Write the following program in C++. 1. Create a class called Employee that includes attributes: empid, name, points, group, and avg with data types: “int”, “String”, “double”, “String”, and “double” respectively. 2. Include a constructor with parameters: empid and name. 3. Include another constructor to assign default values to the attributes. 4. Include a function called addPoints that is used to add a given amount to the value of the attribute points. 5. Include a function called upgradePoints that is used to increase the value of the attribute points by a given percentage. 6. Include a function called removePoints that is used to reduce a given amount from the value of the attribute points. If the resultant value is negative then the value of the attribute should be set into zero. 7. Include a function called computeGroup that assigns a value to the attribute group based on the value of the attribute points as given in the following table. Points Group points < 100 Silver 100 ≤ points < 500 Gold 500 < points ≤ 2000 Platinum 2000 < points Diamond 8. Include a function called display to display the values of all the attributes of a Employee object. 9. Include a static function called getBest that returns the value of the attribute id of the Employee with maximum number of points. 10. Create an array in main function that holds five Employee objects. Assign the objects given in the following table to each array element using the parameterized constructor. Attributes Obj[0] Obj[1] Obj[2] Obj[3] Obj[4] ID 1543 6561 6954 3485 8546 Name Adil Waseem Ayesha Adnan Wajid 11. Use the function addPoints to add following points respectively to each Employee object created above. 129 785 3258 59 1652 12. Add extra 1000 points to the third employee using the function addPoints. 13. Increase the points of the second employee by 2% using the function upgradePoints. 14. Include a function called display to the that displays all the attributes of each Employee. Each set of attributes should be separated by a sequence of dots. The part of output for the first Employee is given below. …………………………… Employee: 1 ID: 1543 Name: Nimal Points: 129.0 Group: null Average: 0.0 15. Call the function display, 16. Compute the group of each Employee using the function computeGroup. You should use a loop. 17. Again, call the function display. 18. Display the value of the attribute id of the Employee with highest number of points using the function getBest. 19. Create a reference variable called emp of type Employee. 20. Assign fifth Employee object to the reference variable emp. You should use the object you have already created. 21. Reduce 2000 points from the fifth Employee using the function removePoints. You should call the function using the reference variable emp. 22. Call the function display of the class Employee using the array element c[4] to display the values of attributes of the fifth Employee. 23. Update the group of the fifth Employee invoking the function computeGroup. 24. Create a Employee object with default values to its attributes by using the appropriate constructor. The object should be assigned to the reference variable emp. 25. Copy the values of the attributes of the fourth Employee to the corresponding attributes of the object pointed by the reference variable emp. 26. Create another reference variable called emp1 of type Employee. 27. Assign the object pointed by the reference variable emp to emp1 as well. 28. call the function display for objects pointed by variable emp and emp1.
Write the following program in C++.
1. Create a class called Employee that includes attributes: empid, name, points,
group, and avg with data types: “int”, “String”, “double”, “String”, and “double”
respectively.
2. Include a constructor with parameters: empid and name.
3. Include another constructor to assign default values to the attributes.
4. Include a function called addPoints that is used to add a given amount to the value
of the attribute points.
5. Include a function called upgradePoints that is used to increase the value of the
attribute points by a given percentage.
6. Include a function called removePoints that is used to reduce a given amount from
the value of the attribute points. If the resultant value is negative then the value of
the attribute should be set into zero.
7. Include a function called computeGroup that assigns a value to the attribute group
based on the value of the attribute points as given in the following table.
Points | Group |
points < 100 |
Silver |
100 ≤ points < 500 | Gold |
500 < points ≤ 2000 | Platinum |
2000 < points | Diamond |
8. Include a function called display to display the values of all the attributes of a Employee object.
9. Include a static function called getBest that returns the value of the attribute id of the Employee with maximum number of points.
10. Create an array in main function that holds five Employee objects. Assign the objects given in the following table to each array element using the parameterized constructor.
Attributes | Obj[0] | Obj[1] | Obj[2] | Obj[3] | Obj[4] |
ID | 1543 | 6561 | 6954 | 3485 | 8546 |
Name | Adil | Waseem | Ayesha | Adnan | Wajid |
11. Use the function addPoints to add following points respectively to each Employee object created above.
129 | 785 | 3258 | 59 | 1652 |
12. Add extra 1000 points to the third employee using the function addPoints.
13. Increase the points of the second employee by 2% using the function
upgradePoints.
14. Include a function called display to the that displays all the attributes of each Employee. Each set of attributes should be separated by a sequence of dots. The part of output for the first Employee is given below.
……………………………
Employee: 1
ID: 1543
Name: Nimal
Points: 129.0
Group: null
Average: 0.0
15. Call the function display,
16. Compute the group of each Employee using the function computeGroup. You should use a loop.
17. Again, call the function display.
18. Display the value of the attribute id of the Employee with highest number of points using the function getBest.
19. Create a reference variable called emp of type Employee.
20. Assign fifth Employee object to the reference variable emp. You should use the object you have already created.
21. Reduce 2000 points from the fifth Employee using the function removePoints. You should call the function using the reference variable emp.
22. Call the function display of the class Employee using the array element c[4] to display the values of attributes of the fifth Employee.
23. Update the group of the fifth Employee invoking the function computeGroup.
24. Create a Employee object with default values to its attributes by using the appropriate constructor. The object should be assigned to the reference variable emp.
25. Copy the values of the attributes of the fourth Employee to the corresponding attributes of the object pointed by the reference variable emp.
26. Create another reference variable called emp1 of type Employee.
27. Assign the object pointed by the reference variable emp to emp1 as well.
28. call the function display for objects pointed by variable emp and emp1.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

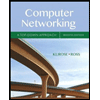
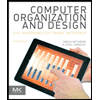
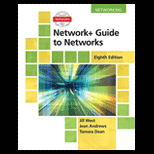
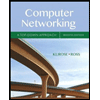
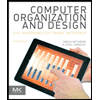
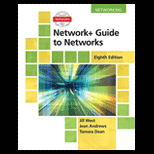
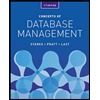
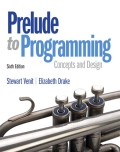
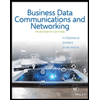