You are going to create a program that will demonstrate your ability to define different functions to process a list. Then you will need to write two additional functions that allow you to access the data from a text file and save the data to a text file. You will need to provide the appropriate calls to these functions in the main method. You will be provided with a starter file and will need to complete the methods outlined in the sections below. Follow the directions in each section to earn the points possible and you must provide a docString for every function you create. Stage 1 - findMin and findMax The Stage 1 version requires you to complete the return findMin and findMax functions. Both function headers are provided to you and you will need to replace the pass instruction with the code necessary to complete each function. The findMin function will iterate over the list to access each score individually to find the smallest value in the list. It will then return the smallest value in the list. Likewise the findMax will do the same but return the largest value in the list. You are not to sort the list or use any built in python function or external libraries for these functions. Stage 1 - Output Stage 2 - dropLowest() The Stage 2 version adds the void function dropLowest function to the program. The function should accept a single list of integers as a parameter. The function should make a call to the findMin function and then remove the score it returns from the list. Make the appropriate call in the main function and add another call to the displayScores function so we can see the lowest score is now missing Stage 2 – Output Stage 3 - getMean() The Stage 3 version adds the return getMean function. This function will accept a single list of integer values and return the average of all the values in the list. It must iteratively process the list to generate the total of all the values in the list. You must make the appropriate call to the function and display the results once before and after dropping the lowest score. Stage 3 Sample Ouput Stage 4 - findMedian() The Stage 4 version has you add the return getMedian function. This function accepts a single list of integers as a parameter and will return the median value of the list. To calculate the median you must first know if there are an odd or even number of elements. See this explanation for help. You must make the appropriate call to the function and display the results once before and after dropping the lowest score. Stage 4 – Sample Output Stage 5 - getScores and saveScores() The Stage 5 version has you add the getScores and saveScores function. Function getScores does not require a parameter and will have you open a text file of scores called scores.txt, read in each score and append it to a list. You must return the list of scores created. Replace the first line of code in the main method from testScores = [90,85,52,74,95,100,78] to testScores = getScores(). Function saveScores will require a single list of integers as a parameter. It will save the list’s contents to a text file named updatedScores.txt. It will write each score in the file as a single entry in each line of text. Assignment4.py is the picture scores.txt 95 74 98 76 84 89 92 74 in python please
You are going to create a program that will demonstrate your ability to define different functions to
process a list. Then you will need to write two additional functions that allow you to access the data
from a text file and save the data to a text file. You will need to provide the appropriate calls to these
functions in the main method.
You will be provided with a starter file and will need to complete the methods outlined in the sections
below. Follow the directions in each section to earn the points possible and you must provide a
docString for every function you create.
Stage 1 - findMin and findMax
The Stage 1 version requires you to complete the return findMin and findMax functions. Both
function headers are provided to you and you will need to replace the pass instruction with the code
necessary to complete each function. The findMin function will iterate over the list to access each
score individually to find the smallest value in the list. It will then return the smallest value in the list.
Likewise the findMax will do the same but return the largest value in the list. You are not to sort the
list or use any built in python function or external libraries for these functions.
Stage 1 - Output
Stage 2 - dropLowest()
The Stage 2 version adds the void function dropLowest function to the program. The function should
accept a single list of integers as a parameter. The function should make a call to the findMin
function and then remove the score it returns from the list. Make the appropriate call in the main
function and add another call to the displayScores function so we can see the lowest score is now
missing
Stage 2 – Output
Stage 3 - getMean()
The Stage 3 version adds the return getMean function. This function will accept a single list of
integer values and return the average of all the values in the list. It must iteratively process the list
to generate the total of all the values in the list. You must make the appropriate call to the
function and display the results once before and after dropping the lowest score.
Stage 3 Sample Ouput
Stage 4 - findMedian()
The Stage 4 version has you add the return getMedian function. This function accepts a single list of
integers as a parameter and will return the median value of the list. To calculate the median you
must first know if there are an odd or even number of elements. See this explanation for help. You
must make the appropriate call to the function and display the results once before and after dropping
the lowest score.
Stage 4 – Sample Output
Stage 5 - getScores and saveScores()
The Stage 5 version has you add the getScores and saveScores function.
Function getScores does not require a parameter and will have you open a text file of scores called
scores.txt, read in each score and append it to a list. You must return the list of scores created.
Replace the first line of code in the main method from testScores = [90,85,52,74,95,100,78] to
testScores = getScores().
Function saveScores will require a single list of integers as a parameter. It will save the list’s contents
to a text file named updatedScores.txt. It will write each score in the file as a single entry in each line
of text.
Assignment4.py is the picture
scores.txt
95
74
98
76
84
89
92
74
in python please
![def displayScores (scoreArray) :
"""Displays the current test scores"""
print ("The current test scores are: ")
for i in range (len (scoreArray) -1):
print (float (scoreArray[i]), end= ", ")
print (float (scoreArray [len (scoreArray)-1]), end = "\n")
def findMin (scoreArray) :
"""Finds the minimum value in list passed by parameter"""
pass
def findMax (scoreArray) :
"""Finds the maximum value in list passed by parameter"""
pass
def main ():
testScores = [90,85, 52, 74, 95, 100, 781
displayScores (testScores)
print ()
minimum = findMin (testScores)
print ("Lowest Score: ", minimum)
maximum = findMax (testScores)
print ("Highest Score: ", maximum)
#Replace the commments for each stage with the appropriate function calls
main ()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc4b4111f-0cb3-46a3-bff1-74a667fd5dd6%2F82920dc9-514a-4709-8a32-2358fec7c361%2Fgt280w6_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

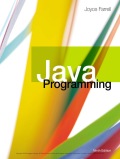
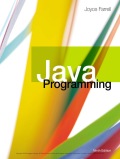