this is my retail item code: //Import statements public class RetailItem { private String description; private int units; private double price; public RetailItem() { } public RetailItem(String x, int y, double z) { description = x; units = y; price = z; } public RetailItem(RetailItem i) { } public void setDescription(String x) { description = x; } public void setPrice(double z) { price = z; } void setUnits(int y) { units = y; } public int getUnits() { return units; } public String getDescription() { return description; } public double getPrice() { return price; } // Main class public static void main(String[] args) { String str = "Shirt"; RetailItem r1 = new RetailItem("Jacket", 12, 59.95); RetailItem r2 = new RetailItem("Designer Jeans", 40, 34.95); RetailItem r3 = new RetailItem(); // call the set methods r3.setDescription(str); r3.setUnits(20); r3.setPrice(24.95); // print all statements System.out.println("Item #1\t" + r1.getDescription() + "\t\t" + r1.getUnits() + " \t" + r1.getPrice()); System.out.println("Item #2\t" + r2.getDescription() + "\t" + r2.getUnits() + " \t" + r2.getPrice()); System.out.println("Item #3\t" + r3.getDescription() + "\t\t" + r3.getUnits() + " \t " + r3.getPrice()); System.exit(0); }
this is my retail item code: //Import statements public class RetailItem { private String description; private int units; private double price; public RetailItem() { } public RetailItem(String x, int y, double z) { description = x; units = y; price = z; } public RetailItem(RetailItem i) { } public void setDescription(String x) { description = x; } public void setPrice(double z) { price = z; } void setUnits(int y) { units = y; } public int getUnits() { return units; } public String getDescription() { return description; } public double getPrice() { return price; } // Main class public static void main(String[] args) { String str = "Shirt"; RetailItem r1 = new RetailItem("Jacket", 12, 59.95); RetailItem r2 = new RetailItem("Designer Jeans", 40, 34.95); RetailItem r3 = new RetailItem(); // call the set methods r3.setDescription(str); r3.setUnits(20); r3.setPrice(24.95); // print all statements System.out.println("Item #1\t" + r1.getDescription() + "\t\t" + r1.getUnits() + " \t" + r1.getPrice()); System.out.println("Item #2\t" + r2.getDescription() + "\t" + r2.getUnits() + " \t" + r2.getPrice()); System.out.println("Item #3\t" + r3.getDescription() + "\t\t" + r3.getUnits() + " \t " + r3.getPrice()); System.exit(0); }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
this is my retail item code:
//Import statements
public class RetailItem {
private String description;
private int units;
private double price;
public RetailItem()
{
}
public RetailItem(String x, int y, double z)
{
description = x;
units = y;
price = z;
}
public RetailItem(RetailItem i) {
}
public void setDescription(String x)
{
description = x;
}
public void setPrice(double z) {
price = z;
}
void setUnits(int y) {
units = y;
}
public int getUnits()
{
return units;
}
public String getDescription()
{
return description;
}
public double getPrice()
{
return price;
}
// Main class
public static void main(String[] args)
{
String str = "Shirt";
RetailItem r1 = new RetailItem("Jacket", 12, 59.95);
RetailItem r2 = new RetailItem("Designer Jeans", 40, 34.95);
RetailItem r3 = new RetailItem();
// call the set methods
r3.setDescription(str);
r3.setUnits(20);
r3.setPrice(24.95);
// print all statements
System.out.println("Item #1\t" + r1.getDescription() + "\t\t" + r1.getUnits() + " \t" + r1.getPrice());
System.out.println("Item #2\t" + r2.getDescription() + "\t" + r2.getUnits() + " \t" + r2.getPrice());
System.out.println("Item #3\t" + r3.getDescription() + "\t\t" + r3.getUnits() + " \t " + r3.getPrice());
System.exit(0);
}
}

Transcribed Image Text:Part II CashRegister Class
Write a CashRegister class that can be used with the Retailltem class that you wrote. The Cash Register class should simulate the sale of a retail item.
It should have a constructor that accepts a Retailltem object as an argument. The constructor should also accept an integer that represents the
quantity of items being purchased. In addition, the class should have the following methods:
The getSubtotal method should return the subtotal of the sale, which is the quantity multiplied by the price. This method must get the price from the
Retailltem object that was passed as an argument to the constructor.
The getTax method should return the amount of sales tax on the purchase. The sales tax rate is 6 percent of a retail sale.
The getTotal method should return the total of the sale, which is the subtotal plus the sales tax.
Demonstrate the class in a program that performs the following tasks:
displays the list of items available in the store
• prompts the user to enter an item number and reads the value
• asks the user for the quantity of items being purchased
• displays the sale's subtotal, amount of sales tax, and total.
Save the purchases in an array of type Cash Register.
Simulate at least 3 purchases for a day, and save all values in the array defined earlier. Calculate the total number of items purchased for the day, and
the total price paid for them.
Upload all the JAVA files in a zip file and create a word document with all the screenshots of your running program and upload all these files.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
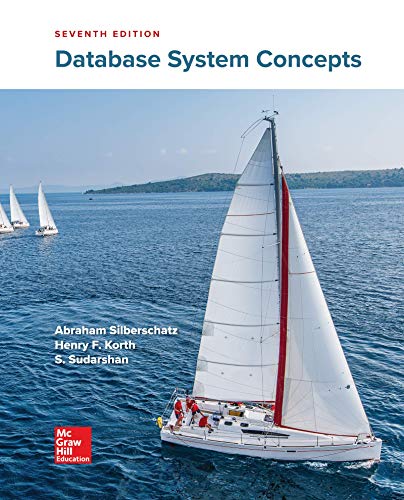
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
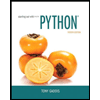
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
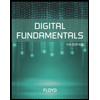
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
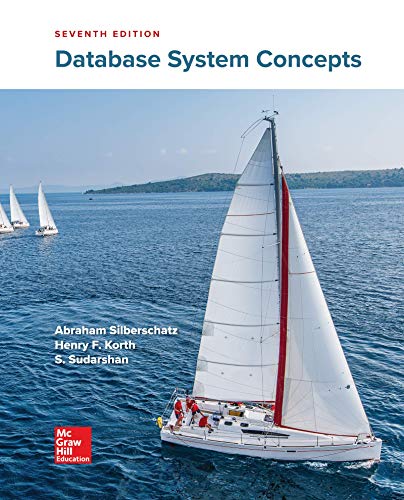
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
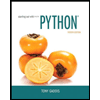
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
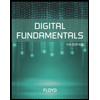
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
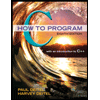
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
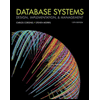
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
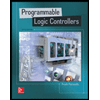
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education