the following piece of code. (The numbers on the left margin denote line numbers.) line# 01 class LL_node { 02 int val; 03 LL_node next; 04 public LL_node (int n) { 05 val = n; 06 next = null; 07 } 08 public void set_next (LL_node nextNode) { 09 next = nextNode; 10 } 11 public LL_node get_next () {
Consider the following piece of code. (The numbers on the left margin denote line numbers.)
line#
01 class LL_node {
02 int val;
03 LL_node next;
04 public LL_node (int n) {
05 val = n;
06 next = null;
07 }
08 public void set_next (LL_node nextNode) {
09 next = nextNode;
10 }
11 public LL_node get_next () {
12 return next;
13 }
14 public void set_value (int input) {
15 val = input;
16 }
17 public int get_value () {
18 return val;
19 }
20 }
21 public class LL {
22 protected LL_node head;
23 protected LL_node tail;
24 public LL () {
25 head = null;
26 tail = null;
27 }
28 public int append (int n) {
29 if (head == null) {
30 head = new LL_node(n);
31 tail = head;
32 } else {
33 LL_node new_node;
34 new_node = new LL_node(n);
35 tail.set_next (new_node);
36 tail = new_node;
37 }
38 return n;
39 }
40 }
Out of the following, tick the true statements and leave the false statements unticked.
(More than one of the statements could be true. You get full marks only for marking all the statements correctly. There are no partial marks.)
To search an element in a linked list represented by an object/instance of class LL, we have to start from the head node and traverse the list until we find the element or reach the tail node without success.
When the linked list represented by an object/instance of class LL is empty, the head and tail references point to null.
An object/instance of class LL can represent a linked list that allows nodes to be added at the tail end only.

Step by step
Solved in 2 steps

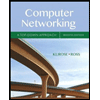
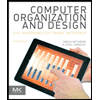
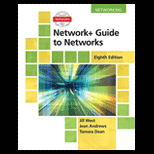
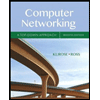
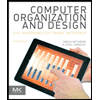
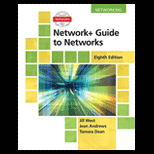
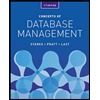
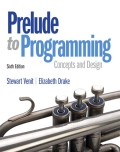
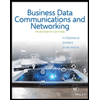