Write the 3 classes for the Java code below. the 3 classes and their details: Circle Circle should be a sub-class of Shape, so it inherits all of the above plus... 1 protected instance variable to store the circle's radius. 1 public constructor with 1 argument to initialize the radius. 2 public methods which override Shape methods: area and perimeter. (Use the @Override annotation.) They should return the area and perimeter of the circle. Rectangle Rectangle should be a sub-class of Shape, so it inherits all of Shape's members plus... 2 protected instance variables to store the rectangle's length and height. 1 public constructor with 2 arguments to initialize the length and height. 2 public methods: area and perimeter. (Again, use the @Override annotation.) They should return the area and perimeter of the rectangle. Square Square should be a sub-class of Rectangle, so it inherits all of Rectangle's members plus... 1 public constructor with 1 argument to initialize the length and height to be that value. Code: class Main { publicstaticvoid main(String[] args) { // Array to store all types of Shape objects: Shape[] shapes = new Shape[4]; // Parallel array to store text descriptions of each shape. String[] descriptions = new String[4]; shapes[0] = new Circle(10); descriptions[0] = "10 unit radius Circle"; shapes[1] = new Rectangle(15, 25); descriptions[1] = "15x25 Rectangle"; shapes[2] = new Square(20); descriptions[2] = "20 unit Square"; shapes[3] = new Circle(2); descriptions[3] = "2 unit radius Circle"; System.out.println("This program examines the ratio of area to perimeter"); System.out.println("for a few different shapes."); System.out.println("(The larger the ratio, the greater the efficiency of space contained)"); for(int i=0; i/
Write the 3 classes for the Java code below. the 3 classes and their details: Circle Circle should be a sub-class of Shape, so it inherits all of the above plus... 1 protected instance variable to store the circle's radius. 1 public constructor with 1 argument to initialize the radius. 2 public methods which override Shape methods: area and perimeter. (Use the @Override annotation.) They should return the area and perimeter of the circle. Rectangle Rectangle should be a sub-class of Shape, so it inherits all of Shape's members plus... 2 protected instance variables to store the rectangle's length and height. 1 public constructor with 2 arguments to initialize the length and height. 2 public methods: area and perimeter. (Again, use the @Override annotation.) They should return the area and perimeter of the rectangle. Square Square should be a sub-class of Rectangle, so it inherits all of Rectangle's members plus... 1 public constructor with 1 argument to initialize the length and height to be that value. Code: class Main { publicstaticvoid main(String[] args) { // Array to store all types of Shape objects: Shape[] shapes = new Shape[4]; // Parallel array to store text descriptions of each shape. String[] descriptions = new String[4]; shapes[0] = new Circle(10); descriptions[0] = "10 unit radius Circle"; shapes[1] = new Rectangle(15, 25); descriptions[1] = "15x25 Rectangle"; shapes[2] = new Square(20); descriptions[2] = "20 unit Square"; shapes[3] = new Circle(2); descriptions[3] = "2 unit radius Circle"; System.out.println("This program examines the ratio of area to perimeter"); System.out.println("for a few different shapes."); System.out.println("(The larger the ratio, the greater the efficiency of space contained)"); for(int i=0; i/
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 2CP
Related questions
Question
100%
Write the 3 classes for the Java code below.
the 3 classes and their details:
- Circle
- Circle should be a sub-class of Shape, so it inherits all of the above plus...
- 1 protected instance variable to store the circle's radius.
- 1 public constructor with 1 argument to initialize the radius.
- 2 public methods which override Shape methods: area and perimeter. (Use the @Override annotation.) They should return the area and perimeter of the circle.
- Rectangle
- Rectangle should be a sub-class of Shape, so it inherits all of Shape's members plus...
- 2 protected instance variables to store the rectangle's length and height.
- 1 public constructor with 2 arguments to initialize the length and height.
- 2 public methods: area and perimeter. (Again, use the @Override annotation.) They should return the area and perimeter of the rectangle.
- Square
- Square should be a sub-class of Rectangle, so it inherits all of Rectangle's members plus...
- 1 public constructor with 1 argument to initialize the length and height to be that value.
Code:
class Main
{
publicstaticvoid main(String[] args)
{
// Array to store all types of Shape objects:
Shape[] shapes = new Shape[4];
// Parallel array to store text descriptions of each shape.
String[] descriptions = new String[4];
shapes[0] = new Circle(10);
descriptions[0] = "10 unit radius Circle";
shapes[1] = new Rectangle(15, 25);
descriptions[1] = "15x25 Rectangle";
shapes[2] = new Square(20);
descriptions[2] = "20 unit Square";
shapes[3] = new Circle(2);
descriptions[3] = "2 unit radius Circle";
System.out.println("This program examines the ratio of area to perimeter");
System.out.println("for a few different shapes.");
System.out.println("(The larger the ratio, the greater the efficiency of space contained)");
for(int i=0; i<shapes.length && i<descriptions.length; i++)
{
System.out.println("Shape #" + shapes[i].getId()
+ " is a " + descriptions[i] + " with ratio "
+ shapes[i].area() / shapes[i].perimeter());
}
}
}
/* Output:
This program examines the ratio of area to perimeter
for a few different shapes.
(The larger the ratio, the greater the efficiency of space contained)
Shape #1 is a 10 unit radius Circle with ratio 5.0
Shape #2 is a 15x25 Rectangle with ratio 4.6875
Shape #3 is a 20 unit Square with ratio 5.0
Shape #4 is a 2 unit radius Circle with ratio 1.0
*/
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
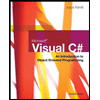
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
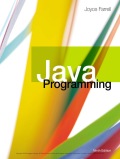
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
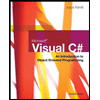
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
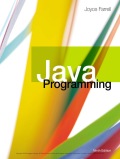
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT