unctionality. (20pts) No Syntax Errors. (20pts*) *Code that cannot be compiled due to syntax errors is nonfunctional code and will receive no points for this entire section. User Inputs the size and values of the array. (5pts) The program must ask the user for the size of the array. This value must be strictly greater than zero, ot
PLEASE WRITE IN JAVA USING ECLIPSE IED
Objective:
Write a
Requirements:
- Functionality. (20pts)
- No Syntax Errors. (20pts*)
- *Code that cannot be compiled due to syntax errors is nonfunctional code and will receive no points for this entire section.
- User Inputs the size and values of the array. (5pts)
- The program must ask the user for the size of the array. This value must be strictly greater than zero, otherwise the program must print “Invalid Array Size” and immediately terminate. Otherwise, the program must create an array of integers of that size.
- The program must ask the user to input each value, and then must store those values into the array.
- All must apply for full credit.
- Find the Minimum Even Value. (5pts)
- The program must then determine the minimum even value found in the array.
- The program must not assume that the first value in the array is either even or odd but must instead find the first even value – if it exists.
- Assume the value 0 is even.
- All must apply for full credit.
- Find the Minimum Odd Value. (5pts)
- The program must then determine the minimum odd value found in the array.
- The program must not assume that the first value in the array is either even or odd but must instead find the first odd value – if it exists.
- All must apply for full credit.
- Print the Results. (5pts)
- The program must clearly print the minimum even or odd value found in the array.
- In the even there were either no even or odd values (or both), then the program must print “No Even Values” or “No Odd Values” respectively. HINT: It may be a good idea to keep track of how many even or odd values are found in the array.
- All must apply for full credit.
- Coding Style. (2pts)
- Readable Code
- Meaningful identifiers for data and methods.
- Proper indentation that clearly identifies statements within the body of a class, a method, a branching statement, a loop statement, etc.
- All the above must apply for full credit.
- Comments. (3pts)
- Your name in the file. (1pts)
- At least 5 meaningful comments in addition to your name. These must describe the function of the code it is near. (2pts)
Solution Tests:
- Does the solution compile?
- Does the solution have your name in the comments and 5 meaningful comments that describe the code?
- If the array has the values {9,1,8,2,7,3,6,4,5} does the program print out:
Min Even: 2
Min Odd: 1
- If the array has the values {1,2,3,4,5,6,-7,-8} does the program print out:
Min Even: -8
Min Odd: -7
- If the array has the values {1,1,1,1,1} does the program print out:
Min Even: No Even Values
Min Odd: 1
- If the array has the values {2,-4,6,8,10} does the program print out:
Min Even: -4
Min Odd: No Odd Values

Java Program:
import java.util.Scanner;
import java.lang.*;
public class Main
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
int n; //Size of the array
System.out.print("Enter size of the array: ");
n = sc.nextInt();
if(n <= 0)
{
System.out.print("Invalid Array Size");
System.exit(0);//Terminate
}
int arr[] = new int[n]; //create an array of integers of that size.
System.out.print("Enter array values:\n");
for(int i=0;i<n;i++)
arr[i] = sc.nextInt();
//Find the Minimum Even Value.
int minEven = 9999; //In case no minimum even value is found.
int minOdd = 9999; //In case no minimum odd value is found.
for(int i=0;i<n;i++)
{
if(arr[i]%2 == 0)
{
if(minEven > arr[i])
minEven = arr[i];
}
else
{
if(minOdd > arr[i])
minOdd = arr[i];
}
}
if(minEven == 9999)
System.out.print("\nNo Even Values");
else
System.out.print("\nMinimum Even Value = " + minEven);
if(minOdd == 9999)
System.out.print("\nNo Odd Values");
else
System.out.print("\nMinimum Odd Value = " + minOdd);
}
}
Output:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

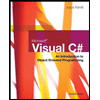
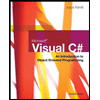