The program opens up the data.txt file using the fopen function and will read the data into two arrays called column1 and column2. Since we don't know how big the file is, we can't just declare arrays of a fixed size. Instead, we will allocate memory using the malloc function. Initially, we allocate space for 10 rows of integers. The next step is where you write code to read in the file. This will be a loop in which you read each line of the file one by one. To read a line of the file, use the fscanf function to read in the two columns as follows int num1, num2; int retval = fscanf(fp,"%d %d\n", &numl, &num2) You will need to put the num1 and num2 variables into the appropriate array location of column1 and column2. Every time you read a line, increment the num_rows variable. fscanf will return an EOF when it has reached the end of the file. So, when retval==EOF, you can exit the loop. Since the file may have more than 10 rows, you may need to change memory allocation. So, check if the number of rows is a multiple of 10 (which you can do by checking if num_rows 10 == 0), and if so, reallocate the memory to 10 more than num_rows. column1 = realloc (column1, (num_rows + 10)*sizeof (int)); At the end of the program, iterate through the rows and write the columns to the data2 file with the columns switched.
The program opens up the data.txt file using the fopen function and will read the data into two arrays called column1 and column2. Since we don't know how big the file is, we can't just declare arrays of a fixed size. Instead, we will allocate memory using the malloc function. Initially, we allocate space for 10 rows of integers. The next step is where you write code to read in the file. This will be a loop in which you read each line of the file one by one. To read a line of the file, use the fscanf function to read in the two columns as follows int num1, num2; int retval = fscanf(fp,"%d %d\n", &numl, &num2) You will need to put the num1 and num2 variables into the appropriate array location of column1 and column2. Every time you read a line, increment the num_rows variable. fscanf will return an EOF when it has reached the end of the file. So, when retval==EOF, you can exit the loop. Since the file may have more than 10 rows, you may need to change memory allocation. So, check if the number of rows is a multiple of 10 (which you can do by checking if num_rows 10 == 0), and if so, reallocate the memory to 10 more than num_rows. column1 = realloc (column1, (num_rows + 10)*sizeof (int)); At the end of the program, iterate through the rows and write the columns to the data2 file with the columns switched.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section8.4: File Streams As Function Arguments
Problem 3E
Related questions
Question
Data.txt is given in the image

Transcribed Image Text:The program opens up the data.txt file using the fopen function and will read the data into two
arrays called column1 and column2. Since we don't know how big the file is, we can't just
declare arrays of a fixed size. Instead, we will allocate memory using the malloc function. Initially,
we allocate space for 10 rows of integers.
The next step is where you write code to read in the file. This will be a loop in which you read each
line of the file one by one. To read a line of the file, use the fscanf function to read in the two
columns as follows
int numl, num2;
int retval= fscanf(fp,"%d %d\n", &numl, &num2)
You will need to put the num1 and num2 variables into the appropriate array location of column1
and column2. Every time you read a line, increment the num_rows variable. fscanf will
return an EOF when it has reached the end of the file. So, when retval==EOF, you can exit the
loop.
Since the file may have more than 10 rows, you may need to change memory allocation. So, check if
the number of rows is a multiple of 10 (which you can do by checking if num_rows % 10 == 0),
and if so, reallocate the memory to 10 more than num_rows.
column1 = realloc (column1, (num_rows + 10) *sizeof (int));
At the end of the program, iterate through the rows and write the columns to the data2 file with
the columns switched.
![1. The following program is the start of a program that will read a file consisting of two columns of
data. It is unknown how many rows of data there are.
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char **argv)
{
if (arge ! 3 ) {
printf("%s input file outfile\n", argv[0]);
exit(0);
}
FILE *fp = fopen (argv[1], "r");
if (fp == NULL) {
perror ("Could not open file");
exit(0);
}
FILE *fp2 = fopen (argv [2], "w");
if (fp2 == NULL) {
perror ("Could not open file");
exit(0);
12 72
DATA.TXT
}
int num_rows = 0;
int *column1 = malloc (10*sizeof(int));
int *column2 = malloc (10*sizeof(int));
// TODO read from the data.txt file with a loop using fscanf
78 29
for (int i=0; i<num_rows; i++) {
// TODO write to the data2.txt file using fprintf
The program will be executed as follows:
$ ./fileio data.txt data2.txt
where data.txt is the name of the file containing the data. data.txt has been provided for
you on
data2.txt is the name of the file that you will be writing to. The data.txt
file has two columns of numbers and you don't know how many rows of data there are. Your
program will need to read in the two columns into two arrays. After you read in the two arrays,
you will need to write to the data2.txt file with the columns flipped.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe8b51a5c-8c6a-40f8-bbb0-bd49d361cfb6%2F794beebd-9e3f-4f8b-bd37-b359cbf7c101%2Fngxv1xe_processed.png&w=3840&q=75)
Transcribed Image Text:1. The following program is the start of a program that will read a file consisting of two columns of
data. It is unknown how many rows of data there are.
#include <stdio.h>
#include <stdlib.h>
int main(int argc, char **argv)
{
if (arge ! 3 ) {
printf("%s input file outfile\n", argv[0]);
exit(0);
}
FILE *fp = fopen (argv[1], "r");
if (fp == NULL) {
perror ("Could not open file");
exit(0);
}
FILE *fp2 = fopen (argv [2], "w");
if (fp2 == NULL) {
perror ("Could not open file");
exit(0);
12 72
DATA.TXT
}
int num_rows = 0;
int *column1 = malloc (10*sizeof(int));
int *column2 = malloc (10*sizeof(int));
// TODO read from the data.txt file with a loop using fscanf
78 29
for (int i=0; i<num_rows; i++) {
// TODO write to the data2.txt file using fprintf
The program will be executed as follows:
$ ./fileio data.txt data2.txt
where data.txt is the name of the file containing the data. data.txt has been provided for
you on
data2.txt is the name of the file that you will be writing to. The data.txt
file has two columns of numbers and you don't know how many rows of data there are. Your
program will need to read in the two columns into two arrays. After you read in the two arrays,
you will need to write to the data2.txt file with the columns flipped.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
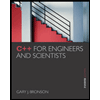
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
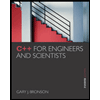
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage