Write an assembly program that first reads a 2 digit decimal number using the function "reads" then checks if the number is prime or not using the function "isPrime" and then shows on the display a message that says if the number was prime or not by using the function "results". The program should run in an infinite loop (when the function results returns, the program jumps back to function readS). A typical screen dump of the console should look something like this: Input a 2 digit decimal number:12 The number is not prime Input a 2 digit decimal number:02 The number is prime
LC3 ASSEMBLY HELP. I have written the code for "Reads", "IsPrime" and "ResultS" now i need to combine them so they work as an
"Reads" Code:
.ORIG x3000
readS LEA R0, prompt ; Load effective address of prompt message
PUTS ; Display prompt message
AND R0, R0, #0 ; Clear R0 to store the result
; Read first digit
GETC ; Read first digit
OUT ; Echo first digit
LD R1, ASCII_OFFSET ; Load ASCII offset to subtract from digit character
ADD R0, R0, R1 ; Convert digit character to integer
; Subtract '0' (ASCII 48) from the character to get the numerical value
ADD R0, R0, #-48 ; Convert ASCII digit to integer
; Shift left by 4 bits to make room for the second digit
ADD R0, R0, R0 ; Shift to left to make room for second digit
; Read second digit
GETC ; Read second digit
OUT ; Echo second digit
LD R1, ASCII_OFFSET ; Load ASCII offset to subtract from digit character
ADD R2, R0, R1 ; Convert digit character to integer (store in R2)
ADD R2, R2, #-48 ; Convert ASCII digit to integer (of the second digit)
; Add the two digits (already converted to integers) and store in R0
ADD R0, R0, R2 ; Combine first and second digit
RET ; Return from function
prompt .STRINGZ "Input a 2 digit decimal number: "
ASCII_OFFSET .FILL xFFD0 ; ASCII offset to convert digit character to integer
.END
"IsPrime" Code:
.ORIG x3000
isPrime
ST R1, SAVE_R1 ; Save the original value of R1
AND R1, R1, #0 ; Clear R1 (to use as a counter)
BRz ZERO_CASE ; Handle the case when the input is 0
LOOP
ADD R1, R1, #1 ; Increment the counter
ADD R1, R1, #1 ; Check for divisibility by counter + counter
DIV R0, R1 ; Divide the input by (counter + counter)
BRnp IS_PRIME ; If the remainder is not 0, the number is prime
BRz NOT_PRIME ; If the remainder is 0, the number is not prime
IS_PRIME
AND R0, R0, #0 ; Clear R0
ADD R0, R0, #1 ; Set R0 to 1 (prime)
BRnzp DONE ; Branch to DONE
NOT_PRIME
AND R0, R0, #0 ; Clear R0 (not prime)
BRnzp DONE ; Branch to DONE
ZERO_CASE
AND R0, R0, #0 ; Clear R0 (0 is not prime)
DONE
LD R1, SAVE_R1 ; Restore the original value of R1
RET ; Return from the function
SAVE_R1 .BLKW 1 ; Storage for saving R1
.END
"ResultS" Code:
.orig x3000
;; Function: results
results LD R0, RO ; Load the value from RO into R0
BRz not_prime ; Branch if the value in R0 is zero
LEA R0, prime_msg ; Load the address of the prime message
OUT ; Output the prime message
HALT ; Halt the program
not_prime
LEA R0, not_prime_msg ; Load the address of the not prime message
OUT ; Output the not prime message
HALT ; Halt the program
;; Data section
prime_msg .STRINGZ "The number is prime\n"
not_prime_msg .STRINGZ "The number is not prime\n"
RO .FILL #value ; Fill the value of RO here
.end


Step by step
Solved in 1 steps

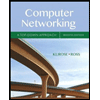
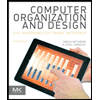
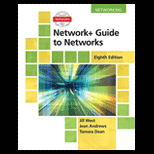
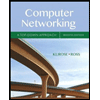
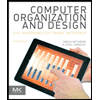
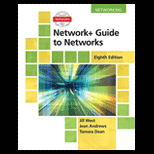
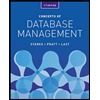
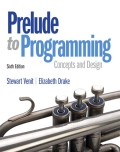
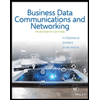