Write a python code Step 1: Start Visual Studio Code.. Prior to entering code, save your file by clicking on File and then Save. Select your location and save this file as Lab7.py. Be sure to include the .py extension. Step 2: Document the first few lines of your program to include your name, the date, and a brief description of what the program does. Step 3: Start your program with the following code for main: # Lab 7-3 The Dice Game # add libraries needed # the main function def main(): print() # initialize variables # call to inputNames # while loop to run program again while endProgram == 'no': # populate variables # call to rollDice # call to displayInfo endProgram = input('Do you want to end program? (yes/no): ') #this function gets the players names #this function will get the random values #this function displays the winner # calls main main() Step 4: Under the comment for adding libraries, add the following statement: import random Step 5: Under the comment for initialize variables, set endProgram to ‘no’ and playerOne and playerTwo to ‘NO NAME’. Step 6: Under the comment for making a call to inputNames, set the function call to both playerOne and playerTwo and pass both variables to the function as arguments. This must be done because both values need to be returned from the function. This is done as follows: playerOne, playerTwo = inputNames(playerOne, playerTwo) Step 7: Inside your while loop, set winnersName to ‘NO NAME’ and p1number and p2number to 0. Step 8: Make a call to rollDice and pass the necessary variables needed in this function. This function should be set to the winnerName as that variable will be returned from the function. This is done as follows: winnerName = rollDice(p1number, p2number, playerOne, playerTwo, winnerName) Step 9: Make a call to displayInfo and pass it winnerName. Step 10: The next step is to write the function that will allow both players to enter their names. Write a function heading that matches your function call in Step 6, making sure to accept two arguments. The body of this function will use the input function to take in both players names, and one return statement that returns both playerOne and playerTwo variable The return statement should look as follows: return playerOne, playerTwo Step 11: The next function to code is the rollDice function. Write the function header to match the function call in Step 8. This function body will call the random function to determine p1number and p2number. The code should look as follows: p1number = random.randint(1, 6) p2number = random.randint(1, 6) Step 12: Next, inside this function write a nested if else statement that will set winnerName to either playerOne, playerTwo, or "TIE". Step 13: The final step in this function is to return winnerName. Step 14: The final function to code is the displayInfo function. Write the function header to match the call made in Step 9. The body of the function should simply print the winnerName variable to the screen.
Write a python code
Step 1: Start Visual Studio Code.. Prior to entering code, save your file by clicking on File and then Save. Select your location and save this file as Lab7.py. Be sure to include the .py extension.
Step 2: Document the first few lines of your
Step 3: Start your program with the following code for main:
# Lab 7-3 The Dice Game
# add libraries needed
# the main function
def main():
print()
# initialize variables
# call to inputNames
# while loop to run program again
while endProgram == 'no':
# populate variables
# call to rollDice
# call to displayInfo
endProgram = input('Do you want to end program? (yes/no): ')
#this function gets the players names
#this function will get the random values
#this function displays the winner
# calls main
main()
Step 4: Under the comment for adding libraries, add the following statement:
import random
Step 5: Under the comment for initialize variables, set endProgram to ‘no’ and playerOne and playerTwo to ‘NO NAME’.
Step 6: Under the comment for making a call to inputNames, set the function call to both playerOne and playerTwo and pass both variables to the function as arguments. This must be done because both values need to be returned from the function. This is done as follows:
playerOne, playerTwo = inputNames(playerOne, playerTwo)
Step 7: Inside your while loop, set winnersName to ‘NO NAME’ and p1number and p2number to 0.
Step 8: Make a call to rollDice and pass the necessary variables needed in this function. This function should be set to the winnerName as that variable will be returned from the function. This is done as follows:
winnerName = rollDice(p1number, p2number, playerOne, playerTwo,
winnerName)
Step 9: Make a call to displayInfo and pass it winnerName.
Step 10: The next step is to write the function that will allow both players to enter their names. Write a function heading that matches your function call in Step 6, making sure to accept two arguments. The body of this function will use the input function to take in both players names, and one return statement that returns both playerOne and playerTwo variable The return statement should look as follows:
return playerOne, playerTwo
Step 11: The next function to code is the rollDice function. Write the function header to match the function call in Step 8. This function body will call the random function to determine p1number and p2number. The code should look as follows:
p1number = random.randint(1, 6)
p2number = random.randint(1, 6)
Step 12: Next, inside this function write a nested if else statement that will set winnerName to either playerOne, playerTwo, or "TIE".
Step 13: The final step in this function is to return winnerName.
Step 14: The final function to code is the displayInfo function. Write the function header to match the call made in Step 9. The body of the function should simply print the winnerName variable to the screen.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

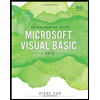
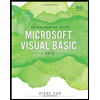