Topical Information Use C++. This lab is designed to give you practice with labeled data in files. Again, classes can help ease your design woes. Program Information Write a program that transfers the contents of one file to a second file. The first file will contain an arbitrary (unknown) number of data groups. A data group will normally consist of a person's name (a string containing spaces), the person's student ID (a large integer), the person's GPA (a floating point number), and the person's gender (a character). The data in each group should be in a labeled data format. Be sure to use some kind of string library to help you with the label/value processing (as we discussed in class). (The library provided here is good but not everything you'll need. You still need functions to convert strings into integers and into floating point numbers. string to bool, string to string, and string to character conversions should be fairly easy.) You can choose the actual values to use for the people's data, but you should be able to handle: invalid labels comments (at least whole-line) unknown labels missing labels unordered (but properly blocked) labels Remember that your program cannot know how many data groups are in the file ahead of time! You'll have to read the files' names from the user. Protect your program against any errors that may occur during the opening of the files. (Try to use a class/functions to break up the program into more manageable pieces.) As an example, you might have the input data file contain: # format is 'label = value' -- one per line # known labels are: name, ID, GPA, and gender # spacing around '=' is okay name = Jason James ID= 123456 GPA =9.2 gender=M # mixed items name = Tammy James GPA = 11.2 gender = f ID = 123457 # mixed, missing, and extra fields name = Henry Ramirez GPA = 12.3 ID = 111888 major = ChE class = soph ID = 788531 # missing fields name=Suzie Shah geNDEr=t The program should produce from this a 'clean copy' such as: # format is 'label = value' -- one per line # known labels are: name, ID, GPA, and gender # spacing around '=' is okay name = Jason James ID = 123456 GPA = 9.2 gender = M name = Tammy James ID = 123457 GPA = 11.2 gender = F name = Henry Ramirez ID = 111888 GPA = 12.3 name = Suzie Shah ID = 788531 gender = T Note how the user's commentary is gone and only the program's reminder commentary is replicated. Also all labels (and gender values) are now in standard capitalization/format and order of data in each group as well as spacing of each data line is uniform. Labels that had their defaulted values (and therefore were not assigned) are not stored. (Although you could output a comment noting that the value was missing: # no GPA specified.) And the program interaction might look something like (the parts underlined are typed by the user): $ ./copypeople.out Welcome to the People Data Copying Program!!! Please enter the name of your data file: bob.dat I'm sorry, I could not open 'bob.dat'. Please enter another name: students File 'students' opened successfully! Please enter the name of the copy file: /can't write here I'm sorry, I could not open '/can't write here'. Please enter another name: students.bak File 'students.bak' opened successfully! Copying data from 'students' to 'students.bak'... Done copying data! Thank you for using the PCP!! Endeavor to have a tremendous day! $
Topical Information
Use C++. This lab is designed to give you practice with labeled data in files. Again, classes can help ease your design woes.
Program Information
Write a program that transfers the contents of one file to a second file. The first file will contain an arbitrary (unknown) number of data groups. A data group will normally consist of a person's name (a string containing spaces), the person's student ID (a large integer), the person's GPA (a floating point number), and the person's gender (a character).
The data in each group should be in a labeled data format. Be sure to use some kind of string library to help you with the label/value processing (as we discussed in class). (The library provided here is good but not everything you'll need. You still need functions to convert strings into integers and into floating point numbers. string to bool, string to string, and string to character conversions should be fairly easy.)
You can choose the actual values to use for the people's data, but you should be able to handle:
- invalid labels
- comments (at least whole-line)
- unknown labels
- missing labels
- unordered (but properly blocked) labels
Remember that your program cannot know how many data groups are in the file ahead of time!
You'll have to read the files' names from the user. Protect your program against any errors that may occur during the opening of the files. (Try to use a class/functions to break up the program into more manageable pieces.)
As an example, you might have the input data file contain:
# format is 'label = value' -- one per line
# known labels are: name, ID, GPA, and gender
# spacing around '=' is okay
name = Jason James
ID= 123456
GPA =9.2
gender=M
# mixed items
name = Tammy James
GPA = 11.2
gender = f
ID = 123457
# mixed, missing, and extra fields
name = Henry Ramirez
GPA = 12.3
ID = 111888
major = ChE
class = soph
ID = 788531
# missing fields
name=Suzie Shah
geNDEr=t
The program should produce from this a 'clean copy' such as:
# format is 'label = value' -- one per line
# known labels are: name, ID, GPA, and gender
# spacing around '=' is okay
name = Jason James
ID = 123456
GPA = 9.2
gender = M
name = Tammy James
ID = 123457
GPA = 11.2
gender = F
name = Henry Ramirez
ID = 111888
GPA = 12.3
name = Suzie Shah
ID = 788531
gender = T
Note how the user's commentary is gone and only the program's reminder commentary is replicated. Also all labels (and gender values) are now in standard capitalization/format and order of data in each group as well as spacing of each data line is uniform. Labels that had their defaulted values (and therefore were not assigned) are not stored. (Although you could output a comment noting that the value was missing: # no GPA specified.)
And the program interaction might look something like (the parts underlined are typed by the user):
$ ./copypeople.out
Welcome to the People Data Copying Program!!!
Please enter the name of your data file: bob.dat
I'm sorry, I could not open 'bob.dat'. Please enter another name: students
File 'students' opened successfully!
Please enter the name of the copy file: /can't write here
I'm sorry, I could not open '/can't write here'. Please enter another name: students.bak
File 'students.bak' opened successfully!
Copying data from 'students' to 'students.bak'...
Done copying data!
Thank you for using the PCP!!
Endeavor to have a tremendous day!
$

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

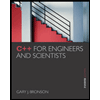
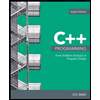
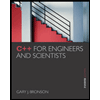
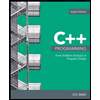
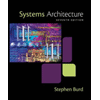