The push member function simulates the push of a numeric button with value 0 to 9. The open member function simulates the push of the # key that causes the lock to open, provided that the right combination was entered. (For now, the combination is hardwired as 1729. You will see in Section 9.6 how to change it.) As the data representation, use an integer containing the digits that have been entered up to this point. You will need to figure out how to update that number. For example, if the user had previously pushed 1 and 7, causing input to be 17, and now pushes 9, how can you set input to 179? In the open member function, return true if the lock would have been opened, false otherwise. Clicking that button resets the entered keys, whether or not the correct combination has been entered. Complete the following file: c++ please #include using namespace std; /** A simulated lock with digit buttons. */ class Lock { public: /** Simulates a digit button push. @param button a digit 0 ... 9 */ void push(int button); /** Simulates a push of the open button. @return true if the lock opened */ bool open(); private: int combination = 1729; int input = 0; }; void Lock::push(int button) { . . . } bool Lock::open() { . . . } int main() { Lock my_lock; my_lock.push(1); my_lock.push(7); my_lock.push(3); my_lock.push(9); cout << boolalpha; cout << my_lock.open() << endl; cout << "Expected: false" << endl; my_lock.push(1); my_lock.push(7); my_lock.push(2); my_lock.push(9); cout << my_lock.open() << endl; cout << "Expected: true" << endl; my_lock.push(1); my_lock.push(7); my_lock.push(2); cout << my_lock.open() << endl; cout << "Expected: false" << endl; my_lock.push(9); cout << my_lock.open() << endl; cout << "Expected: false" << endl; my_lock.push(1); my_lock.push(7); my_lock.push(2); my_lock.push(9); cout << my_lock.open() << endl; cout << "Expected: true" << endl; return 0; }
The push member function simulates the push of a numeric button with value 0 to 9.
The open member function simulates the push of the # key that causes the lock to open, provided that the right combination was entered. (For now, the combination is hardwired as 1729. You will see in Section 9.6 how to change it.)
As the data representation, use an integer containing the digits that have been entered up to this point. You will need to figure out how to update that number. For example, if the user had previously pushed 1 and 7, causing input to be 17, and now pushes 9, how can you set input to 179?
In the open member function, return true if the lock would have been opened, false otherwise. Clicking that button resets the entered keys, whether or not the correct combination has been entered.
Complete the following file:
c++ please
#include <iostream>
using namespace std;
/**
A simulated lock with digit buttons.
*/
class Lock
{
public:
/**
Simulates a digit button push.
@param button a digit 0 ... 9
*/
void push(int button);
/**
Simulates a push of the open button.
@return true if the lock opened
*/
bool open();
private:
int combination = 1729;
int input = 0;
};
void Lock::push(int button)
{
. . .
}
bool Lock::open()
{
. . .
}
int main()
{
Lock my_lock;
my_lock.push(1);
my_lock.push(7);
my_lock.push(3);
my_lock.push(9);
cout << boolalpha;
cout << my_lock.open() << endl;
cout << "Expected: false" << endl;
my_lock.push(1);
my_lock.push(7);
my_lock.push(2);
my_lock.push(9);
cout << my_lock.open() << endl;
cout << "Expected: true" << endl;
my_lock.push(1);
my_lock.push(7);
my_lock.push(2);
cout << my_lock.open() << endl;
cout << "Expected: false" << endl;
my_lock.push(9);
cout << my_lock.open() << endl;
cout << "Expected: false" << endl;
my_lock.push(1);
my_lock.push(7);
my_lock.push(2);
my_lock.push(9);
cout << my_lock.open() << endl;
cout << "Expected: true" << endl;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

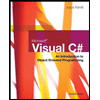
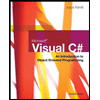