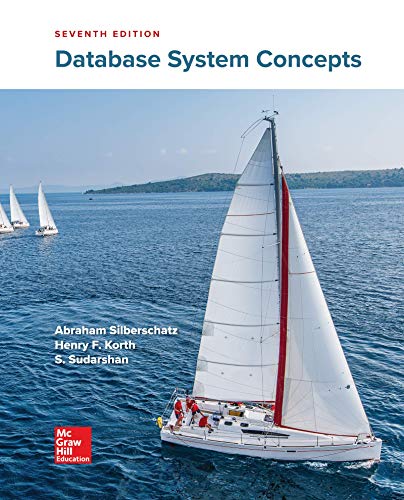
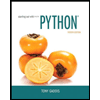
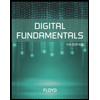
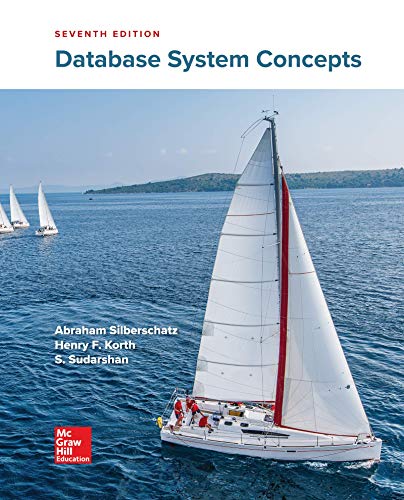
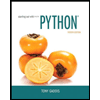
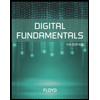
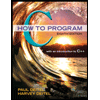
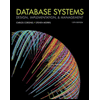
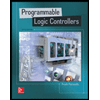