Using Python write a genetic algorithm code to solve this strange bank problem. Suppose, you are the owner of a bank that operates in a strange way. Customers can lend money from your bank (just like a normal bank) and they can also deposit money in your bank. A register is maintained to track the daily transactions. However, being the strange owner of a strange bank, you have a fascination with finding out whether a portion of your daily transactions (in/out) balance out to zero. For example, 1 Lend 100 2 Deposit 150 3 Lend 400 4 Lend 500 5 Deposit 1000 6 Lend 460 7 Deposit 160 8 Deposit 200 9 Lend 500 10 Deposit 100 In this case, there is a portion of the transactions that would balance itself out. (6th, 7th, 8th, and 10th transactions would amount to 0). Task Breakdown: Model the transaction register in a way suitable for the problem. Write a fitness function. Hint: It is the sum of the non-zero elements of a register. Write the crossover function. Write the mutation function. Create a population of randomly generated registers. Run genetic algorithms on the population until highest fitness has been reached and/or number of maximum iterations has been reached. Input The first line has a number N denoting the number of daily transactions followed by N lines each starting with either l or d and a number S denoting the amount of transaction. Here: N ( 1 <=N<= 10^2 ) S ( 1 <=S<= 10^5 ) Output
Using Python write a genetic
Suppose, you are the owner of a bank that operates in a strange way. Customers can lend money from your bank (just like a normal bank) and they can also deposit money in your bank. A register is maintained to track the daily transactions. However, being the strange owner of a strange bank, you have a fascination with finding out whether a portion of your daily transactions (in/out) balance out to zero. For example,
1 |
Lend |
100 |
2 |
Deposit |
150 |
3 |
Lend |
400 |
4 |
Lend |
500 |
5 |
Deposit |
1000 |
6 |
Lend |
460 |
7 |
Deposit |
160 |
8 |
Deposit |
200 |
9 |
Lend |
500 |
10 |
Deposit |
100 |
In this case, there is a portion of the transactions that would balance itself out. (6th, 7th, 8th, and 10th transactions would amount to 0).
Task Breakdown:
- Model the transaction register in a way suitable for the problem.
- Write a fitness function. Hint: It is the sum of the non-zero elements of a register.
- Write the crossover function.
- Write the mutation function.
- Create a population of randomly generated registers.
- Run genetic algorithms on the population until highest fitness has been reached and/or number of maximum iterations has been reached.
Input
The first line has a number N denoting the number of daily transactions followed by N lines each starting with either l or d and a number S denoting the amount of transaction. Here:
N ( 1 <=N<= 10^2 )
S ( 1 <=S<= 10^5 )
Output
The output would be a binary string denoting the specific transactions that balance themselves to zero or -1 if such a string cannot be formed. String consisting of all zeros won’t be accepted.


Step by step
Solved in 3 steps with 2 images

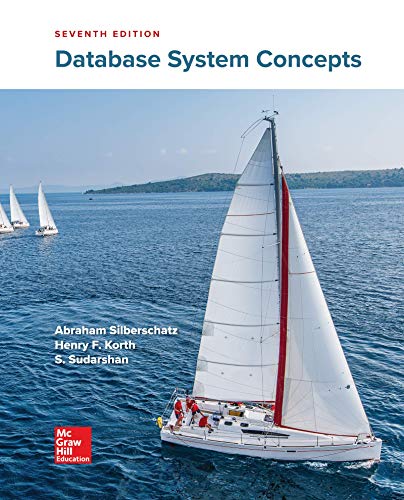
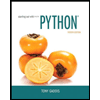
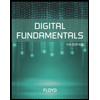
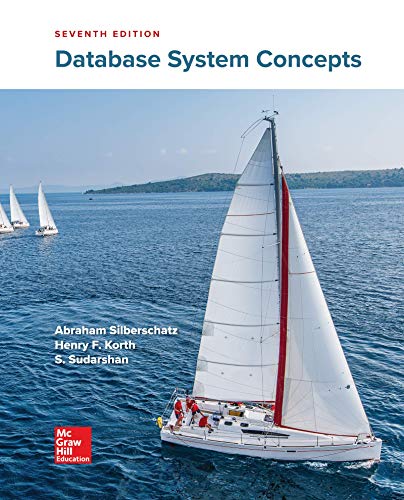
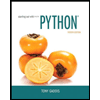
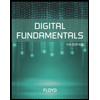
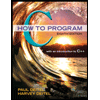
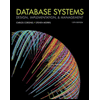
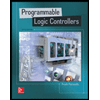