This code is a part of a C dictionary. Please write the code for the below requirements. dict_get Next, you will implement: char* dict_get (const dict_t* dict, const char* key); This function goes through the list given by dict. If you use the above structure, this means starting at el = dict->head and checking each time whether the key at el is key; if it is not, we set el = el->next, until either key is found, or we reach el == NULL. To compare key with el->key, we need to compare one by one each character in key with those in el->key. Remember that strings in C are just pointers (memory addresses) to the first character in the string, so comparing el->key == key will not do what you want. So how do you even get the length of a string s? You would start at memory location s and advance as long as the byte at s (i.e. *s) is not the end-of-string marker (\0, the NULL character). This can get a bit messy, so luckily, you are allowed to use the string comparison function strcmp(3) provided by the standard library: strcmp (s1, s2): returns 0 iff s1 and s2 are the same strings. Hence in if (strcmp (s1, s2))..., the condition holds if the strings are different—be careful. If key is found, return the corresponding value; otherwise, return NULL. —Note remark that the type of the argument dict is not simply dict_t* but const dict_t*. This is used to indicate to the compiler that the function dict_get guarantees that it won’t modify the contents of dict (that is, *dict). These indications are crucial to providing clear contracts to the user, helping the compiler make correct assumptions, and avoiding modifying objects you did not intend to modify.
This code is a part of a C dictionary. Please write the code for the below requirements.
dict_get
Next, you will implement:
This function goes through the list given by dict. If you use the above structure, this means starting at el = dict->head and checking each time whether the key at el is key; if it is not, we set el = el->next, until either key is found, or we reach el == NULL.
To compare key with el->key, we need to compare one by one each character in key with those in el->key. Remember that strings in C are just pointers (memory addresses) to the first character in the string, so comparing el->key == key will not do what you want. So how do you even get the length of a string s? You would start at memory location s and advance as long as the byte at s (i.e. *s) is not the end-of-string marker (\0, the NULL character). This can get a bit messy, so luckily, you are allowed to use the string comparison function strcmp(3) provided by the standard library:
- strcmp (s1, s2): returns 0 iff s1 and s2 are the same strings. Hence in if (strcmp (s1, s2))..., the condition holds if the strings are different—be careful.
If key is found, return the corresponding value; otherwise, return NULL.
—Note
remark that the type of the argument dict is not simply dict_t* but const dict_t*. This is used to indicate to the compiler that the function dict_get guarantees that it won’t modify the contents of dict (that is, *dict). These indications are crucial to providing clear contracts to the user, helping the compiler make correct assumptions, and avoiding modifying objects you did not intend to modify.
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

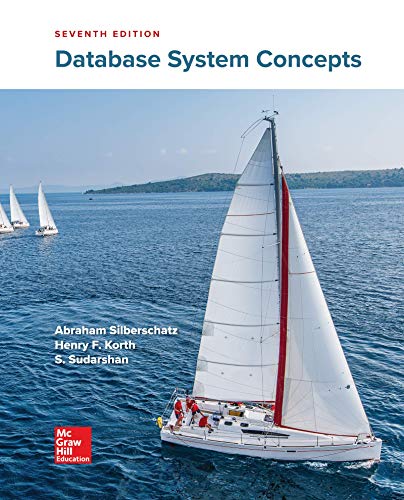
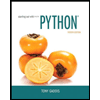
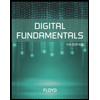
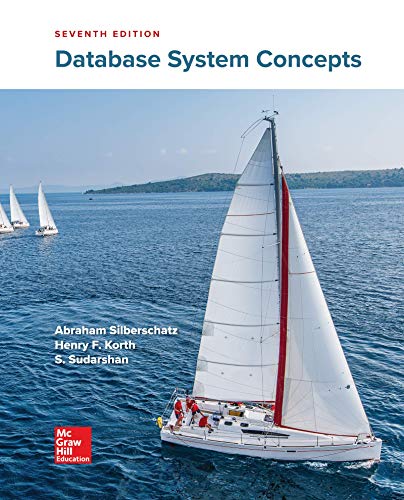
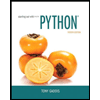
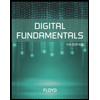
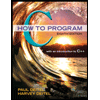
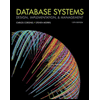
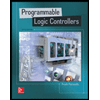