(The Rectangle class) Following the example of the Circle class in Section 8.2, design a class named Rectangle to represent a rectangle. The class contains: 1 Two double data fields named width and height that specify the width and height of the rectangle. The default values are 1 for both width and height. 1A no-arg constructor that creates a default rectangle. 1A constructor that creates a rectangle with the specified width and height. 1A method named getArea() that returns the area of this rectangle. 1A method named getPerimeter() that returns the perimeter. Draw the UML diagram for the class and then implement the class. Write a test program that creates two Rectangle objects-one with width 4 and height 40 and the other with width 3.5 and height 35.9. Display the width, height, area, and perimeter of each rectangle in this order. SAMPLE RUN #0: java Rectangle Standard Output Hide Invisibles The area of a:4.0.x.40.0.Rectangle is.160.04 The perimeter.of.a:4.0.x.40.0. Rectangle·is 88.04 The area of a:3.5.x:35.9.Rectangle is125.64999999999999e The perimeter.of·a•3.5.x.35.9• Rectangle·is·78.84
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
I tried to get this answered before but I couldn't get the code to work fully from what he gave me.
"
//Definition of class rectangle
public class Rectangle
{
//Declare required variables
private double w;
private double h;
//No-argument constructor
public Rectangle()
{
//Assign "w" to "1"
w = 1;
//Assign "h" to "1"
h = 1;
}
//Parameterized constructor
public Rectangle(double wid, double ht)
{
//Assign "wid" to "w"
this.w = wid;
//Assign "ht" to "w"
this.h = ht;
}
//Method definition for width
public double getWidth()
{
//Return width
return w;
}
//Method definition for height
public double getHeight()
{
//Return height
return h;
}
//Method definition for perimeter
public double getPerimeter()
{
//Return the perimeter
return 2.0*(w + h);
}
//Method definition for area
public double getArea()
{
//Return the area
return w*h;
}
}
//Definition of test class
public class Test
{
//Definition of main class
public static void main(String[] args)
{
//Create an object for rectangle class
Rectangle rect1 = new Rectangle(4, 40);
//Printing the area of rectangle
System.out.printf("The area of a 4.0 x 40.0 Rectangle is %.1f ", rect1.getArea());
//Printing the perimeter of rectangle
System.out.printf("The perimeter of a 4.0 x 40.0 Rectangle is %.1f ", rect1.getPerimeter());
//Create an object for rectangle class
Rectangle rect2 = new Rectangle(3.5, 35.9);
//Printing the area of rectangle
System.out.printf("The area of a 3.5 x 35.9.0 Rectangle is %.1f ", rect2.getArea());
//Printing the perimeter of rectangle
System.out.printf("The perimeter of a 3.5 x 35.9.0 Rectangle is %.1f ", rect2.getPerimeter());
}
}
"
When I put it in I get this error ( Rectangle.java:96: error: class Test is public, should be declared in a file named Test.java
public class Test )
I tried removing the public from the class it, and I don't get an error, but nothing gets output.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

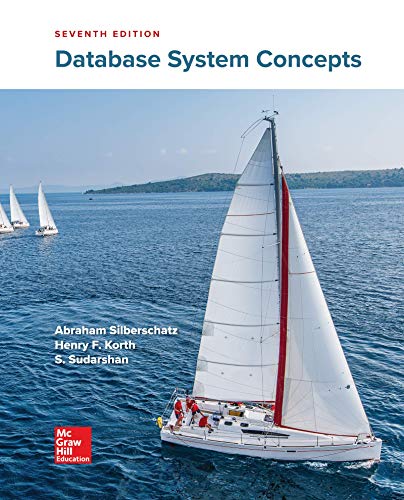
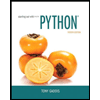
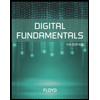
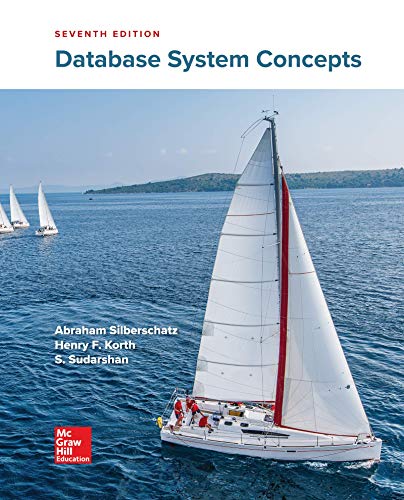
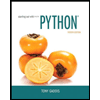
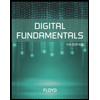
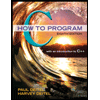
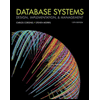
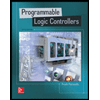