USE THE PROGRAM CODE BELOW TO CODE 1. COMMAND LINE ARGS CODE IN JAVA 1. Command Line Args Modify problem # 1 from Lab 8 to accept the values for the key as command line arguments. Your program may assume the arguments given at the command line are integers. If there are no arguments, print a message. If there is at least one argument, compute and print the average of the arguments. Note that you will need to use the parseInt method of the Integer class to extract integer values from the strings that are passed in. If any non-integer values are passed in, your program will produce an error, which is unavoidable at this point. Test your program in NetBeans using the data for problem # 1 Lab 8 as command line arguments. import java.util.Scanner; import java.text.NumberFormat; public class Quizzes { //---------------------------------------------- // Read in the number of questions followed by // the key, then read in each student's answers // and calculate the number and percent correct. //---------------------------------------------- public static void main(String[] args) { int numQuestions; int numCorrect; String anotherQuiz; int answer; NumberFormat percent = NumberFormat.getPercentInstance(); Scanner scan = new Scanner (System.in); System.out.println ("Quiz Grading"); System.out.println (); System.out.println("Enter the number of questions on the quiz: "); numQuestions = scan.nextInt(); //CREATE THE ARRAY FOR THE KEY int[] key = new int[numQuestions]; System.out.println("Enter the answer key: "); //LOAD THE ARRAY FOR THE ANSWER KEY WITH INPUT FROM THE USER for (int i = 0; i < numQuestions; i++) { key[i] = scan.nextInt(); } //OUTER LOOP TO ALLOW THE USER TO ENTER GRADES // FOR ANY NUMBER OF QUIZZES do { //INNER LOOP TO GET ANSWERS FROM THE USER AND COUNT THE NUMBER OF // CORRECT ANSWERS System.out.println("Enter the student answers: "); numCorrect = 0; for (int i = 0; i < numQuestions; i++) { answer = scan.nextInt(); if (answer == key[i]) { numCorrect++; } } //DISPLAY THE NUMBER OF CORRECT ANSWERS AND PERCENT System.out.println("Number of correct answers: " + numCorrect + " | Percent: " + percent.format((double)numCorrect/numQuestions)); System.out.println(); //ASK USER IF THEY WISH TO GRADE ANOTHER QUIZ System.out.println("Grade another quiz? (y/n): "); anotherQuiz = scan.next(); if (anotherQuiz.equalsIgnoreCase("y")) { } else { System.out.println("Good-bye"); break; } } while (true); } }
USE THE
CODE IN JAVA
1. Command Line Args
Modify problem # 1 from Lab 8 to accept the values for the key as command line arguments.
Your program may assume the arguments given at the command line are integers. If there are no
arguments, print a message. If there is at least one argument, compute and print the average of the arguments.
Note that you will need to use the parseInt method of the Integer class to extract integer values from the strings that are passed in. If any non-integer values are passed in, your program will produce an error, which is unavoidable at this point.
Test your program in NetBeans using the data for problem # 1 Lab 8 as command line arguments.
import java.util.Scanner;
import java.text.NumberFormat;
public class Quizzes
{
//----------------------------------------------
// Read in the number of questions followed by
// the key, then read in each student's answers
// and calculate the number and percent correct.
//----------------------------------------------
public static void main(String[] args)
{
int numQuestions;
int numCorrect;
String anotherQuiz;
int answer;
NumberFormat percent = NumberFormat.getPercentInstance();
Scanner scan = new Scanner (System.in);
System.out.println ("Quiz Grading");
System.out.println ();
System.out.println("Enter the number of questions on the quiz: ");
numQuestions = scan.nextInt();
//CREATE THE ARRAY FOR THE KEY
int[] key = new int[numQuestions];
System.out.println("Enter the answer key: ");
//LOAD THE ARRAY FOR THE ANSWER KEY WITH INPUT FROM THE USER
for (int i = 0; i < numQuestions; i++)
{
key[i] = scan.nextInt();
}
//OUTER LOOP TO ALLOW THE USER TO ENTER GRADES
// FOR ANY NUMBER OF QUIZZES
do
{
//INNER LOOP TO GET ANSWERS FROM THE USER AND COUNT THE NUMBER OF
// CORRECT ANSWERS
System.out.println("Enter the student answers: ");
numCorrect = 0;
for (int i = 0; i < numQuestions; i++)
{
answer = scan.nextInt();
if (answer == key[i])
{
numCorrect++;
}
}
//DISPLAY THE NUMBER OF CORRECT ANSWERS AND PERCENT
System.out.println("Number of correct answers: " + numCorrect +
" | Percent: " + percent.format((double)numCorrect/numQuestions));
System.out.println();
//ASK USER IF THEY WISH TO GRADE ANOTHER QUIZ
System.out.println("Grade another quiz? (y/n): ");
anotherQuiz = scan.next();
if (anotherQuiz.equalsIgnoreCase("y"))
{
}
else
{
System.out.println("Good-bye");
break;
}
} while (true);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

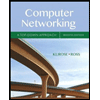
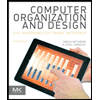
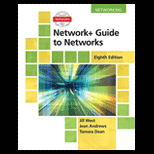
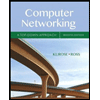
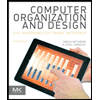
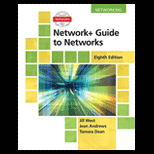
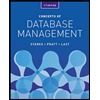
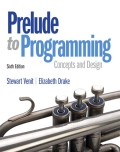
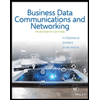