I am trying to create a digital clock however, it has come to my attention that I forgot to put in a main method. Is there any chance you could help me develop a main method for my program so it compiles? This is my code: public class DigitalClock { private int currentHour; private int currentMinutes; public int getHour() { return currentHour; } public void setHour(int currentHour) { this.currentHour = currentHour; } public int getMinutes() { return currentMinutes; } public void setMinutes(int currentMinutes) { this.currentMinutes = currentMinutes; } public static final int HOUR_MAX = 23; // Refactored hourly max public static final int HOUR_MIN = 0; // Refactored hourly min public static final int MINUTES_MAX = 59; // Refactored minute max public static final int MINUTES_MIN = 0; // Refactored minute min public static final int TOTAL_NUMBERS_HOURS = 24; public static final int TOTAL_NUMBERS_MINUTES = 60; /** * Creates a new digital clock with the time set at the given * hours and minutes. * * @precondition 0 <= hour <= 23 AND 0 <= minutes <= 59 * @postcondition getHour()==hour AND getMinutes()==minutes * * @param hour the hour to set for the time * @param minutes the minutes to set for the time */ public DigitalClock (int hour, int minutes) // 2-parameter constructor (parameters: int hour and int minutes) { // enforcing hourly preconditions using appropriate ranges if (hour >= HOUR_MIN && hour <= HOUR_MAX){ currentHour = hour; } else { currentHour = 0; //throw an exception on invalid hour's input throw new IllegalArgumentException("Invalid input for Hours"); } // enforcing minute preconditions using appropriate ranges if (minutes >= MINUTES_MIN && minutes <= MINUTES_MAX) { currentMinutes = minutes; } else { currentMinutes = 0; //throw an exception on invalid minute's input throw new IllegalArgumentException("Invalid input for Minutes"); } } /** * Advances the entire clock by the given number of hours. * If the hours advances past 23 they wrap around. * * @precondition hours >= 0 * @postcondition the clock has moved forward * by the appropriate number of hours * * @param hours the number of hours to add */ public void incrementHoursBy(int hour) { int h = getHour()+hour; { // if statement enforcing precondition if(h>HOUR_MAX) h%=TOTAL_NUMBERS_HOURS; // increasing this.hours by the parameter setHour(h);} // if statement which prevents negative hours from being inputted if (h MINUTES_MAX) { m%=TOTAL_NUMBERS_MINUTES; setMinutes(m);} if (m
I am trying to create a digital clock however, it has come to my attention that I forgot to put in a main method. Is there any chance you could help me develop a main method for my
This is my code:
public class DigitalClock
{
private int currentHour;
private int currentMinutes;
public int getHour()
{
return currentHour;
}
public void setHour(int currentHour)
{
this.currentHour = currentHour;
}
public int getMinutes()
{
return currentMinutes;
}
public void setMinutes(int currentMinutes)
{
this.currentMinutes = currentMinutes;
}
public static final int HOUR_MAX = 23; // Refactored hourly max
public static final int HOUR_MIN = 0; // Refactored hourly min
public static final int MINUTES_MAX = 59; // Refactored minute max
public static final int MINUTES_MIN = 0; // Refactored minute min
public static final int TOTAL_NUMBERS_HOURS = 24;
public static final int TOTAL_NUMBERS_MINUTES = 60;
/**
* Creates a new digital clock with the time set at the given
* hours and minutes.
*
* @precondition 0 <= hour <= 23 AND 0 <= minutes <= 59
* @postcondition getHour()==hour AND getMinutes()==minutes
*
* @param hour the hour to set for the time
* @param minutes the minutes to set for the time
*/
public DigitalClock (int hour, int minutes) // 2-parameter constructor (parameters: int hour and int minutes)
{
// enforcing hourly preconditions using appropriate ranges
if (hour >= HOUR_MIN && hour <= HOUR_MAX){
currentHour = hour;
}
else {
currentHour = 0;
//throw an exception on invalid hour's input
throw new IllegalArgumentException("Invalid input for Hours");
}
// enforcing minute preconditions using appropriate ranges
if (minutes >= MINUTES_MIN && minutes <= MINUTES_MAX) {
currentMinutes = minutes;
}
else {
currentMinutes = 0;
//throw an exception on invalid minute's input
throw new IllegalArgumentException("Invalid input for Minutes");
}
}
/**
* Advances the entire clock by the given number of hours.
* If the hours advances past 23 they wrap around.
*
* @precondition hours >= 0
* @postcondition the clock has moved forward
* by the appropriate number of hours
*
* @param hours the number of hours to add
*/
public void incrementHoursBy(int hour)
{
int h = getHour()+hour; {
// if statement enforcing precondition
if(h>HOUR_MAX)
h%=TOTAL_NUMBERS_HOURS;
// increasing this.hours by the parameter
setHour(h);}
// if statement which prevents negative hours from being inputted
if (h<HOUR_MIN) {
throw new IllegalArgumentException("Invalid input. No negative hours");
}
}
public void incrementMinutesBy(int minutes) {
int m = getMinutes()+minutes;
if (m > MINUTES_MAX) {
m%=TOTAL_NUMBERS_MINUTES;
setMinutes(m);}
if (m<MINUTES_MIN) {
throw new IllegalArgumentException("Invalid input. No negative minutes");
}
}
}

Step by step
Solved in 3 steps

This solution is unclear and does not answer my question. I am trying to add a main method to my code.
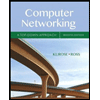
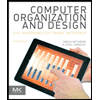
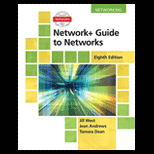
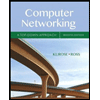
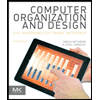
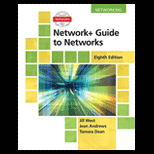
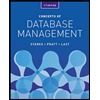
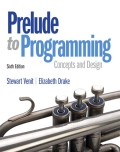
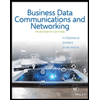